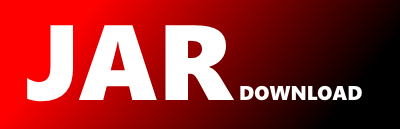
nl.open.jwtdependency.org.bouncycastle.asn1.LazyEncodedSequence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-jwt-nodependencies Show documentation
Show all versions of java-jwt-nodependencies Show documentation
This is a drop in replacement for the auth0 java-jwt library (see https://github.com/auth0/java-jwt). This jar makes sure there are no external dependencies (e.g. fasterXml, Apacha Commons) needed. This is useful when deploying to an application server (e.g. tomcat with Alfreso or Pega).
The newest version!
package org.bouncycastle.asn1;
import java.io.IOException;
import java.util.Enumeration;
/**
* Note: this class is for processing DER/DL encoded sequences only.
*/
class LazyEncodedSequence
extends ASN1Sequence
{
private byte[] encoded;
LazyEncodedSequence(
byte[] encoded)
throws IOException
{
this.encoded = encoded;
}
private void parse()
{
Enumeration en = new LazyConstructionEnumeration(encoded);
while (en.hasMoreElements())
{
seq.addElement(en.nextElement());
}
encoded = null;
}
public synchronized ASN1Encodable getObjectAt(int index)
{
if (encoded != null)
{
parse();
}
return super.getObjectAt(index);
}
public synchronized Enumeration getObjects()
{
if (encoded == null)
{
return super.getObjects();
}
return new LazyConstructionEnumeration(encoded);
}
public synchronized int size()
{
if (encoded != null)
{
parse();
}
return super.size();
}
ASN1Primitive toDERObject()
{
if (encoded != null)
{
parse();
}
return super.toDERObject();
}
ASN1Primitive toDLObject()
{
if (encoded != null)
{
parse();
}
return super.toDLObject();
}
int encodedLength()
throws IOException
{
if (encoded != null)
{
return 1 + StreamUtil.calculateBodyLength(encoded.length) + encoded.length;
}
else
{
return super.toDLObject().encodedLength();
}
}
void encode(
ASN1OutputStream out)
throws IOException
{
if (encoded != null)
{
out.writeEncoded(BERTags.SEQUENCE | BERTags.CONSTRUCTED, encoded);
}
else
{
super.toDLObject().encode(out);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy