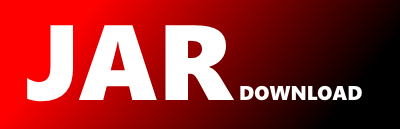
nl.pdok.workspacebuilder.model.Service Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geoserver-workspace-builder Show documentation
Show all versions of geoserver-workspace-builder Show documentation
PDOK Geoserver Workspace Builder
The newest version!
package nl.pdok.workspacebuilder.model;
import java.util.ArrayList;
import java.util.List;
import nl.pdok.catalogus.Catalogus;
import nl.pdok.catalogus.model.CatalogusEntry;
import nl.pdok.workspacebuilder.model.datastore.Datastore;
import nl.pdok.workspacebuilder.model.layer.DbLayer;
import nl.pdok.workspacebuilder.model.layer.GroupLayer;
import nl.pdok.workspacebuilder.model.layer.Layer;
import nl.pdok.workspacebuilder.model.layer.Coverage;
import nl.pdok.workspacebuilder.model.layer.SingleLayer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Created with IntelliJ IDEA.
* User: nijhur
* Date: 4-3-14
* Time: 14:41
* To change this template use File | Settings | File Templates.
*/
public class Service {
final static Logger logger = LoggerFactory.getLogger(Service.class);
public enum ServiceType { WFS, WMS, WMTS, WCS, TMS, ATOM }
private String title;
private String workspaceName;
private String authorityName;
private String authorityUrl;
private Boolean inspire = false;
private List layers;
private List groupLayers;
private List coverages;
private List serviceSettings;
private List datastores;
private List requestSrsList;
private List responseSrsList;
private Catalogus catalogus;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Boolean getInspire() {
return inspire;
}
public void setInspire(Boolean inspire) {
this.inspire = inspire;
}
public Service(String serviceName, Catalogus catalogus){
logger.info("== Start configuring service: " + serviceName);
this.catalogus = catalogus;
CatalogusEntry catalogusEntry = this.catalogus.getCatalogus(serviceName);
if ( catalogusEntry == null ) {
logger.error("No valid Catalog found for: " + serviceName);
return;
}
workspaceName = catalogusEntry.getWorkspace();
layers = new ArrayList();
coverages = new ArrayList();
groupLayers = new ArrayList();
serviceSettings = new ArrayList();
datastores = new ArrayList();
setTitle(catalogusEntry.getServiceinfo().getName());
setInspire(catalogusEntry.getServiceinfo().isInspire());
setAuthorityName(catalogusEntry.getServiceinfo().getAuthority_name());
setAuthorityUrl(catalogusEntry.getServiceinfo().getAuthority_url());
}
public List getServiceSettings() {
return serviceSettings;
}
public String getWorkspaceName() {
return workspaceName;
}
public SingleLayer createDbLayer(Catalogus catalogus) {
return new DbLayer(catalogus);
}
public Coverage createCoverage(Catalogus catalogus) {
return new Coverage(catalogus);
}
public void fillCoverageProperties(Coverage coverage, nl.pdok.catalogus.model.Layer catalogusLayer,
String datastoreName, String metadataId, Style defaultStyle,
List