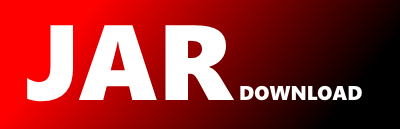
nl.psek.fitnesse.fixtures.selenium.SeleniumFixture Maven / Gradle / Ivy
package nl.psek.fitnesse.fixtures.selenium;
import nl.psek.fitnesse.fixtures.selenium.browsers.*;
import org.openqa.selenium.Capabilities;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.safari.SafariDriver;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.time.Duration;
import java.util.ArrayList;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureUtils.*;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureWaits.DEFAULTFLUENTWAITTIMEOUT;
import static nl.psek.fitnesse.fixtures.util.FixtureOperations.getFromPropertyIfApplicable;
/**
* This class uses Selenium 3 to automate webpages, implementation of methods is done in the other classes in this package.
*
*
* It has been refactored from the SlimWebDriver class. All methods are present, but the setup of Webdriver/the browser is different.
*
* Setup is done programatically in your testcase / scenario
*
* ### an example using Chrome:
*
*
* !|script |
* |start |SeleniumFixture |
* |setDriverLocation; |webdriver.chrome.driver|src/test/tooling/win/chromedriver.exe |
* |addChromeExperimentalOptionAsArray;|excludeSwitches |enable-automation |
* |addChromeOption; |window-size=1024,768 |
* |setChromeHeadless; |true |
* |#setChromeProxy; |host |port |
* |setBrowser; |chrome |
* |setScreenshotLocation; |src/test/resources/FitnesseRoot/files/screenshots;target/fitnesse-test-results/files/screenshots|
* |circumventSelenium3Limitations; |
* |defaultCommandTimeout; |5 |
* |defaultPageLoadTimeout; |30 |
* |openWebsite; |https://example.com |
* |show |takeScreenshot; |
* |closeWebsite; |
*
*
*
* ### an example using Firefox:
*
*
* !|script |
* |start |SeleniumFixture |
* |setDriverLocation; |webdriver.gecko.driver|src/test/tooling/win/geckodriver.exe |
* |addFireFoxOption; |-headless |
* |setFireFoxProxy; |proxy1.kvk.nl |8080 |
* |setBrowser; |firefox |
* |setScreenshotLocation; |src/test/resources/FitnesseRoot/files/screenshots;target/fitnesse-test-results/files/screenshots|
* |circumventSelenium3Limitations; |
* |defaultCommandTimeout; |5 |
* |defaultPageLoadTimeout;|5 |
* |openWebsite; |https://example.com |
* |show |takeScreenshot; |
* |closeWebsite; |
*
*
* ### an example using a Remote Selenium instance (grid):
*
*
* !|script |
* |start |SeleniumFixture |
* |setRemoteServerUrl; |https://boss-selenium-test.svc.npo.kvk.nl/ |
* |setRemoteBrowser; |chrome| |
* |addRemoteChromeOption; |window-size=1024,768 |
* |setBrowser; |remote |
* |setScreenshotLocation; |src/test/resources/FitnesseRoot/files/screenshots|
* |defaultCommandTimeout; |5 |
* |defaultPageLoadTimeout;|5 |
* |openWebsite; |https://jira.kvk.nl |
* |show |takeScreenshot; |
* |closeWebsite; |
*/
public class SeleniumFixture extends SeleniumFixtureCommon {
private static final Logger LOG = LoggerFactory.getLogger(SeleniumFixture.class);
private static ArrayList loopCommands = new ArrayList<>();
private static String browser = "";
/**
* Instantiates a new Selenium fixture.
*/
public SeleniumFixture() {
SYSTEMISMACOS = isSystemMacOs();
setCmdKeyifMacOs();
}
/** @name Setup of Webdriver
* How to set up WebDriver
*/
///@{
/** @name setup_firefox
* ## Setup for Firefox
* Use these commands to customise the Firefox driver
*/
///@{
/**
* Add firefox option.
*
* @param option the option
*/
public void addFireFoxOption(String option) {
initFireFoxDriver.addFireFoxOption(option);
}
/**
* Add fire fox string preference.
*
* @param key the key
* @param value the value
*/
public void addFireFoxStringPreference(String key, String value) {
initFireFoxDriver.addFireFoxStringPreference(key, value);
}
/**
* Add firefox int preference.
*
* @param key the key
* @param value the value
*/
public void addFireFoxIntPreference(String key, int value) {
initFireFoxDriver.addFireFoxIntPreference(key, value);
}
/**
* Add firefox boolean preference.
*
* @param key the key
* @param value the value
*/
public void addFireFoxBooleanPreference(String key, boolean value) {
initFireFoxDriver.addFireFoxBooleanPreference(key, value);
}
/**
* Sets firefox proxy.
*
* @param proxyhost the proxyhost
* @param proxyport the proxyport
*/
public void setFireFoxProxy(String proxyhost, String proxyport) {
initFireFoxDriver.setFireFoxProxy(proxyhost, proxyport);
}
/**
* Sets firefox profile.
*
* @param profileFolder the profile folder
*/
public void setFireFoxProfile(String profileFolder) {
initFireFoxDriver.setFireFoxProfile(profileFolder);
}
///@}
/** @name setup_chrome
* ## Setup for Chrome
*
* Use these commands to customise the Chrome driver
*/
///@{
/**
* Add chrome option.
*
* Look for capabilities and ChromeOptions at:
*
* - https://chromedriver.chromium.org/capabilities
* - list of arguments: https://peter.sh/experiments/chromium-command-line-switches/
*
* @param option the option
*/
public void addChromeOption(String option) {
initChromeDriver.addChromeOption(option);
}
/**
* Add chrome experimental option.
*
* @param name the name
* @param value the value
*/
public void addChromeExperimentalOption(String name, Object value) {
initChromeDriver.addChromeExperimentalOption(name, value);
}
/**
* Add chrome experimental option as array.
*
* @param name the name
* @param value the value
*/
public void addChromeExperimentalOptionAsArray(String name, Object value) {
initChromeDriver.addChromeExperimentalOptionAsArray(name, value);
}
/**
* Sets chrome headless.
*
* @param headless the headless
*/
public void setChromeHeadless(boolean headless) {
initChromeDriver.setChromeHeadless(headless);
}
/**
* Sets chrome proxy.
*
* @param proxyhost the proxyhost
* @param proxyport the proxyport
*/
public void setChromeProxy(String proxyhost, String proxyport) {
initChromeDriver.setChromeProxy(proxyhost, proxyport);
}
///@}
/** @name setup_safari
* ## Setup for Safari
*
* Use these commands to customise the Safari driver
*/
///@{
/**
* Add safari capability.
*
* @param key the key
* @param value the value
*/
public void addSafariCapability(String key, String value) {
initSafariDriver.addSafariCapability(key, value);
}
/**
* Add safari boolean capability.
*
* @param key the key
* @param value the value
*/
public void addSafariBooleanCapability(String key, boolean value) {
initSafariDriver.addSafariBooleanCapability(key, value);
}
/**
* Sets safari automatic inspection.
*
* @param value the value
*/
public void setSafariAutomaticInspection(boolean value) {
initSafariDriver.setSafariAutomaticInspection(value);
}
/**
* Sets safari automatic profiling.
*
* @param value the value
*/
public void setSafariAutomaticProfiling(boolean value) {
initSafariDriver.setSafariAutomaticInspection(value);
}
/**
* Sets safari proxy.
*
* @param proxyhost the proxyhost
* @param proxyport the proxyport
*/
public void setSafariProxy(String proxyhost, String proxyport) {
initSafariDriver.setSafariProxy(proxyhost, proxyport);
}
///@}
/** @name setup_browserstack
* ## Setup for a remote Browserstack instance
*
* Use these commands to customise the remote browserstack driver
*/
///@{
/**
* Sets the url to the browserstack instance, should look like this
* https://" + USERNAME + ":" + AUTOMATE_KEY + "@hub-cloud.browserstack.com/wd/hub";
*
* @param url the url to the browserstack instance
*/
public void setBrowserStackUrl(String url) {
initBrowserstackDriver.setBrowserStackUrl(url);
}
/**
* Add desired capability for use with browserstack.
*
* @param key the key
* @param value the value
*/
public void addBrowserstackDesiredCapability(String key, String value) {
initBrowserstackDriver.addDesiredCapability(key, value);
}
///@}
/** @name setup_remote
* ## Setup for a remote Selenium instance
*
* Use these commands to customise the remote driver
*/
///@{
/**
* Sets the url of the remote selenium instance (without wd/hub)
*
* @param url your selenium instance url
*/
public void setRemoteServerUrl(String url) {
initRemoteWebDriver.setRemoteServerUrl(url);
}
/**
* Sets the remote browser to be used: chrome of firefox
*
* @param browsername chrome or firefox
* @param version can be left empty
*/
public void setRemoteBrowser(String browsername, String version) {
initRemoteWebDriver.setRemoteBrowser(browsername, version);
}
/**
* Add desired capability.
*
* @param key the key
* @param value the value
*/
public void addDesiredCapability(String key, String value) {
initRemoteWebDriver.addDesiredCapability(key, value);
}
/**
* Add remote chrome option.
*
* @param option the option
*/
public void addRemoteChromeOption(String option) {
initRemoteWebDriver.addRemoteChromeOption(option);
}
/**
* Set hte remote Chrome pageload strategy
*
* @param strategy none, normal or eager
*/
public void setRemoteChromePageLoadStrategy(String strategy) {
initRemoteWebDriver.setRemoteChromePageLoadStrategy(strategy);
}
/**
* Sets the remote proxy
*
* @param proxyhost the host
* @param proxyport the port
*/
public void setRemoteProxy(String proxyhost, String proxyport) {
initRemoteWebDriver.setRemoteProxy(proxyhost, proxyport);
}
///@}
/** @name setup_general
* # General setup
*
* Use these commands to set the driver location AND select the previously configured driver instance with "setBrowser"
*
*/
///@{
/**
* Location of the driver executable
*
* @param driverName "webdriver.chrome.driver" or "webdriver.gecko.driver"
* @param driverLocation full path to the executable driver file
*/
public void setDriverLocation(String driverName, String driverLocation) {
System.setProperty(driverName, driverLocation);
}
/**
* Some methods allow for overriding of Selenium 3 functionality. Use this command to allow this.
* For example: Selenium won't click on an element if there is an overlay present, with this command set the click command will attempt to use JavaScript to click on the specified element.
*/
public void circumventSelenium3Limitations() {
SeleniumFixtureCommon.circumventSelenium3LimitationsUsingJavascriptToPerformActionIfElementNotAccessible();
}
/**
* Restores to Selenium 3 default
*/
public void restoreSelenium3Limitations() {
SeleniumFixtureCommon.restoreSelenium3LimitationsDontUseJavascriptLetSeleniumDoItsTheDefaultThing();
}
/**
* Sets screenshot location.
*
* @param path the path
*/
public void setScreenshotLocation(String path) {
SCREENSHOTLOCATION = path;
}
/**
* Starts WebDriver using the specified browser: chrome, firefox, safari, browserstack or remote
*
* @param browser chrome, firefox, safari, browserstack or remote
*/
public void startWebDriver(String browser) {
LOG.info("Using browser: " + browser);
Capabilities caps;
this.browser = browser;
switch (browser.toLowerCase()) {
case "chrome":
driver = initChromeDriver.getChromeDriver();
caps = ((ChromeDriver) driver).getCapabilities();
break;
case "firefox":
driver = initFireFoxDriver.getFireFoxDriver();
caps = ((FirefoxDriver) driver).getCapabilities();
break;
case "safari":
driver = initSafariDriver.getSafariDriver();
caps = ((SafariDriver) driver).getCapabilities();
case "browserstack":
driver = initBrowserstackDriver.getBrowserstackDriver();
caps = ((RemoteWebDriver) driver).getCapabilities();
case "remote":
driver = initRemoteWebDriver.getRemoteWebDriver();
caps = ((RemoteWebDriver) driver).getCapabilities();
break;
default:
throw new SeleniumFixtureException("Unsupported browser at this time %s", browser);
}
LOG.info("This driver supports xpath version: " + getSupportedXPathVersion(driver));
LOG.info("This driver supports the following capabilities ");
for (Map.Entry entry : caps.asMap().entrySet()) {
LOG.info("capability " + entry.toString());
}
}
///@}
///@}
// FOR USE WITH OTHER FIXTURES
/**
* Returns the currect webdriver for use in other fixture
*
* @return the current webdriver
*/
public WebDriver giveWebDriver() {
return driver;
}
/** @name timeouts
* ## Timeouts
* default waits, timeouts, opening, closing, resizing, etc..
*
*/
///@{
/**
* Register the default timeout that will apply to all commands.
* The selenium impliciyt wait
*
* @param seconds the amount of seconds to wait until the test fails.
* @throws SeleniumFixtureException when the specified seconds is a negative number.
*/
public void setDefaultCommandTimeout(int seconds) {
if (seconds < 1) {
throw new SeleniumFixtureException("Unable to set a negative amount of seconds as the default command timeout.");
} else {
DEFAULTFLUENTWAITTIMEOUT = Duration.ofSeconds(seconds);
driver.manage().timeouts().implicitlyWait(seconds, TimeUnit.SECONDS);
}
}
/**
* Default command timeout.
*
* @param seconds the seconds
*/
@Deprecated
public void defaultCommandTimeout(int seconds) {
setDefaultCommandTimeout(seconds);
}
/**
* Register the default timeout that will apply to page loading actions.
*
* @param seconds the amount of seconds to wait until the page loads
* @throws SeleniumFixtureException when the specified seconds is a negative number.
*/
public void setDefaultPageLoadTimeout(int seconds) {
if (seconds < 1) {
throw new SeleniumFixtureException("Unable to set a negative amount of seconds as the default page load timeout.");
} else {
driver.manage().timeouts().pageLoadTimeout(seconds, TimeUnit.SECONDS);
}
}
/**
* Default page load timeout.
*
* @param seconds the seconds
*/
@Deprecated
public void defaultPageLoadTimeout(int seconds) {
setDefaultPageLoadTimeout(seconds);
}
///@}
/** @name interaction_page
* ## INTERACTION WITH PAGE - WINDOWS AND FRAMES
* opening, closing, resizing, switching frames, etc..
*
*/
///@{
/**
* Open a new window with the given url
*
* @param url the url to call upon opening the new window.
* @throws SeleniumFixtureException when the specified URL is not valid.
*/
public void openWebsite(String url) {
SeleniumFixturePageInteractions.openWebsite(getFromPropertyIfApplicable(url));
}
/**
* Refreshes the current web site..
*/
public void refreshWebsite() {
SeleniumFixturePageInteractions.refreshWebsite();
}
/**
* Close the current window
*/
public void closeWindow() {
SeleniumFixturePageInteractions.closeWindow();
}
/**
* Quit the browser.
*/
public void closeWebsite() {
SeleniumFixturePageInteractions.closeWebsite();
}
/**
* Maximize window.
*/
public void maximizeWindow() {
SeleniumFixturePageInteractions.maximizeWindow();
}
/**
* Resizes the window to the specified dimensions
*
* @param pixelsWidth the pixels width
* @param pixelsHeight the pixels height
*/
public void resizeWindow(int pixelsWidth, int pixelsHeight) {
SeleniumFixturePageInteractions.resizeWindow(pixelsWidth, pixelsHeight);
}
/**
* Moves the window
*
* @param horizontal shift in pixels
* @param vertical shift in pixels
*/
public void moveWindow(int horizontal, int vertical) {
SeleniumFixturePageInteractions.moveWindow(horizontal, vertical);
}
/**
* Sets the window position
*
* @param x the x
* @param y the y
*/
public void setWindowPosition(int x, int y) {
SeleniumFixturePageInteractions.setWindowPosition(x, y);
}
/**
* Performs a pause action using the Actions class
*
* @param millis the time to pause in milliseconds meer informatie http://selenium.googlecode.com/git/docs/api/java/org/openqa/selenium/interactions/Actions.html let op gebruik alleen als het echt nodig is of voor de debug van je fitnesse test. Pascal: DONE
*/
public void sleep(long millis) {
SeleniumFixtureUtils.sleep(millis);
}
/**
* Presses the browser back button.
*/
public void browserBack() {
SeleniumFixturePageInteractions.browserBack();
}
/**
* Presses the browser back button.
*/
public void browserForward() {
SeleniumFixturePageInteractions.browserForward();
}
/**
* Executes the specified javascript in the browser.
*
* @param script the javascript to execute.
* @throws SeleniumFixtureException when the javascript failed to execute.
*/
public void executeJavaScript(String script) {
SeleniumFixturePageInteractions.executeJavaScript(script);
}
/**
* Execute java script with return value string.
*
* @param script the script
* @return the string
*/
public String executeJavaScriptWithReturnValue(String script) {
return SeleniumFixturePageInteractions.executeJavaScriptWithReturnValue(script);
}
///@}
/** @name interaction_frames
* ## INTERACTION WITH PAGE - Working with frames
* opening, closing, resizing, switching frames, etc..
*
*/
///@{
/**
* Pushes a new frame as the current driver.
*
* @param locator the \locator to use to find the web element.
*/
public void switchToFrameUsingLocator(String locator) {
SeleniumFixturePageInteractions.switchToFrameUsingLocator(locator);
}
/**
* Switch to frame using index.
*
* @param index the index
*/
public void switchToFrameUsingIndex(int index) {
SeleniumFixturePageInteractions.switchToFrameUsingIndex(index);
}
/**
* Go back to the initial state.
*/
public void switchToStart() {
SeleniumFixturePageInteractions.switchToStart();
}
/**
* Pushes a new window as the current driver.
*
* @param nameOrHandle the name or id attribute.
*/
public void switchToWindow(String nameOrHandle) {
SeleniumFixturePageInteractions.switchToWindow(nameOrHandle);
}
/**
* Pushes a new window as the current driver using the window title or a regexp as input
* Will retrieve all window handles and switch between them. If the title of the window is found we stop. Otherwise switch back to the original window
*
* @param pattern the expected window title (optionally witg REGEXPI: prefix)
*/
public void switchToWindowByTitle(String pattern) {
SeleniumFixturePageInteractions.switchToWindowByTitle(pattern);
}
/**
* Switches to the opened window that is not the curent window for webdriver.
*/
public void switchToUnfocussedWindow() {
SeleniumFixturePageInteractions.switchToUnfocussedWindow();
}
///@}
/** @name interaction_webelements
* ## INTERACTION WITH PAGE - Webelements
* clicking, typing, selecting, etc..
*
*/
///@{
/**
* Clicks on an element in the page.
*
* @param locator the \locator to use to find the element.
*/
public void click(String locator) {
SeleniumFixturePageInteractions.click(driver, locator);
}
/**
* Click using action.
*
* @param locator the locator
*/
public void clickUsingAction(String locator) {
SeleniumFixturePageInteractions.clickUsingAction(locator);
}
/**
* Richt click / context click action
*
* @param locator the \locator
* @throws SeleniumFixtureException when the element can not be found
*/
public void contextClick(String locator) {
SeleniumFixturePageInteractions.contextClick(locator);
}
/**
* Doubleclicks on an element in the page
*
* @param locator the \locator to use to find the element
* @throws SeleniumFixtureException when the element can not be found
*/
public void doubleClick(String locator) {
SeleniumFixturePageInteractions.doubleClick(locator);
}
/**
* Clicks on an element in the page for a certain number of times..
*
* @param locator the \locator to use to find the element.
* @param numberOfTimesToExecute the number of times to click the element.
* @throws SeleniumFixtureException when the element can not be found.
*/
public void clickUpToTimes(String locator, String numberOfTimesToExecute) {
SeleniumFixturePageInteractions.clickUpToTimes(locator, numberOfTimesToExecute);
}
/**
* Clicks on an element in the page that is not visible i.e. hidden
*
* @param locator the \locator
*/
public void clickUsingJavascript(String locator) {
SeleniumFixturePageInteractions.clickUsingJavascript(driver, locator);
}
/**
* Mag nog mooier
*
* Clicks on an element until a different element becomes present
* WARNING this methods resets the implicit wait time (command timeout) to 15 seconds after completion of the method
*
* @param elementToClick the element to click
* @param elementToWaitFor the element to wait for
* @param commandTimeout - alternative command timeout for this command, needs to be set
* @param maxAttempts the max attempts
*/
public void clickUntilElementPresent(String elementToClick, String elementToWaitFor, long commandTimeout, int maxAttempts) {
}
/**
* Click if other element present.
*
* @param elementToClick the element to click
* @param elementToWaitFor the element to wait for
*/
public void clickIfOtherElementPresent(String elementToClick, String elementToWaitFor) {
SeleniumFixturePageInteractions.clickIfOtherElementPresent(elementToClick, elementToWaitFor);
}
/**
* Click if other element not present.
*
* @param elementToClick the element to click
* @param elementThatShoudBeAbsent the element that shoud be absent
*/
public void clickIfOtherElementNotPresent(String elementToClick, String elementThatShoudBeAbsent) {
SeleniumFixturePageInteractions.clickIfOtherElementNotPresent(elementToClick, elementThatShoudBeAbsent);
}
/**
* Writes the specified value to the element found using the \locator. Will select text in the element first and type over it.
*
* @param locator the \locator to use to find the element.
* @param value the value to write to the element.
* @throws SeleniumFixtureException when the element can not be found or is not an INPUT or TEXTAREA element.
*/
public void type(String locator, String value) {
SeleniumFixturePageInteractions.type(locator, value);
}
/**
* Writes the specified value to the element found using the \locator.
*
* @param locator the \locator to use to find the element.
* @param value the value to write to the element.
* @throws SeleniumFixtureException when the element can not be found or is not an INPUT or TEXTAREA element. Ronald: DONE
*/
public void typeWithClear(String locator, String value) {
SeleniumFixturePageInteractions.typeWithClear(locator, value);
}
/**
* Types a value in an element on the page using Javascript
*
* @param locator the \locator
* @param value the text to be typed
*/
public void typeUsingJavascript(String locator, String value) {
SeleniumFixturePageInteractions.typeUsingJavascript(locator, value);
}
/**
* Types the specified value if the element is present. Can not be used as a test condition - returntype is void. Method will catch all exceptions
* Use in the test preparation steps.
*
* @param locator the \locator
* @param value the value
*/
public void typeIfElementPresent(String locator, String value) {
SeleniumFixturePageInteractions.typeIfElementPresent(locator, value);
}
/**
* Type without clear.
*
* @param locator the \locator
* @param value the value
*/
public void typeWithoutClear(String locator, String value) {
SeleniumFixturePageInteractions.typeWithoutClear(locator, value);
}
/**
* Type key code without clear.
*
* @param locator the \locator
* @param keycode the keycode
*/
public void typeKeyCodeWithoutClear(String locator, Keys keycode) {
SeleniumFixturePageInteractions.typeKeyCodeWithoutClear(locator, keycode);
}
/**
* Type key code using actions.
*
* @param keycode the keycode
*/
public void typeKeyCodeUsingActions(Keys keycode) {
SeleniumFixturePageInteractions.typeKeyCodeUsingActions(keycode);
}
/**
* Tab out of the element found using the locator
*
* @param locator the locator to use to find the element
* @throws SeleniumFixtureException when the element can not be found
*/
public void tab(String locator) {
SeleniumFixturePageInteractions.tab(locator);
}
///@}
/** @name interaction_alerts
* ## INTERACTION WITH PAGE - Alerts
* accepting, dismissing
*
*/
///@{
/**
* Accept alert.
*/
public void acceptAlert() {
SeleniumFixturePageInteractions.acceptAlert();
}
/**
* Dismiss alert.
*/
public void dismissAlert() {
SeleniumFixturePageInteractions.dismissAlert();
}
///@}
/** @name interaction_scrolling
* ## INTERACTION WITH PAGE - Scrolling
* accepting, dismissing
*
*/
///@{
/**
* Scroll to.
*
* @param locator the \locator
*/
public void scrollTo(String locator) {
SeleniumFixturePageInteractions.scrollTo(locator);
}
/**
* Scrolls up to the element using the \locator.
*
* @param locator the \locator to use to find the element.
*/
public void scrollUpTo(String locator) {
SeleniumFixturePageInteractions.scrollUpTo(locator);
}
/**
* Scrolls down to the element using the \locator.
*
* @param locator the \locator to use to find the element.
*/
public void scrollDownTo(String locator) {
SeleniumFixturePageInteractions.scrollDownTo(locator);
}
///@}
/** @name interaction_special
* ## INTERACTION WITH PAGE - Enabling or disabling textboxes
*
*/
///@{
/**
* Enable textbox.
*
* @param locator the \locator
*/
public void enableTextbox(String locator) {
SeleniumFixturePageInteractions.enableTextbox(locator);
}
/**
* Disable textbox.
*
* @param locator the \locator
*/
public void disableTextbox(String locator) {
SeleniumFixturePageInteractions.disableTextbox(locator);
}
///@}
/** @name interaction_upload
* ## INTERACTION WITH PAGE - Uploading files
*
*/
///@{
/**
* Method to send inut to a INPUT element with TYPE="FILE".
* If a RemoteWebdriver is used the file will be streamed to the selenium node.
* If a local Webdriver is used this command will type the filename in the input field.
*
* @param locator the \locator
* @param fileName the file name
*/
public void uploadFile(final String locator, final String fileName) {
SeleniumFixturePageInteractions.uploadFile(browser, locator, fileName);
}
/**
* Method to send inut to a INPUT element with TYPE="FILE".
* If a RemoteWebdriver is used the file will be streamed to the selenium node.
* If a local Webdriver is used this command will type the filename in the input field.
*
* @param locator the \locator
* @param fileLocation the file location
* @param fileName the file name
*/
public void uploadFile(final String locator, String fileLocation, String fileName) {
SeleniumFixturePageInteractions.uploadFile(browser, locator, fileLocation, fileName);
}
///@}
/** @name interaction_selects
* ## INTERACTION WITH PAGE - Checkboxes and Selects
*
*/
///@{
/**
* Checks a checkbox (or radiobutton), if it was checked then nothing will be done.
*
* @param locator the \locator to use to find the element. Ronald: DONE
*/
public void makeChecked(String locator) {
SeleniumFixturePageInteractions.makeChecked(locator);
}
/**
* Unchecks a checkbox (or radiobutton), if it was checked then nothing will be done.
*
* @param locator the \locator to use to find the element. Ronald: DONE
*/
public void makeNotChecked(String locator) {
SeleniumFixturePageInteractions.makeNotChecked(locator);
}
/**
* Selects an option in a select box using the specified label.
*
* @param locator the \locator to use to find the element.
* @param label the label of the option within the select box.
* @throws SeleniumFixtureException when the element could not be found, is not an SELECT element, there is no option with the specified label.
*/
public void selectUsingLabel(String locator, String label) {
SeleniumFixturePageInteractions.selectUsingLabel(locator, label);
}
/**
* Select using label.
*
* @param locator the \locator
* @param label the label
* @param keywordAllow the keyword allow: if supplied als "allowdefault" this method will not throw an exception when the preselected label is selected again
*/
public void selectUsingLabel(String locator, String label, String keywordAllow) {
SeleniumFixturePageInteractions.selectUsingLabel(locator, label, keywordAllow);
}
/**
* Selects the specified value if the element is present. Can not be used as a test condition - returntype is void. Method will catch all exceptions
* Use in the test preparation steps.
*
* @param locator the \locator
* @param label the label
*/
public void selectUsingLabelIfElementPresent(String locator, String label) {
SeleniumFixturePageInteractions.selectUsingLabelIfElementPresent(locator, label);
}
/**
* Deselects an option in a select box using the specified label.
*
* @param locator the \locator to use to find the element.
* @param label the label of the option within the select box.
* @throws SeleniumFixtureException when the element could not be found, is not an SELECT element, there is no option with the specified label or when the option was not selected in the first place.
*/
public void deselectUsingLabel(String locator, String label) {
SeleniumFixturePageInteractions.deselectUsingLabel(locator, label);
}
/**
* Selects an option in a select box using the specified value.
*
* @param locator the \locator to use to find the element.
* @param value the value of the option within the select box.
* @throws SeleniumFixtureException when the element could not be found, is not an SELECT element, there is no option with the specified value. Ronald: DONE
*/
public void select(String locator, String value) {
SeleniumFixturePageInteractions.select(locator, value);
}
/**
* Deselects an option in a select box using the specified value.
*
* @param locator the \locator to use to find the element.
* @param value the value of the option within the select box.
* @throws SeleniumFixtureException when the element could not be found, is not an SELECT element, there is no option with the specified value or when the option was not selected in the first place.
*/
public void deselect(String locator, String value) {
SeleniumFixturePageInteractions.deselect(locator, value);
}
/**
* Selects all options in a select.
*
* @param locator the \locator to use to find the element.
* @throws SeleniumFixtureException when the element could not be found or when the element is not an SELECT element.
*/
public void selectAllOptions(String locator) {
SeleniumFixturePageInteractions.selectAllOptions(locator);
}
/**
* Deselects all options in a select box.
*
* @param locator the \locator to use to find the element.
* @throws SeleniumFixtureException when the element could not be found.
*/
public void deselectAllOptions(String locator) {
SeleniumFixturePageInteractions.deselectAllOptions(locator);
}
/**
* Returns the selected label of the select box
*
* @param locator the \locator to use to find the element.
* @return the option selected
*/
public static String getOptionSelected(String locator) {
return SeleniumFixturePageInteractions.getOptionSelected(locator);
}
/**
* Verifies if an option in a select box is selected.
*
* @param locator the \locator to use to find the element. param value the value of the option.
* @param value the value
* @return the boolean
*/
public boolean verifyOptionSelected(String locator, String value) {
return SeleniumFixtureVerifyElements.verifyOptionSelected(locator, value);
}
/**
* Verifies if all the options in a select box are selected.
*
* @param locator the \locator to use to find the element. param label the label of the option.
* @return the boolean
*/
public boolean verifyAllOptionsSelected(String locator) {
return SeleniumFixtureVerifyElements.verifyAllOptionsSelected(locator);
}
/**
* Verifies if an option in a select box is not selected.
*
* @param locator the \locator to use to find the element. param value the value of the option.
* @param value the value
* @return the boolean
*/
public boolean verifyOptionNotSelected(String locator, String value) {
return SeleniumFixtureVerifyElements.verifyOptionNotSelected(locator, value);
}
/**
* Verifies if an option in a select box is selected.
*
* @param locator the \locator to use to find the element.
* @param label the label of the option.
* @return the boolean
*/
public boolean verifyOptionSelectedByLabel(String locator, String label) {
return SeleniumFixtureVerifyElements.verifyOptionSelectedByLabel(locator, label);
}
/**
* Verifies if all the options in a select box are not selected.
*
* @param locator the \locator to use to find the element. param label the label of the option.
* @return the boolean
*/
public boolean verifyAllOptionsNotSelected(String locator) {
return SeleniumFixtureVerifyElements.verifyAllOptionsNotSelected(locator);
}
/**
* Verifies if an option in a select box is not selected.
*
* @param locator the \locator to use to find the element. param label the label of the option.
* @param label the label
* @return the boolean
*/
public boolean verifyOptionNotSelectedByLabel(String locator, String label) {
return SeleniumFixtureVerifyElements.verifyOptionNotSelectedByLabel(locator, label);
}
///@}
/** @name interaction_presence
* ## PRESENCE AND VALUE OF ELEMENTS
*
*/
///@{
/**
* Check that the element is not present
*
* @param locator the \locator to find the element.
* @return true if there are no elements present with the specified \locator.
*/
public boolean verifyElementNotPresent(String locator) {
return SeleniumFixtureVerifyElements.verifyElementNotPresent(locator);
}
/**
* Verifies if the text at the specified \locator is compliant with the given pattern.
* If the \locator refers to an input element it will check if it is a checkbox or radio button, if so it will use "true" or "false", if it
* is any other input element it will use the value of the attribute "value".
*
* @param locator the \locator to use to find the element.
* @param pattern the pattern.
* @return the boolean
*/
public boolean verifyValue(String locator, String pattern) {
return SeleniumFixtureVerifyElements.verifyValue(locator, pattern);
}
/**
* Checks whether a value is empty.
*
* @param locator the \locator
* @return the boolean
*/
public boolean verifyValueEmpty(String locator) {
return SeleniumFixtureVerifyElements.verifyValueEmpty(locator);
}
/**
* Checks whether a value is not empty.
*
* @param locator the \locator
* @return the boolean
*/
public boolean verifyValueNotEmpty(String locator) {
return SeleniumFixtureVerifyElements.verifyValueNotEmpty(locator);
}
/**
* Verifies if the text at the specified \locator is compliant with the given pattern.
* If the \locator refers to an input element it will check if it is a checkbox or radio button, if so it will use "true" or "false", if it
* is any other input element it will use the value of the attribute "value".
*
* @param locator the \locator to use to find the element.
* @param pattern the pattern. Ronald: DONE
* @return the boolean
*/
public boolean verifyText(String locator, String pattern) {
return SeleniumFixtureVerifyElements.verifyText(locator, pattern);
}
/**
* Verifies if the given text is present anywhere in the source of the page.
*
* @param text the value to verify.
* @return the boolean
*/
public boolean verifyTextPresent(String text) {
return SeleniumFixtureVerifyElements.verifyTextPresent(text);
}
/**
* Verifies if the given text is not present anywhere in the source of the page.
*
* @param text the value to verify. Ronald: DONE
* @return the boolean
*/
public boolean verifyTextNotPresent(String text) {
return SeleniumFixtureVerifyElements.verifyTextNotPresent(text);
}
/**
* Verifies that a specific String is present in a specific part of the page
*
* @param locator the \locator
* @param pattern the pattern
* @return boolean boolean
*/
public boolean verifyTextContainsString(String locator, String pattern) {
return SeleniumFixtureVerifyElements.verifyTextContainsString(locator, pattern);
}
/**
* Verify pattern present boolean.
*
* @param pattern the pattern
* @return the boolean
*/
public boolean verifyPatternPresent(String pattern) {
return SeleniumFixtureVerifyElements.verifyPatternPresent(pattern);
}
/**
* Verify attribute value boolean.
*
* @param locator the \locator
* @param attribute the attribute
* @param pattern the pattern
* @return the boolean
*/
public Boolean verifyAttributeValue(String locator, String attribute, String pattern) {
return SeleniumFixtureVerifyElements.verifyAttributeValue(locator, attribute, pattern);
}
/**
* Verify attribute present boolean.
*
* @param locator the \locator
* @param attribute the attribute
* @return the boolean
*/
public Boolean verifyAttributePresent(String locator, String attribute) {
return SeleniumFixtureVerifyElements.verifyAttributePresent(locator, attribute);
}
/**
* Checks if the element is visible
*
* @param locator the \locator
* @return the boolean
* @throws SeleniumFixtureException when the element was not visible.
*/
public boolean isElementVisible(String locator) {
return SeleniumFixtureVerifyElements.isElementVisible(locator);
}
/**
* Is element enabled boolean.
*
* @param locator the \locator
* @return the boolean
*/
public boolean isElementEnabled(String locator) {
return SeleniumFixtureVerifyElements.isElementEnabled(locator);
}
/**
* Counts the number of times an element is present on the page
*
* @param locator the \locator
* @return int with the times the element is present
*/
public int countTimesElementPresent(String locator) {
return SeleniumFixtureVerifyElements.countTimesElementPresent(locator);
}
/**
* Verifies that a specific element is x times present on the page
*
* @param locator the \locator
* @param timesPresent the times present
* @return true or false
*/
public boolean verifyTimesElementPresent(String locator, int timesPresent) {
return SeleniumFixtureVerifyElements.verifyTimesElementPresent(locator, timesPresent);
}
/**
* Checks that a specific element is x times present on the page. If the element is not x times present a SeleniumFixtureExceptionType is thrown.
*
* @param locator the \locator
* @param timesPresent the times present
* @return true or a SeleniumFixtureExceptionType with the number of times the element was found
*/
public boolean checkTimesElementPresent(String locator, int timesPresent) {
return SeleniumFixtureVerifyElements.checkTimesElementPresent(locator, timesPresent);
}
/**
* Check that the element is present
*
* @param locator the \locator
* @return true if there are elements present with the specified \locator.
*/
public boolean verifyElementPresent(String locator) {
return SeleniumFixtureVerifyElements.verifyElementPresent(locator);
}
/**
* Checks if the title of the website matches the title supplied.
*
* @param title the title of the website.
* @return true if it matches.
* @throws SeleniumFixtureException when the element can not be found or the value does not match.
*/
public boolean checkWebsiteTitle(String title) {
return SeleniumFixtureVerifyElements.checkWebsiteTitle(title);
}
///@}
/** @name interaction_get
* ## GETTING SOMETHING FROM THE PAGE
*
*/
///@{
/**
* Gets alert text.
*
* @return the alert text
*/
public String getAlertText() {
return SeleniumFixturePageInteractions.getAlertText();
}
/**
* Returns the current windowhandle
*
* @return the window handle
*/
public String getWindowHandle() {
return SeleniumFixturePageInteractions.getWindowHandle();
}
/**
* Gets current url.
*
* @return the current url
*/
public String getCurrentUrl() {
return SeleniumFixturePageInteractions.getCurrentUrl();
}
/**
* Gets title.
*
* @return the title
*/
public String getTitle() {
return SeleniumFixturePageInteractions.getTitle();
}
/**
* Returns the text that is present in the specified \locator.
*
* @param locator the \locator to use to find the element.
* @return the text
*/
public String getText(String locator) {
return SeleniumFixturePageInteractions.getText(locator);
}
/**
* Returns the value that is present in the specified \locator.
*
* @param locator the \locator to find the element
* @return the value
*/
public String getValue(String locator) {
return SeleniumFixturePageInteractions.getValue(locator);
}
/**
* Returns the value of an attribute at a specific \locator
*
* @param locator the \locator to find the element on the page.
* @param attribute the name of the attribute.
* @return the attribute
*/
public String getAttribute(String locator, String attribute) {
return SeleniumFixturePageInteractions.getAttribute(locator, attribute);
}
/**
* Returns the CSS value at a specific \locator
*
* @param locator the \locator to find the element on the page.
* @param style the css style to check, e.g. color, background-color, font-size.
* @return the css value
*/
public String getCssValue(String locator, String style) {
return SeleniumFixturePageInteractions.getCssValue(locator, style);
}
/**
* Gets page debug information.
*
* @return the page debug information
*/
public String getPageDebugInformation() {
return SeleniumFixtureUtils.getPageDebugInformation();
}
///@}
/** @name interaction_cookies
* ## WORKING WITH COOKIES
*
*/
///@{
/**
* Gets all cookies for the current website and returns them as a string
*
* @return all cookies
*/
public String getAllCookies() {
return SeleniumFixturePageInteractions.getAllCookies();
}
/**
* Deletes all cookies.
*/
public void deleteAllCookies() {
SeleniumFixturePageInteractions.deleteAllCookies();
}
/**
* Delete the cookie with the specified name.
*
* @param name the name of the cookie.
*/
public void deleteCookieByName(String name) {
SeleniumFixturePageInteractions.deleteCookieByName(name);
}
/**
* Checks if a cookie exists with the specified name
.
*
* @param name the name of the cookie.
* @return the boolean
* @throws SeleniumFixtureException When the cookie with the specified name does not exist.
*/
public boolean checkIfCookieExists(String name) {
return SeleniumFixturePageInteractions.checkIfCookieExists(name);
}
/**
* Sets a cookie for the curent domain and path with specified name and value
*
* @param name the name of the cookie.
* @param value the value of the cookie.
*/
public void setCookieForThisDomain(String name, String value) {
SeleniumFixturePageInteractions.setCookieForThisDomain(name, value);
}
/**
* Verifies the value of a cookie with the specified name
using the supplied expected value
*
* @param name the name of the cookie.
* @param value the expexted value
* @return the boolean
* @throws SeleniumFixtureException When the cookie with the specified name does not exist.
* @throws SeleniumFixtureException When the cookie with the specified name does not have the specified value.
*/
public boolean verifyValueOfCookie(String name, String value) {
return SeleniumFixturePageInteractions.verifyValueOfCookie(name, value);
}
///@}
/** @name interaction_wait
* ## WAITING FOR STUFF ON THE PAGE
*
*/
///@{
/**
* Waits for an element to become present in the dom tree.
* Will also return true if the element was already present.
*
* @param locator the \locator to use to find the element.
* @return the boolean
* @throws SeleniumFixtureException when the element was not found or the element did not appear in the dom tree.
*/
public boolean waitForElementPresent(String locator) {
return SeleniumFixtureWaits.waitForElementPresentWithFluentWait(driver, locator);
}
/**
* Returns true if the element is not present, otherwise
* Waits for an element to become detached from the dom tree.
*
* @param locator the \locator to use to find the element.
* @return the boolean
* @throws SeleniumFixtureException when the element was not found or the element did not detach itself from the dom tree.
*/
public boolean waitForElementNotPresent(String locator) {
return SeleniumFixtureWaits.waitForElementNotPresentWithFluentWait(driver, locator);
}
/**
* Returns true if the element is not present, otherwise waits the default timeout to check
* If the element has not been found in the default timeout this method continues
* Waits for an element to become detached from the dom tree.
*
* @param locator the \locator to use to find the element.
* @return the boolean
*/
public boolean waitForPossibleElementNotPresent(String locator) {
return SeleniumFixtureWaits.waitForPossibleElementNotPresent(locator);
}
/**
* Waits for an element to become visible (meaning visible and width and height != 0).
*
* @param locator the \locator to use to find the element.
* @throws SeleniumFixtureException when the element was not found or the element did not become visible.
*/
public void waitForVisible(String locator) {
SeleniumFixtureWaits.waitForVisibleWithFluentWait(locator);
}
/**
* Waits for an element to become invisible (meaning visible and width and height != 0).
*
* @param locator the \locator to use to find the element.
* @throws SeleniumFixtureException when the element was not found or the element did not become visible.
*/
public void waitForNotVisible(String locator) {
SeleniumFixtureWaits.waitForNotVisibleWithFluentWait(locator);
}
/**
* Waits for an element to become editable.
*
* @param locator the \locator to use to find the element.
* @throws SeleniumFixtureException when the element was not found or the element did not become editable.
*/
public void waitForEditable(String locator) {
SeleniumFixtureWaits.waitForEditableWithFluentWait(locator);
}
/**
* Waits for an element to become not editable.
*
* @param locator the \locator to use to find the element.
* @throws SeleniumFixtureException when the element was not found or the element did not become un-editable.
*/
public void waitForNotEditable(String locator) {
SeleniumFixtureWaits.waitForNotEditableWithFluentWait(locator);
}
/**
* Wait for element to be clickable.
*
* @param locator the \locator
*/
public void waitForElementToBeClickable(String locator) {
SeleniumFixtureWaits.waitForEditableWithFluentWait(locator);
}
/**
* Wait for alert.
*/
public void waitForAlert() {
SeleniumFixtureWaits.waitForAlertWithFluentWait(driver);
}
/**
* Wait for an alert dialog and when it appears it will accept it.
*
* @throws SeleniumFixtureException when a timeout occurs, a webdriver exception occurs or when the dialog is not appearing.
*/
public void waitForAlertAndAccept() {
SeleniumFixtureWaits.waitForAlertAndAcceptWithFluentWait(driver);
}
/**
* Wait for an alert dialog and when it appears it will accept it.
* If it doesn't appear in the wait time of 2 seconds, we continue
*
* @throws SeleniumFixtureException when a timeout occurs, a webdriver exception occurs or when the dialog is not appearing.
*/
public void waitForPossibleAlertAndAccept() {
SeleniumFixtureWaits.waitForPossibleAlertAndAcceptWithFluentWait(driver);
}
/**
* Wait for an alert dialog and when it appears it will dismiss it.
*
* @throws SeleniumFixtureException when a timeout occurs, a webdriver exception occurs or when the dialog is not appearing.
*/
public void waitForAlertAndDismiss() {
SeleniumFixtureWaits.waitForAlertAndDismissWithFluentWait(driver);
}
/**
* Wait for an alert dialog and when it appears it will dismiss it.
* If it doesn't appear in the wait time of 2 seconds, we continue
*
* @throws SeleniumFixtureException when a timeout occurs, a webdriver exception occurs or when the dialog is not appearing.
*/
public void waitForPossibleAlertAndDismiss() {
SeleniumFixtureWaits.waitForPossibleAlertAndDismissWithFluentWait(driver);
}
/**
* Waits for the text to become visible anywhere on the page.
*
* @param pattern the text to search for.
* @throws SeleniumFixtureException when the text did not become visible.
*/
public void waitForTextPresent(String pattern) {
SeleniumFixtureWaits.waitForTextPresent(pattern);
}
/**
* Waits for the text to become absent anywhere on the page.
*
* @param text the text to search for.
* @return the boolean
* @throws SeleniumFixtureException when the text did not become visible.
*/
public boolean waitForTextNotPresent(String text) {
return SeleniumFixtureWaits.waitForTextNotPresent(text);
}
/**
* Waits for the text to become visible in the specified \locator.
*
* @param locator the \locator to use to find the element.
* @param pattern the text to search for.
* @return the boolean
* @throws SeleniumFixtureException when the text did not become visible.
*/
public boolean waitForText(String locator, String pattern) {
return SeleniumFixtureWaits.waitForText(locator, pattern);
}
/**
* Waits for the specified text to be removed / adjusted.
*
* @param locator the \locator to use to find the element.
* @param pattern the text to search for.
* @return the boolean
* @throws SeleniumFixtureException when the text did not change.
*/
public boolean waitForNotText(String locator, String pattern) {
return SeleniumFixtureWaits.waitForNotText(locator, pattern);
}
/**
* Waits for the text to become absent anywhere on the page.
*
* @param locator the \locator to use to find the element.
* @param value the value to search for.
* @return the boolean
* @throws SeleniumFixtureException when the text did not become visible.
*/
public boolean waitForValue(String locator, String value) {
return SeleniumFixtureWaits.waitForValue(locator, value);
}
/**
* Waits for the element to have a value.
*
* @param locator the \locator to use to find the element.
* @return the boolean
* @throws SeleniumFixtureException when the text did not become visible.
*/
public boolean waitForValueNotEmpty(String locator) {
return SeleniumFixtureWaits.waitForValueNotEmpty(locator);
}
///@}
/** @name interaction_logging_and_util
* ## Logging information
*
*/
///@{
/**
* Log page source.
*
* @param driver the driver
*/
public void logPageSource(WebDriver driver) {
SeleniumFixtureUtils.logPageSource(driver);
}
/**
* Log this.
*
* @param input the input
*/
public void logThis(String input) {
SeleniumFixtureUtils.logThis(input);
}
/**
* Gets working dir.
*
* @return the working dir
*/
public String getWorkingDir() {
return SeleniumFixtureUtils.getWorkingDir();
}
///@}
/** @name interaction_screenshots
* ## Taking screesnhots
*
*/
///@{
/**
* Takes a screenshot using Selenium.TakesScreenshot
*
* @return a link that in the FitNesse page shows a thumbnail, the clickthroug is the complete screenshot
*/
public String takeScreenshot() {
return SeleniumFixtureScreenshot.makeScreenshotAndReturnLink(driver);
}
///@}
/** @name interaction_download
* ## Downloading a file from a page
*
*/
///@{
/**
* Sets download path.
*
* @param path the path
*/
public void setDownloadPath(String path) {
SeleniumFixtureDownloadUtil.setDownloadPath(path);
}
/**
* Sets download proxy.
*
* @param proxyHost the proxy host
* @param proxyport the proxyport
*/
public void setDownloadProxy(String proxyHost, int proxyport) {
SeleniumFixtureDownloadUtil.setDownloadProxy(proxyHost, proxyport);
}
/**
* Sets download proxy basic credentials.
*
* @param username the username
* @param password the password
*/
public void setDownloadProxyBasicCredentials(String username, String password) {
SeleniumFixtureDownloadUtil.setDownloadProxyBasicCredentials(username, password);
}
/**
* Sets download proxy ntlm credentials.
*
* @param username the username
* @param password the password
* @param workstation the workstation
* @param domain the domain
*/
public void setDownloadProxyNtlmCredentials(String username, String password, String workstation, String domain) {
SeleniumFixtureDownloadUtil.setDownloadProxyNtlmCredentials(username, password, workstation, domain);
}
/** @name interaction_download
* ## Downloading a file from a page
*
*/
///@{
/**
* Download a file from a \locator using the selenium session (cookies, login state, etc..) and save it in the java TEMP folder using the specified name.
* This method resolves the 'href' attribute of the locator and downloads the file
*
* @param locator the \locator
* @param filename the filename
* @return full path to the file
* @throws Exception the exception
*/
public String downloadFile(String locator, String filename) throws Exception {
return SeleniumFixtureDownloadUtil.downloadFile(locator, filename);
}
/**
* Download a file from a URL using the selenium session (cookies, login state, etc..) and save it in the java TEMP folder using the specified name.
*
* @param url the complete url of the file to be downloades
* @param filename the name of the file that will be written to disk
* @return full path to the file
*/
public String downloadUrl(String url, String filename) {
return SeleniumFixtureDownloadUtil.downloadUrl(url, filename);
}
/**
* Download an image from a \locator using the selenium session (cookies, login state, etc..) and save it in the java TEMP folder using the specified name.
* This method resolves the 'src' attribute of the locator and downloads the image
*
* @param locator the \locator
* @param filename the filename
* @return full path to the image
* @throws Exception the exception
*/
public String downloadImage(String locator, String filename) throws Exception {
return SeleniumFixtureDownloadUtil.downloadImage(locator, filename);
}
///@}
/** @name interaction_conditional
* ## LOOPING AND CONDITIONAL EXECUTION OF COMMANDS
*
*/
///@{
/**
* Sets loop command.
*
* @param command the command
*/
public void setLoopCommand(String command) {
loopCommands.add(new WebdriverCommand(command));
}
/**
* Sets loop command.
*
* @param command the command
* @param target the target
*/
public void setLoopCommand(String command, String target) {
loopCommands.add(new WebdriverCommand(command, target));
}
/**
* Sets loop command.
*
* @param command the command
* @param target the target
* @param value the value
*/
public void setLoopCommand(String command, String target, String value) {
loopCommands.add(new WebdriverCommand(command, target, value));
}
/**
* Sets loop command.
*
* @param command the command
* @param target the target
* @param value the value
* @param param the param
*/
public void setLoopCommand(String command, String target, String value, String param) {
loopCommands.add(new WebdriverCommand(command, target, value, param));
}
/**
* Reset loop commands.
*/
public void resetLoopCommands() {
loopCommands.clear();
}
/**
* Run loop.
*
* @param numberOfTimes the number of times
* @param timeBetweenLoopsInMillis the time between loops in millis
*/
public void runLoop(int numberOfTimes, int timeBetweenLoopsInMillis) {
for (int x = 0; x < numberOfTimes; x++) {
for (WebdriverCommand command : loopCommands) {
command.execute();
}
try {
Thread.sleep(timeBetweenLoopsInMillis);
} catch (InterruptedException e) {
//do nothing
}
}
}
/**
* Run loop command if element is present.
*
* @param locator the \locator
*/
public void runLoopCommandIfElementIsPresent(String locator) {
if (verifyElementPresent(locator)) {
for (WebdriverCommand command : loopCommands) {
command.execute();
}
}
}
/**
* Run loop until element present boolean.
*
* @param locator the \locator
* @param maxTimesToLoop the max times to loop
* @param timeBetweenLoopsInMillis the time between loops in millis
* @return the boolean
*/
public boolean runLoopUntilElementPresent(String locator, int maxTimesToLoop, int timeBetweenLoopsInMillis) {
int current = 0;
while (current < maxTimesToLoop && !verifyElementPresent(locator)) {
for (WebdriverCommand command : loopCommands) {
command.execute();
}
try {
Thread.sleep(timeBetweenLoopsInMillis);
} catch (InterruptedException e) {
//do nothing
}
current++;
}
return verifyElementPresent(locator);
}
/**
* Run loop until javascript return value boolean.
*
* @param javascript the javascript
* @param expectedExpressionReturnValue the expected expression return value
* @param maxTimesToLoop the max times to loop
* @param timeBetweenLoopsInMillis the time between loops in millis
* @return the boolean
*/
public boolean runLoopUntilJavascriptReturnValue(String javascript, String expectedExpressionReturnValue, int maxTimesToLoop, int timeBetweenLoopsInMillis) {
int current = 0;
while (current < maxTimesToLoop && !((JavascriptExecutor) driver).executeScript(javascript).equals(expectedExpressionReturnValue)) {
for (WebdriverCommand command : loopCommands) {
command.execute();
}
try {
Thread.sleep(timeBetweenLoopsInMillis);
} catch (InterruptedException e) {
//do nothing
}
current++;
}
return ((JavascriptExecutor) driver).executeScript(javascript).equals(expectedExpressionReturnValue);
}
///@}
/// @cond SKIP
/**
* The type Webdriver command.
*/
protected class WebdriverCommand {
private String command;
private String target;
private String value;
private String param;
/**
* Instantiates a new Webdriver command.
*
* @param command the command
* @param target the target
* @param value the value
* @param param the param
*/
protected WebdriverCommand(String command, String target, String value, String param) {
this(command, target, value);
this.param = param;
}
/**
* Instantiates a new Webdriver command.
*
* @param command the command
* @param target the target
* @param value the value
*/
protected WebdriverCommand(String command, String target, String value) {
this(command, target);
this.value = value;
}
/**
* Instantiates a new Webdriver command.
*
* @param command the command
*/
protected WebdriverCommand(String command) {
this.command = command.replaceAll(";", "");
}
/**
* Instantiates a new Webdriver command.
*
* @param command the command
* @param target the target
*/
protected WebdriverCommand(String command, String target) {
this(command);
this.target = target;
}
/**
* Execute.
*/
protected void execute() {
try {
if (target == null && value == null) {
try {
Method method = SeleniumFixture.this.getClass().getMethod(command);
method.invoke(SeleniumFixture.this);
} catch (NoSuchMethodException e) {
throw new SeleniumFixtureException(e.getMessage());
}
} else if (target != null && value == null) {
try {
Method method = SeleniumFixture.this.getClass().getMethod(command, String.class);
method.invoke(SeleniumFixture.this, target);
} catch (NoSuchMethodException e) {
throw new SeleniumFixtureException(e.getMessage());
}
} else if (target != null && value != null && param == null) {
try {
Method method = SeleniumFixture.this.getClass().getMethod(command, String.class, String.class);
method.invoke(SeleniumFixture.this, target, value);
} catch (NoSuchMethodException e) {
throw new SeleniumFixtureException(e.getMessage());
}
} else {
try {
Method method = SeleniumFixture.this.getClass().getMethod(command, String.class, String.class, String.class);
method.invoke(SeleniumFixture.this, target, value, param);
} catch (NoSuchMethodException e) {
throw new SeleniumFixtureException(e.getMessage());
}
}
} catch (IllegalAccessException | InvocationTargetException e) {
throw new SeleniumFixtureException(e.getMessage());
}
}
}
/// @endcond SKIP
}