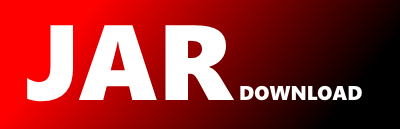
com.auth0.jwt.impl.PayloadImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-jwt-nodependencies Show documentation
Show all versions of java-jwt-nodependencies Show documentation
This is a drop in replacement for the auth0 java-jwt library (see https://github.com/auth0/java-jwt). This jar makes sure there are no external dependencies (e.g. fasterXml, Apacha Commons) needed. This is useful when deploying to an application server (e.g. tomcat with Alfreso or Pega).
The newest version!
package com.auth0.jwt.impl;
import com.auth0.jwt.interfaces.Claim;
import com.auth0.jwt.interfaces.Payload;
import com.fasterxml.jackson.databind.JsonNode;
import java.util.*;
import static com.auth0.jwt.impl.JsonNodeClaim.extractClaim;
/**
* The PayloadImpl class implements the Payload interface.
*/
class PayloadImpl implements Payload {
private final String issuer;
private final String subject;
private final List audience;
private final Date expiresAt;
private final Date notBefore;
private final Date issuedAt;
private final String jwtId;
private final Map tree;
PayloadImpl(String issuer, String subject, List audience, Date expiresAt, Date notBefore, Date issuedAt, String jwtId, Map tree) {
this.issuer = issuer;
this.subject = subject;
this.audience = audience;
this.expiresAt = expiresAt;
this.notBefore = notBefore;
this.issuedAt = issuedAt;
this.jwtId = jwtId;
this.tree = Collections.unmodifiableMap(tree == null ? new HashMap() : tree);
}
Map getTree() {
return tree;
}
@Override
public String getIssuer() {
return issuer;
}
@Override
public String getSubject() {
return subject;
}
@Override
public List getAudience() {
return audience;
}
@Override
public Date getExpiresAt() {
return expiresAt;
}
@Override
public Date getNotBefore() {
return notBefore;
}
@Override
public Date getIssuedAt() {
return issuedAt;
}
@Override
public String getId() {
return jwtId;
}
@Override
public Claim getClaim(String name) {
return extractClaim(name, tree);
}
@Override
public Map getClaims() {
Map claims = new HashMap<>();
for (String name : tree.keySet()) {
claims.put(name, extractClaim(name, tree));
}
return Collections.unmodifiableMap(claims);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy