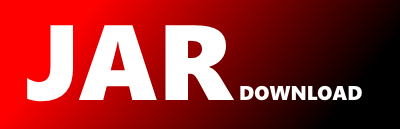
nl.pdok.shapeprocessing.data.shapes.ShapesProcessor Maven / Gradle / Ivy
The newest version!
package nl.pdok.shapeprocessing.data.shapes;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.geotools.data.*;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.geotools.data.simple.SimpleFeatureSource;
import org.geotools.feature.AttributeTypeBuilder;
import org.geotools.feature.simple.SimpleFeatureTypeBuilder;
import org.opengis.feature.Property;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.feature.type.AttributeDescriptor;
import org.opengis.referencing.crs.CoordinateReferenceSystem;
public class ShapesProcessor {
public static void loadShapeToDB(File shapeFile,
String host,
int port,
String database,
String user,
String password,
String schema,
FeatureToTable featureToTable,
String encoding)
throws Exception {
Map shapeLookup = new HashMap();
shapeLookup.put("url", shapeFile.toURI().toString());
if (encoding != null && !encoding.equals("")) {
shapeLookup.put("charset", encoding);
}
DataStore shapeStore = DataStoreFinder.getDataStore(shapeLookup);
Map pgDbLookup = new HashMap<>();
pgDbLookup.put("dbtype", "postgis");
pgDbLookup.put("host", host);
pgDbLookup.put("port", port);
pgDbLookup.put("database", database);
pgDbLookup.put("user", user);
pgDbLookup.put("passwd", password);
pgDbLookup.put("schema", schema);
DataStore dbStore = DataStoreFinder.getDataStore(pgDbLookup);
loadShape(shapeStore, dbStore, featureToTable);
}
public static void loadShape(DataStore shapeStore, DataStore dataStore, FeatureToTable featureToTable)
throws Exception {
try{
String typeName = shapeStore.getTypeNames()[0]; // shapefile always contains 1 feature!
SimpleFeatureSource featureSource = shapeStore.getFeatureSource(typeName);
List targetFeatureTypes = getTargetFeatureTypes(featureSource, dataStore,
typeName, featureToTable);
for (SimpleFeatureType type : targetFeatureTypes) {
writeFeatures(featureSource, dataStore, type, featureToTable);
}
}
finally{
if(dataStore != null){
dataStore.dispose();
}
if(shapeStore != null){
shapeStore.dispose();
}
}
}
private static void writeFeatures(SimpleFeatureSource featureSource, DataStore dataStore,
SimpleFeatureType targetFeatureType, FeatureToTable featureToTable) throws Exception {
SimpleFeatureCollection featureSourceCollection = featureSource.getFeatures();
SimpleFeatureIterator featureSourceIterator = featureSourceCollection.features();
Transaction transaction = new DefaultTransaction(targetFeatureType.getTypeName());
FeatureWriter,?> writer = dataStore.getFeatureWriterAppend(targetFeatureType.getTypeName(), transaction);
int featureCount = 0;
while (featureSourceIterator.hasNext()) {
featureCount++;
SimpleFeature sourceFeature = featureSourceIterator.next();
SimpleFeature targetFeature = (SimpleFeature) writer.next();
writeFeature(sourceFeature, targetFeature, targetFeatureType, featureToTable);
writer.write();
if (featureCount % 10000 == 0) {
transaction.commit();
}
}
try {
transaction.commit();
}
finally {
transaction.close();
}
writer.close();
featureSourceIterator.close();
}
private static void writeFeature(SimpleFeature sourceFeature, SimpleFeature targetFeature,
SimpleFeatureType targetFeatureType, FeatureToTable featuresToTablesFile) throws Exception {
Map targetTableAttributes = getAttributes(targetFeatureType.getName().getLocalPart(),
featuresToTablesFile);
if (targetTableAttributes != null && targetTableAttributes.size() > 0) {
Set attributes = targetTableAttributes.keySet();
Set sourceProperties = new HashSet();
for (Property sourcePropertie : sourceFeature.getProperties()) {
sourceProperties.add(sourcePropertie.getName().getLocalPart());
}
for (String attribute : attributes) {
if (sourceProperties.contains(attribute)) {
targetFeature.setAttribute(targetTableAttributes.get(attribute),
createNullFromEmptyString(sourceFeature.getAttribute(attribute)));
}
}
} else {
for (Property propertieName : sourceFeature.getProperties()) {
targetFeature.setAttribute(propertieName.getName().getLocalPart().toLowerCase(),
createNullFromEmptyString(sourceFeature.getAttribute(propertieName.getName().getLocalPart())));
}
}
}
private static Object createNullFromEmptyString(Object valueToCheck) {
if ("".equals(valueToCheck)) {
return null;
}
return valueToCheck;
}
private static List getTargetFeatureTypes(SimpleFeatureSource featureSource,
DataStore dataStore, String sourceTypeName, FeatureToTable featureToTable) throws Exception {
List targetTables = getTables(sourceTypeName, featureToTable);
List simpleFeatureTypes = new ArrayList();
for (String targetTable : targetTables) {
SimpleFeatureType simpleFeatureType = null;
List typeNamesList = Arrays.asList(dataStore.getTypeNames());
Map targetTableAttributes = getAttributes(targetTable, featureToTable);
if (targetTableAttributes != null) {
if (typeNamesList.contains(targetTable)) {
simpleFeatureType = dataStore.getSchema(targetTable);
} else {
SimpleFeatureTypeBuilder featureBuilder = new SimpleFeatureTypeBuilder();
featureBuilder.setName(targetTable);
featureBuilder.setNamespaceURI(featureSource.getSchema().getName().getNamespaceURI());
CoordinateReferenceSystem sourceCRS = featureSource.getSchema()
.getCoordinateReferenceSystem();
featureBuilder.setCRS(sourceCRS);
List attributeSourceDescriptions = featureSource.getSchema()
.getAttributeDescriptors();
Set keys = targetTableAttributes.keySet();
for (AttributeDescriptor attributeSourceDescription : attributeSourceDescriptions) {
if (keys.contains(attributeSourceDescription.getName().getLocalPart())) {
String atrributeName = targetTableAttributes
.get(attributeSourceDescription.getName().getLocalPart());
AttributeTypeBuilder builder = new AttributeTypeBuilder();
builder.init(attributeSourceDescription);
builder.setName(atrributeName);
featureBuilder.add(builder.buildDescriptor(atrributeName));
}
}
simpleFeatureType = featureBuilder.buildFeatureType();
}
} else {
simpleFeatureType = DataUtilities.createSubType(featureSource.getSchema(), null,
featureSource.getSchema().getCoordinateReferenceSystem(), targetTable, null);
}
simpleFeatureTypes.add(simpleFeatureType);
if (!typeNamesList.contains(simpleFeatureType.getTypeName())) {
dataStore.createSchema(simpleFeatureType);
}
}
return simpleFeatureTypes;
}
private static List getTables(String sourceTypeName, FeatureToTable featureToTable) throws Exception {
List tables = null;
Set patternStrings = featureToTable.getFeatureToTables().keySet();
for (String patternString : patternStrings) {
Pattern pattern = Pattern.compile(patternString);
Matcher matcher = pattern.matcher(sourceTypeName);
if (matcher.find()) {
tables = (List) featureToTable.getFeatureToTables().get(patternString);
}
}
if (tables == null) {
tables = new ArrayList();
}
return tables;
}
private static Map getAttributes(String targetTable, FeatureToTable featureToTable)
throws Exception {
if (featureToTable.getTableDefinitions().containsKey(targetTable)) {
return featureToTable.getTableDefinitions().get(targetTable);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy