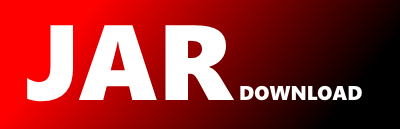
nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureDownloadUtil Maven / Gradle / Ivy
The newest version!
package nl.psek.fitnesse.fixtures.selenium;
import org.apache.http.HttpEntity;
import org.apache.http.HttpHost;
import org.apache.http.HttpResponse;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.NTCredentials;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.CookieStore;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.*;
import org.apache.http.impl.cookie.BasicClientCookie;
import org.openqa.selenium.Cookie;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Set;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.constructLocatorFromString;
/**
* A util to download a file from the current page using the current WebDriver session for authentication
*/
public class SeleniumFixtureDownloadUtil {
private static final Logger LOG = LoggerFactory.getLogger(SeleniumFixtureDownloadUtil.class);
static CredentialsProvider credsProviderBasic;
static CredentialsProvider credsProviderNtlm;
static String downloadProxyHost;
static int downloadProxyPort;
static String downloadProxyUsernameBasic;
static String downloadProxyPasswordBasic;
static String downloadProxyUsernameNtlm;
static String downloadProxyPasswordNtlm;
static String downloadProxyWorstationNtlm;
static String downloadProxyDomainNtlm;
private static HttpHost proxy;
static int statuscode;
static HttpResponse httpResponse;
static String localDownloadPath = System.getProperty("java.io.tmpdir");
private WebDriver driver;
private static boolean mimicWebDriverCookieState = false;
private static final String ERROR_GETTING_FILE_CONTENT = "Error getting file content for HttpEntity: ";
private static final String ERROR_NO_HTTP_ENTITY_IN_RESPONSE = "No http entity in the response";
public SeleniumFixtureDownloadUtil(WebDriver driverObject) {
this.driver = driverObject;
mimicWebDriverCookieState = true;
}
public SeleniumFixtureDownloadUtil() {
}
static void setDownloadPath(String path) {
setLocalDownloadPath(path);
}
static void setDownloadProxy(String proxyHost, int proxyport) {
downloadProxyHost = proxyHost;
downloadProxyPort = proxyport;
}
static void setDownloadProxyBasicCredentials(String username, String password) {
downloadProxyUsernameBasic = username;
downloadProxyPasswordBasic = password;
}
static void setDownloadProxyNtlmCredentials(String username, String password, String workstation, String domain) {
downloadProxyUsernameNtlm = username;
downloadProxyPasswordNtlm = password;
downloadProxyWorstationNtlm = workstation;
downloadProxyDomainNtlm = domain;
}
static String downloadFile(String locator, String filename) throws Exception {
SeleniumFixtureDownloadUtil seleniumFixtureDownloadUtil = new SeleniumFixtureDownloadUtil(nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.driver);
return downloadFileCommand(locator, filename);
}
static String downloadUrl(String url, String filename) {
SeleniumFixtureDownloadUtil seleniumFixtureDownloadUtil = new SeleniumFixtureDownloadUtil(nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.driver);
return downloadUrlCommand(url, filename);
}
static String downloadImage(String locator, String filename) throws Exception {
SeleniumFixtureDownloadUtil seleniumFixtureDownloadUtil = new SeleniumFixtureDownloadUtil(nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.driver);
return downloadFileCommand(locator, filename);
}
private static void setProxy() {
if (downloadProxyHost != null && downloadProxyPort > 0) {
proxy = new HttpHost(downloadProxyHost, downloadProxyPort);
LOG.info("Set proxy to: " + downloadProxyHost + ":" + downloadProxyPort);
} else {
LOG.info("Not using a proxy to download");
}
}
private static void setBasicProxyCredentials() {
credsProviderBasic = null; //reset credsProvider, can set only one
if (downloadProxyUsernameBasic != null && downloadProxyPasswordBasic != null) {
credsProviderBasic = new BasicCredentialsProvider();
credsProviderBasic.setCredentials(
new AuthScope(downloadProxyHost, downloadProxyPort),
new UsernamePasswordCredentials(downloadProxyUsernameBasic, downloadProxyPasswordBasic));
LOG.info("Set basicproxy credentials to: " + downloadProxyUsernameBasic + " : ***** (password hidden)");
} else {
LOG.info("Not using basic proxy credentials to download");
}
}
private static void setNtlmProxyCredentials() {
credsProviderNtlm = null; //reset credsProvider, can set only one
if (downloadProxyUsernameNtlm != null && downloadProxyPasswordNtlm != null) {
credsProviderNtlm = new BasicCredentialsProvider();
credsProviderNtlm.setCredentials(
new AuthScope(downloadProxyHost, downloadProxyPort, AuthScope.ANY_REALM, "ntlm"),
new NTCredentials(downloadProxyUsernameNtlm, downloadProxyPasswordNtlm, downloadProxyWorstationNtlm, downloadProxyDomainNtlm));
LOG.info("Set NTLM proxy credentials to: " + downloadProxyUsernameNtlm + " : ***** (password hidden)");
} else {
LOG.info("Not using NTLM proxy credentials to download");
}
}
private static void setLocalDownloadPath(String localDownloadPath) {
SeleniumFixtureDownloadUtil.localDownloadPath = localDownloadPath;
}
private static String downloadFileCommand(String locator, String targetFilename) {
String url = getUrlFromLocator(locator, "href");
return downloadTheFile(url, targetFilename);
}
private static String downloadUrlCommand(String url, String targetFilename) {
return downloadTheFile(url, targetFilename);
}
private String downloadImageCommand(String locator, String targetFilename) {
String url = getUrlFromLocator(locator, "src");
return downloadTheFile(url, targetFilename);
}
private static String getUrlFromLocator(String locator, String attribute) {
WebElement element = findElement(locator);
String fileToDownloadSourceLocation = element.getAttribute(attribute);
LOG.info("Source file for this download: " + fileToDownloadSourceLocation);
return fileToDownloadSourceLocation;
}
private static HttpResponse executeGetRequest(String url) {
HttpGet httpget = new HttpGet(url);
try {
httpResponse = createHttpClient().execute(httpget);
statuscode = httpResponse.getStatusLine().getStatusCode();
} catch (IOException e) {
LOG.info(e.getMessage());
}
return httpResponse;
}
private static String downloadTheFile(String url, String targetFilename) {
Path downloadedFile = Paths.get(localDownloadPath + targetFilename);
LOG.info("Downloaded file will be saved as: " + downloadedFile);
executeGetRequest(url);
if (statuscode == 200) {
HttpEntity entity = httpResponse.getEntity();
if (entity != null) {
InputStream instream = null;
try {
instream = entity.getContent();
} catch (IOException e) {
LOG.info(ERROR_GETTING_FILE_CONTENT + url);
throw new SeleniumFixtureException(ERROR_GETTING_FILE_CONTENT + url);
}
try {
Files.copy(instream, downloadedFile);
return downloadedFile.toString();
} catch (IOException e) {
LOG.info(e.getMessage());
throw new SeleniumFixtureException("Error copying inputstream to file " + e.getMessage());
}
} else {
LOG.info(ERROR_NO_HTTP_ENTITY_IN_RESPONSE);
throw new SeleniumFixtureException(ERROR_NO_HTTP_ENTITY_IN_RESPONSE);
}
} else throw new SeleniumFixtureException("Http statuscode was not 200 but " + statuscode);
}
public InputStream download(URL url) throws IOException {
executeGetRequest(url.toString());
if (statuscode == 200) {
HttpEntity entity = httpResponse.getEntity();
if (entity != null) {
InputStream instream = null;
try {
instream = entity.getContent();
return instream;
} catch (IOException e) {
LOG.info(ERROR_GETTING_FILE_CONTENT + url);
throw new SeleniumFixtureException(ERROR_GETTING_FILE_CONTENT + url + e.getMessage());
}
} else {
LOG.info(ERROR_NO_HTTP_ENTITY_IN_RESPONSE);
throw new SeleniumFixtureException(ERROR_NO_HTTP_ENTITY_IN_RESPONSE);
}
} else throw new SeleniumFixtureException("Http statuscode was not 200 but " + statuscode);
}
private static CloseableHttpClient createHttpClient() {
// create the builders
HttpClientBuilder httpClientBuilder = HttpClients.custom();
// add proxy credentials
setBasicProxyCredentials();
setNtlmProxyCredentials();
if (credsProviderBasic != null) {
httpClientBuilder.setDefaultCredentialsProvider(credsProviderBasic);
} else if (credsProviderNtlm != null) {
httpClientBuilder.setDefaultCredentialsProvider(credsProviderNtlm);
}
httpClientBuilder.setDefaultRequestConfig(createRequestConfig());
// copy Selenium cookiestore if required
if (mimicWebDriverCookieState) {
httpClientBuilder.setDefaultCookieStore(copySeleniumCookiesToDownloaderCookies());
}
// build the CloseableHttpClient
return httpClientBuilder.build();
}
private static RequestConfig createRequestConfig() {
RequestConfig.Builder config = RequestConfig.custom();
// building the request
// add proxy
setProxy();
if (proxy != null) {
config.setProxy(proxy);
LOG.info("Setting proxy to: " + proxy.toURI());
}
// build the config
return config.build();
}
private static CookieStore copySeleniumCookiesToDownloaderCookies() {
return mimicCookieState(nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.driver.manage().getCookies());
}
private static BasicCookieStore mimicCookieState(Set seleniumCookieSet) {
BasicCookieStore mimicWebDriverCookieStore = new BasicCookieStore();
for (Cookie seleniumCookie : seleniumCookieSet) {
BasicClientCookie duplicateCookie = new BasicClientCookie(seleniumCookie.getName(), seleniumCookie.getValue());
duplicateCookie.setDomain(seleniumCookie.getDomain());
duplicateCookie.setSecure(seleniumCookie.isSecure());
duplicateCookie.setExpiryDate(seleniumCookie.getExpiry());
duplicateCookie.setPath(seleniumCookie.getPath());
mimicWebDriverCookieStore.addCookie(duplicateCookie);
}
LOG.debug("Cookies copied from Selenium Webdriver: " + mimicWebDriverCookieStore.getCookies().toString());
return mimicWebDriverCookieStore;
}
private static WebElement findElement(String locator) {
try {
return nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.driver.findElement(constructLocatorFromString(locator));
} catch (NoSuchElementException e) {
throw new SeleniumFixtureException("No element found using the locator '%s'.", locator);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy