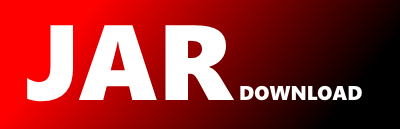
nl.pdok.ServiceCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geoserver-workspace-builder Show documentation
Show all versions of geoserver-workspace-builder Show documentation
PDOK Geoserver Workspace Builder
The newest version!
package nl.pdok;
import java.util.ArrayList;
import java.util.List;
import nl.pdok.catalogus.Catalogus;
import nl.pdok.catalogus.model.CatalogusEntry;
import nl.pdok.catalogus.model.Dataset;
import nl.pdok.catalogus.model.GroupLayerLayer;
import nl.pdok.catalogus.model.RelativeHeight;
import nl.pdok.workspacebuilder.GeoserverRestProxy;
import nl.pdok.workspacebuilder.PdokBuilder;
import nl.pdok.workspacebuilder.model.Service;
import nl.pdok.workspacebuilder.model.ServiceInfo;
import nl.pdok.workspacebuilder.model.Style;
import nl.pdok.workspacebuilder.model.datastore.DbDatastore;
import nl.pdok.workspacebuilder.model.datastore.MosaicDatastore;
import nl.pdok.workspacebuilder.model.datastore.RasterDatastore;
import nl.pdok.workspacebuilder.model.layer.Coverage;
import nl.pdok.workspacebuilder.model.layer.DbLayer;
import nl.pdok.workspacebuilder.model.layer.GroupLayer;
import nl.pdok.workspacebuilder.model.layer.Layer;
import nl.pdok.workspacebuilder.model.layer.SingleLayer;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Created with IntelliJ IDEA.
* User: nijhur
* Date: 29-4-14
* Time: 7:47
* To change this template use File | Settings | File Templates.
*/
public class ServiceCreator {
final static Logger logger = LoggerFactory.getLogger(ServiceCreator.class);
private PdokBuilder pdokBuilder;
private nl.pdok.catalogus.Catalogus catalogus;
private static String WMS = "WMS";
private static String WFS = "WFS";
private static String WCS = "WCS";
private static String AUTHORITY_HREF = "http://www.pdok.nl";
private static String AUTHORITY_NAME = "pdok";
public ServiceCreator(String catalogusRoot){
catalogus = new Catalogus(catalogusRoot);
pdokBuilder = new PdokBuilder(catalogus);
}
public boolean update(String serviceName, GeoserverRestProxy geoserverRestProxy, boolean removeAll){
logger.info("== Start configuring service: " + serviceName);
CatalogusEntry catalogusEntry = catalogus.getCatalogus(serviceName);
boolean result = false;
if ( catalogusEntry == null ) {
logger.error("No valid Catalog found for: " + serviceName);
result = false;
} else {
Service service = new Service(serviceName, catalogus);
getDatastores(catalogusEntry, service);
getServiceInfo(catalogusEntry, service);
getLayersAndCoverages(catalogusEntry, service);
getGroupLayers(catalogusEntry, service);
buildGeoserverWorkspace(service, geoserverRestProxy, removeAll);
result = true;
}
logger.info("== Finish: " + serviceName);
return result;
}
private void buildGeoserverWorkspace(Service service, GeoserverRestProxy geoserverConfig, boolean removeAll) {
if (removeAll) {
pdokBuilder.removeWorkspace(service, geoserverConfig);
}
if (!pdokBuilder.workSpaceExist(service, geoserverConfig)) {
pdokBuilder.createWorkspace(service, geoserverConfig);
}
pdokBuilder.createStores(service, geoserverConfig);
pdokBuilder.createLayers(service, geoserverConfig);
pdokBuilder.createCoverages(service, geoserverConfig);
pdokBuilder.createGroupLayer(service, geoserverConfig);
pdokBuilder.createServiceSettings(service, geoserverConfig);
}
private void getGroupLayers(nl.pdok.catalogus.model.CatalogusEntry catalogusEntry, Service service) {
if ( catalogusEntry.getServiceinfo().getGrouplayers() != null) {
for ( nl.pdok.catalogus.model.GroupLayer catalogusGroupLayer : catalogusEntry.getServiceinfo().getGrouplayers()){
GroupLayer groupLayer = service.addGroupLayer(catalogusGroupLayer.getName(), catalogusGroupLayer.getTitle(), catalogusGroupLayer.getDescription());
if ( "GROUPED".equals( catalogusGroupLayer.getType() ) || "CONTAINER".equals( catalogusGroupLayer.getType() ) )
groupLayer.setGrouplLayerType(GroupLayer.GrouplLayerType.CONTAINER);
if ( "SINGLE".equals( catalogusGroupLayer.getType() ) )
groupLayer.setGrouplLayerType(GroupLayer.GrouplLayerType.SINGLE);
for ( GroupLayerLayer catalogLayer : catalogusGroupLayer.getLayers() ){
// single of grouplayer
for (SingleLayer singlelayer : service.getLayers()) {
boolean isSinglelayer = catalogLayer.getLayername().equals(singlelayer.getName());
if (isSinglelayer) {
DbLayer layer = new DbLayer(catalogLayer.getLayername(), new Style(catalogLayer.getStyle()), catalogus);
groupLayer.addLayer((Layer) layer);
}
}
for (GroupLayer grouplayer : service.getGroupLayers()) {
boolean isGrouplayer = catalogLayer.getLayername().equals(grouplayer.getName());
if (isGrouplayer) {
GroupLayer layer = new GroupLayer(catalogus, catalogLayer.getLayername());
groupLayer.addLayer((Layer) layer);
}
}
}
}
}
}
private void getLayersAndCoverages(nl.pdok.catalogus.model.CatalogusEntry catalogusEntry, Service service) {
for ( nl.pdok.catalogus.model.Layer catalogusLayer : catalogusEntry.getServiceinfo().getLayers() ) {
String datasetMetadataId = null;
String datastoreName = null;
for ( nl.pdok.catalogus.model.Dataset catalogusDataset : catalogusEntry.getDatasets()){
if (catalogusDataset.getName().equals(catalogusLayer.getDataset_name())){
datasetMetadataId = catalogusDataset.getMetadata_id();
datastoreName = catalogusDataset.getName();
Style defaultStyle = new Style(catalogusLayer.getDefault_style());
List