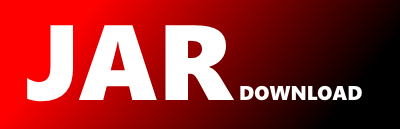
nl.pdok.shapeprocessing.data.shapes.FeatureToTableLoader Maven / Gradle / Ivy
The newest version!
package nl.pdok.shapeprocessing.data.shapes;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
public class FeatureToTableLoader{
private final static String FEATURETOTABLE = "featureToTable";
@SuppressWarnings("unchecked")
public static FeatureToTable LoadFeatureToTableFromJSON(Path featuresToTablesPath) throws Exception {
FeatureToTable featuresToTables = new FeatureToTable();
JSONObject featuresToTableJson = getJSONObject(featuresToTablesPath);
if (featuresToTableJson == null) {
return featuresToTables;
}
try {
JSONObject featuresToTablesData = (JSONObject) featuresToTableJson.get(FEATURETOTABLE);
Set sources = featuresToTablesData.keySet();
for(String source : sources) {
Object list = featuresToTablesData.get(source);
List tables = (List)list;
featuresToTables.getFeatureToTables().put(source, tables);
}
for(Iterator iterator = featuresToTableJson.keySet().iterator(); iterator.hasNext();) {
String key = (String) iterator.next();
if(key.equals(FEATURETOTABLE)) {
continue;
}
Map table = simpleJsonMapToJavaMap((JSONObject)featuresToTableJson.get(key));
featuresToTables.getTableDefinitions().put(key, table);
}
}
catch (ClassCastException e) {
throw new Exception("Invalid data");
}
return featuresToTables;
}
@SuppressWarnings("unchecked")
private static Map simpleJsonMapToJavaMap(JSONObject featureAttributesJson) {
if(featureAttributesJson == null){
return null;
}
Map featureAttributesMap = new HashMap();
Set types = featureAttributesJson.keySet();
for (String type : types) {
featureAttributesMap.put(type, (String) featureAttributesJson.get(type));
}
return featureAttributesMap;
}
private static JSONObject getJSONObject(Path featuresToTablesFile) throws Exception {
JSONObject featuresToTableJson = (JSONObject) (new JSONParser()).parse(Files.newBufferedReader(featuresToTablesFile, StandardCharsets.UTF_8));
if(featuresToTableJson == null || !featuresToTableJson.containsKey(FEATURETOTABLE)) {
return null;
}
return featuresToTableJson;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy