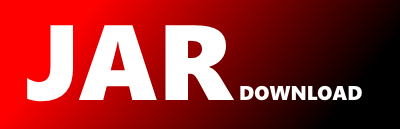
nl.pdok.catalogus.Catalogus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geoserver-workspace-builder Show documentation
Show all versions of geoserver-workspace-builder Show documentation
PDOK Geoserver Workspace Builder
The newest version!
package nl.pdok.catalogus;
import nl.pdok.catalogus.model.CatalogusEntry;
import java.io.File;
import java.io.IOException;
import org.codehaus.jackson.JsonParseException;
import org.codehaus.jackson.map.JsonMappingException;
import org.codehaus.jackson.map.ObjectMapper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Created with IntelliJ IDEA.
* User: nijhur
* Date: 4-11-14
* Time: 10:08
* To change this template use File | Settings | File Templates.
*/
public class Catalogus {
final static Logger logger = LoggerFactory.getLogger(Catalogus.class);
private static String COMMONLOCATION = "pdok_common";
private static String CONFIGURATION_FILE = "configuration.json";
private static String SEPARATOR = File.separator;
private static String SLD_FOLDER = "slds";
private static String SLD_EXT = ".sld";
private String catalogRoot;
public Catalogus(String catalogRoot) {
this.catalogRoot = catalogRoot;
}
public CatalogusEntry getCatalogus(String serviceName){
CatalogusEntry catalogus = null;
ObjectMapper mapper = new ObjectMapper();
try {
File configFile = new File(catalogRoot + SEPARATOR + "datasets" + SEPARATOR + serviceName + SEPARATOR + CONFIGURATION_FILE);
if (configFile.exists() ) {
catalogus = mapper.readValue(configFile, CatalogusEntry.class);
} else {
logger.error("Config file does not exist in catalog: " + serviceName);
}
} catch (JsonMappingException e1) {
logger.error("Error mapping catalog file: " + e1.getMessage());
catalogus = null;
} catch (JsonParseException e1) {
logger.error("Error parsing catalog file: " + e1.getMessage());
catalogus = null;
} catch (IOException e1) {
logger.error("Error reading catalog file: " + e1.getMessage());
catalogus = null;
}
return catalogus;
}
public File getLocalStyle(String serviceName, String styleName){
return getFile(catalogRoot + SEPARATOR + "datasets" + SEPARATOR + serviceName + SEPARATOR + SLD_FOLDER + SEPARATOR + styleName + SLD_EXT);
}
public File getCommonStyle(String styleName){
return getFile(catalogRoot + SEPARATOR + COMMONLOCATION + SEPARATOR + SLD_FOLDER + SEPARATOR + styleName + SLD_EXT);
}
private File getFile(String location){
File file = new File(location);
return file.exists()?file:null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy