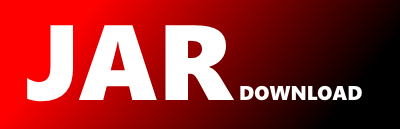
nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon Maven / Gradle / Ivy
The newest version!
package nl.psek.fitnesse.fixtures.selenium;
import nl.psek.fitnesse.ConditionalException;
import org.openqa.selenium.*;
import org.openqa.selenium.support.ui.Select;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
/**
* Common methods used by the classes in this package
*/
public class SeleniumFixtureCommon {
public final static String ELEMENT_INPUT = "input";
public final static String ELEMENT_TEXTAREA = "textarea";
public final static String ATTR_TYPE = "type";
public final static String TYPE_CHECKBOX = "checkbox";
public final static String TYPE_RADIO = "radio";
public final static String ATTR_VALUE = "value";
protected static final Logger LOG = LoggerFactory.getLogger(SeleniumFixtureCommon.class);
public static WebDriver driver;
protected static String SCREENSHOTLOCATION;
protected static boolean SYSTEMISMACOS = false;
protected static Keys CtrlOrCmd = Keys.CONTROL;
private static boolean circumventSelenium3 = false;
static String getTimeStamp() {
DateFormat dateFormat = new SimpleDateFormat("yyyyMMddHHmmssS");
Date date = new Date();
return (dateFormat.format(date));
}
static WebElement findElement(String locator) {
try {
return driver.findElement(constructLocatorFromString(locator));
} catch (NoSuchElementException e) {
throw new SeleniumFixtureException("No element found using the locator '%s'.", locator);
}
}
static List findElements(String locator) {
try {
List elements = driver.findElements(constructLocatorFromString(locator));
if (elements.size() > 0) {
return elements;
} else throw new ConditionalException("No element found using the locator '%s'.", locator);
} catch (NoSuchElementException e) {
throw new ConditionalException("No element found using the locator '%s'.", locator);
}
}
static Select findSelect(String locator) {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase("select")) {
return new Select(element);
}
throw new ConditionalException("Element found using the locator '%s' is not a select element but a '%s' element.", locator, tagName);
}
static WebElement findOptionInSelectByValue(Select select, String value) {
for (WebElement option : select.getOptions()) {
if (option.getAttribute("value").equals(value)) {
return option;
}
}
throw new ConditionalException("The select element does not have an option with value '%s'", value);
}
static WebElement findOptionInSelectByLabel(Select select, String label) {
for (WebElement option : select.getOptions()) {
if (option.getText().equals(label)) {
return option;
}
}
throw new ConditionalException("The select element does not have an option with label '%s'", label);
}
public static By constructLocatorFromString(String locator) {
LOG.debug("Looking for element: " + locator);
if (locator.startsWith("xpath=")) {
return By.xpath(locator.substring("xpath=".length()));
} else if (locator.startsWith("css=")) {
return By.cssSelector(locator.substring("css=".length()));
} else if (locator.startsWith("id=")) {
return By.id(locator.substring("id=".length()));
} else if (locator.startsWith("name=")) {
return By.name(locator.substring("name=".length()));
} else if (locator.startsWith("classname=")) {
return By.className(locator.substring("classname=".length()));
} else if (locator.startsWith("link=")) {
return By.linkText(locator.substring("link=".length()));
} else {
return locator.startsWith("partiallink=") ? By.partialLinkText(locator.substring("partiallink=".length())) : By.xpath(locator);
}
}
/**
* Get the actual value of an element, for checkboxes and radio buttons it will return true
or false
.
*
* @param element the element
* @return the value or null.
*/
static String getValueElement(WebElement element) {
String value = null;
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT)) {
String type = element.getAttribute(ATTR_TYPE);
if (type.equalsIgnoreCase(TYPE_CHECKBOX) || type.equalsIgnoreCase(TYPE_RADIO)) {
value = element.isSelected() ? Boolean.TRUE.toString() : Boolean.FALSE.toString();
} else {
value = element.getAttribute(ATTR_VALUE);
}
} else if (tagName.equalsIgnoreCase(ELEMENT_TEXTAREA)) {
return element.getAttribute(ATTR_VALUE);
} else {
throw new ConditionalException("Element is not an INPUT element, can only get value of elements of type INPUT.");
}
return value;
}
static void circumventSelenium3LimitationsUsingJavascriptToPerformActionIfElementNotAccessible() {
LOG.info("One some methods Selenium3 limitations will be overridden (i.e. overlay on element you want to click)");
circumventSelenium3 = true;
}
static void restoreSelenium3LimitationsDontUseJavascriptLetSeleniumDoItsTheDefaultThing() {
LOG.info("Selenium3 default behaviour restored");
circumventSelenium3 = false;
}
static boolean isCircumventSelenium3() {
return circumventSelenium3;
}
static void quitDriverIfRequired() {
if (Boolean.parseBoolean(System.getProperty("FSTOPONEXCEPTION"))) {
driver.quit();
}
}
static boolean notNullOrEmpty(Object input) {
if (input instanceof String) {
return ((input != null) && !((String) input).isEmpty());
} else if (input instanceof List) {
return (!((List) input).isEmpty());
} else {
return (input != null);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy