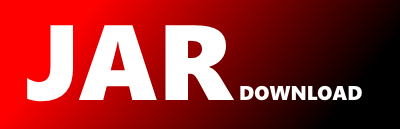
nl.open.jwtdependency.org.bouncycastle.jce.ECNamedCurveTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-jwt-nodependencies Show documentation
Show all versions of java-jwt-nodependencies Show documentation
This is a drop in replacement for the auth0 java-jwt library (see https://github.com/auth0/java-jwt). This jar makes sure there are no external dependencies (e.g. fasterXml, Apacha Commons) needed. This is useful when deploying to an application server (e.g. tomcat with Alfreso or Pega).
The newest version!
package org.bouncycastle.jce;
import java.util.Enumeration;
import org.bouncycastle.asn1.ASN1ObjectIdentifier;
import org.bouncycastle.asn1.x9.X9ECParameters;
import org.bouncycastle.jce.spec.ECNamedCurveParameterSpec;
/**
* a table of locally supported named curves.
*/
public class ECNamedCurveTable
{
/**
* return a parameter spec representing the passed in named
* curve. The routine returns null if the curve is not present.
*
* @param name the name of the curve requested
* @return a parameter spec for the curve, null if it is not available.
*/
public static ECNamedCurveParameterSpec getParameterSpec(
String name)
{
X9ECParameters ecP = org.bouncycastle.crypto.ec.CustomNamedCurves.getByName(name);
if (ecP == null)
{
try
{
ecP = org.bouncycastle.crypto.ec.CustomNamedCurves.getByOID(new ASN1ObjectIdentifier(name));
}
catch (IllegalArgumentException e)
{
// ignore - not an oid
}
if (ecP == null)
{
ecP = org.bouncycastle.asn1.x9.ECNamedCurveTable.getByName(name);
if (ecP == null)
{
try
{
ecP = org.bouncycastle.asn1.x9.ECNamedCurveTable.getByOID(new ASN1ObjectIdentifier(name));
}
catch (IllegalArgumentException e)
{
// ignore - not an oid
}
}
}
}
if (ecP == null)
{
return null;
}
return new ECNamedCurveParameterSpec(
name,
ecP.getCurve(),
ecP.getG(),
ecP.getN(),
ecP.getH(),
ecP.getSeed());
}
/**
* return an enumeration of the names of the available curves.
*
* @return an enumeration of the names of the available curves.
*/
public static Enumeration getNames()
{
return org.bouncycastle.asn1.x9.ECNamedCurveTable.getNames();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy