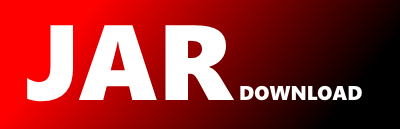
nl.psek.fitnesse.fixtures.database.ResultSetManager Maven / Gradle / Ivy
package nl.psek.fitnesse.fixtures.database;
import nl.psek.fitnesse.ConditionalExceptionType;
import java.sql.ResultSet;
import java.sql.SQLException;
import static nl.psek.fitnesse.fixtures.general.util.OperationsFixture.areValuesEqual;
import static nl.psek.fitnesse.fixtures.util.FixtureOperations.matchOnPattern;
/**
* @author Ronald Mathies
*/
public class ResultSetManager {
private ResultSet resultSet;
public ResultSetManager() {}
public void setResultSet(ResultSet resultSet) {
try {
if (this.resultSet != null && !this.resultSet.isClosed()) {
this.resultSet.close();
}
this.resultSet = resultSet;
} catch (SQLException sqlException) {
ConditionalExceptionType.fail("Could not close resultset due to exception '%s'.", sqlException.getMessage());
}
}
public ResultSet getResultSet() {
return this.resultSet;
}
public int getNumberOfRows() {
isOpen();
try {
this.resultSet.last();
return this.resultSet.getRow();
} catch (SQLException sqlException) {
ConditionalExceptionType.fail("There was a problem counting the number of rows in the resultset '%s'.", sqlException.getMessage());
}
return 0;
}
public boolean verifyNumberOfRows(int expectedNumberOfRows) {
return areValuesEqual(getNumberOfRows(), expectedNumberOfRows);
}
public Object getValue(int index, String column)
{
isOpen();
if (index == 0) {
ConditionalExceptionType.fail("Cannot get value from row 0, the row count starts with 1.");
}
try {
this.resultSet.absolute(index);
return this.resultSet.getObject(column);
} catch (SQLException sqlException) {
ConditionalExceptionType.fail("There was a problem getting a value out of the resultset '%s'.", sqlException.getMessage());
}
return null;
}
public boolean verifyValue(int index, String column, String pattern) {
String foundValue = getValue(index, column).toString();
return matchOnPattern(foundValue, pattern);
}
private void isOpen() {
try {
if (resultSet.isClosed()) {
ConditionalExceptionType.fail("Resultset is closed, cannot retrieve any information.");
}
} catch (SQLException sqlException) {
ConditionalExceptionType.fail("There was a problem checking if the resultset is still open '%s'.", sqlException.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy