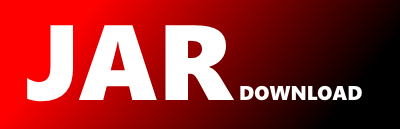
nl.open.jwtdependency.org.bouncycastle.crypto.params.ECDomainParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-jwt-nodependencies Show documentation
Show all versions of java-jwt-nodependencies Show documentation
This is a drop in replacement for the auth0 java-jwt library (see https://github.com/auth0/java-jwt). This jar makes sure there are no external dependencies (e.g. fasterXml, Apacha Commons) needed. This is useful when deploying to an application server (e.g. tomcat with Alfreso or Pega).
The newest version!
package org.bouncycastle.crypto.params;
import java.math.BigInteger;
import org.bouncycastle.math.ec.ECConstants;
import org.bouncycastle.math.ec.ECCurve;
import org.bouncycastle.math.ec.ECPoint;
import org.bouncycastle.util.Arrays;
public class ECDomainParameters
implements ECConstants
{
private ECCurve curve;
private byte[] seed;
private ECPoint G;
private BigInteger n;
private BigInteger h;
public ECDomainParameters(
ECCurve curve,
ECPoint G,
BigInteger n)
{
this(curve, G, n, ONE, null);
}
public ECDomainParameters(
ECCurve curve,
ECPoint G,
BigInteger n,
BigInteger h)
{
this(curve, G, n, h, null);
}
public ECDomainParameters(
ECCurve curve,
ECPoint G,
BigInteger n,
BigInteger h,
byte[] seed)
{
this.curve = curve;
this.G = G.normalize();
this.n = n;
this.h = h;
this.seed = seed;
}
public ECCurve getCurve()
{
return curve;
}
public ECPoint getG()
{
return G;
}
public BigInteger getN()
{
return n;
}
public BigInteger getH()
{
return h;
}
public byte[] getSeed()
{
return Arrays.clone(seed);
}
public boolean equals(
Object obj)
{
if (this == obj)
{
return true;
}
if ((obj instanceof ECDomainParameters))
{
ECDomainParameters other = (ECDomainParameters)obj;
return this.curve.equals(other.curve) && this.G.equals(other.G) && this.n.equals(other.n) && this.h.equals(other.h);
}
return false;
}
public int hashCode()
{
int hc = curve.hashCode();
hc *= 37;
hc ^= G.hashCode();
hc *= 37;
hc ^= n.hashCode();
hc *= 37;
hc ^= h.hashCode();
return hc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy