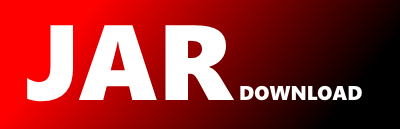
nl.psek.fitnesse.fixtures.database.SqlExecutor Maven / Gradle / Ivy
package nl.psek.fitnesse.fixtures.database;
import nl.psek.fitnesse.ConditionalExceptionType;
import nl.psek.fitnesse.fixtures.util.FileFinder;
import nl.psek.fitnesse.fixtures.util.FileHandler;
import org.apache.ibatis.jdbc.ScriptRunner;
import org.apache.log4j.Logger;
import java.io.*;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
/**
* @author Ronald Mathies, Pascal Smeets
*/
public class SqlExecutor {
private static final Logger LOG = Logger.getLogger(SqlExecutor.class);
/**
* Executes the contents of the sql file using the specified connection.
*
* @param connection the connection to use.
* @param sqlFile the sql file to execute.
*
* @throws ConditionalExceptionType when the execution fails.
*/
public static void execute(final Connection connection, String sqlFile) {
FileFinder.findFileInUserDirectory(sqlFile, new FileHandler() {
@Override
public void handle(File file) {
try {
process(connection, file);
} catch (IOException e) {
ConditionalExceptionType.fail("Failed to import file '%s' due to the following reason '%s'.", file, e.getMessage());
}
}
});
}
private static void process(Connection connection, File sqlFile) throws IOException {
ScriptRunner runner = new ScriptRunner(connection);
runner.setAutoCommit(true);
InputStream commentInputStream = new ByteArrayInputStream("-- Empty comment for SQL Server.\n\r".getBytes());
SequenceInputStream sequenceInputStream = new SequenceInputStream(commentInputStream, new FileInputStream(sqlFile));
BufferedReader reader = new BufferedReader(new InputStreamReader(sequenceInputStream));
runner.runScript(reader);
}
public static void processString(Connection connection, String sqlString) throws SQLException {
LOG.info("Processing SQL query '" + sqlString + "'");
try (PreparedStatement statement = connection.prepareStatement(sqlString)) {
statement.execute();
} catch (SQLException e) {
ConditionalExceptionType.fail(e.getMessage());
}
}
public static int processStringWithResults(Connection connection, String sqlString) {
int rowsAffected = 0;
LOG.info("Processing SQL query '" + sqlString + "'");
try (PreparedStatement statement = connection.prepareStatement(sqlString)) {
rowsAffected = statement.executeUpdate();
LOG.info("Number of rows affected for query '" + sqlString + "' is: " + rowsAffected);
} catch (SQLException e) {
ConditionalExceptionType.fail(e.getMessage());
}
return rowsAffected;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy