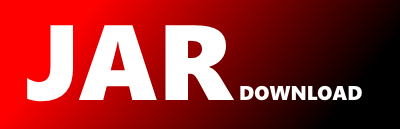
nl.pdok.shapeprocessing.data.shapes.FeatureToTableMappingLoader Maven / Gradle / Ivy
The newest version!
package nl.pdok.shapeprocessing.data.shapes;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
public class FeatureToTableMappingLoader {
private static final String FEATURETOTABLE = "featureToTableMapping";
public FeatureToTableMappingLoader() {
}
public static FeatureToTableMapping LoadFeatureToTableFromJSON(Path featuresToTablesPath) throws Exception {
FeatureToTableMapping featuresToTables = new FeatureToTableMapping();
JSONObject featuresToTableJson = getJSONObject(featuresToTablesPath);
if(featuresToTableJson == null) {
return featuresToTables;
} else {
try {
JSONObject e = (JSONObject)featuresToTableJson.get(FEATURETOTABLE);
Set sources = e.keySet();
Iterator iterator = sources.iterator();
String key;
while(iterator.hasNext()) {
key = (String)iterator.next();
Object table = e.get(key);
String tableName = (String)table;
featuresToTables.getFeatureToTables().put(key, tableName);
}
JSONObject attributes = (JSONObject)featuresToTableJson.get("attributes");
Set attSources = attributes.keySet();
Iterator attIterator = attSources.iterator();
while(attIterator.hasNext()) {
key = (String)attIterator.next();
String value = (String) attributes.get(key);
featuresToTables.getAttributes().put(key, value);
}
return featuresToTables;
} catch (ClassCastException var9) {
throw new Exception("Invalid data");
}
}
}
private static Map simpleJsonMapToJavaMap(JSONObject featureAttributesJson) {
if(featureAttributesJson == null) {
return null;
} else {
HashMap featureAttributesMap = new HashMap();
Set types = featureAttributesJson.keySet();
Iterator i$ = types.iterator();
while(i$.hasNext()) {
String type = (String)i$.next();
featureAttributesMap.put(type, (String)featureAttributesJson.get(type));
}
return featureAttributesMap;
}
}
private static JSONObject getJSONObject(Path featuresToTablesFile) throws Exception {
JSONObject featuresToTableJson = (JSONObject)(new JSONParser()).parse(Files.newBufferedReader(featuresToTablesFile, StandardCharsets.UTF_8));
return featuresToTableJson != null && featuresToTableJson.containsKey(FEATURETOTABLE)?featuresToTableJson:null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy