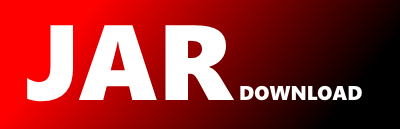
nl.psek.fitnesse.fixtures.selenium.SeleniumFixturePageInteractions Maven / Gradle / Ivy
The newest version!
package nl.psek.fitnesse.fixtures.selenium;
import nl.psek.fitnesse.ConditionalException;
import org.openqa.selenium.*;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.remote.LocalFileDetector;
import org.openqa.selenium.remote.RemoteWebElement;
import org.openqa.selenium.support.ui.Select;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.regex.Pattern;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.*;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureUtils.getWorkingDir;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureVerifyElements.*;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureWaits.waitForElementPresentWithFluentWait;
/**
* Methods that interact with the currently loaded webpage
*/
public class SeleniumFixturePageInteractions {
public final static String ELEMENT_INPUT = "input";
public final static String ELEMENT_TEXTAREA = "textarea";
private static final String REGEXPI_SYNTAX = "regexpi:";
private static final String REGEX_SYNTAX = "regex:";
static void openWebsite(String url) {
try {
new URL(url);
} catch (MalformedURLException e) {
throw new SeleniumFixtureException("The specified URL '%s' is not a valid URL.", url);
}
driver.get(url);
}
static void refreshWebsite() {
driver.navigate().refresh();
}
static void closeWindow() {
driver.close();
}
static void closeWebsite() {
driver.quit();
}
static void maximizeWindow() {
driver.manage().window().maximize();
}
static void browserBack() {
driver.navigate().back();
}
static void browserForward() {
driver.navigate().forward();
}
static void click(WebDriver driver, String locator) {
try {
findElement(locator).click();
} catch (ElementNotVisibleException e) {
scrollTo(locator);
findElement(locator).click();
} catch (ElementNotInteractableException e) {
if (isCircumventSelenium3()) {
LOG.info("We went into circumventSelenium3Mode and clicked using JavaScript on locator: " + locator + ". An ElementNotInteractableException was caught.");
clickUsingJavascript(driver, locator);
} else {
throw new SeleniumFixtureException((e.getMessage()));
}
}
}
static void clickUsingAction(String locator) {
WebElement element = findElement(locator);
Actions action = new Actions(driver);
action.moveToElement(element).click().perform();
}
static void contextClick(String locator) {
WebElement element = findElement(locator);
Actions action = new Actions(driver);
action.contextClick(element);
action.perform();
}
static void doubleClick(String locator) {
WebElement element = findElement(locator);
Actions action = new Actions(driver);
action.doubleClick(element);
action.perform();
}
static void clickUsingJavascript(WebDriver driver, String locator) {
WebElement element = findElement(locator);
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("arguments[0].click();", element);
}
static void clickUpToTimes(String locator, String numberOfTimesToExecute) {
int totalNumberOfTimes = Integer.parseInt(numberOfTimesToExecute);
for (int times = 1; times <= totalNumberOfTimes; times++) {
findElement(locator).click();
}
}
static void clickUntilElementPresent(String elementToClick, String elementToWaitFor, long commandTimeout, int maxAttempts) {
boolean isPresent = findElements((elementToWaitFor)).size() > 0; //controleer of het element er al is, return true
driver.manage().timeouts().implicitlyWait(commandTimeout, TimeUnit.SECONDS); // zet de command timeout op de waarde die is meegegeven
int currentAttempt = 0;
while (!isPresent && currentAttempt < maxAttempts) { // als het er niet is doen we dit
findElement(elementToClick).click(); // klik op een element
isPresent = findElements((elementToWaitFor)).size() > 0; //controleer of het element er al is, return true
currentAttempt++;
}
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // zet de command timeout weer op de default waarde
if (currentAttempt >= maxAttempts) {
LOG.info("The max number of attempts has been reached");
}
LOG.info("It took approximately: " + ((commandTimeout) * currentAttempt) + " seconds.");
}
static void clickIfOtherElementPresent(String elementToClick, String elementToWaitFor) {
boolean isPresent = false;
try {
isPresent = findElements((elementToWaitFor)).size() > 0;
} catch (Exception e) {
//not present go on
}
if (isPresent) {
click(driver, elementToClick);
} else try {
isPresent = waitForElementPresentWithFluentWait(driver, elementToWaitFor);
if (!isPresent) {
//Als het element niet gevonden wordt na het wachten hoeft er ook niet geklikt te worden
} else
click(driver, elementToClick); // als het element wel aanwezig is na het wachten: voer een click actie uit}
} catch (TimeoutException timeoutException) { // In het geval van een TimeoutException geven niets terug, het element is niet gevonden in de gestelde tijd
}
}
static void clickIfOtherElementNotPresent(String elementToClick, String elementThatShoudBeAbsent) {
if (verifyElementNotPresent(elementThatShoudBeAbsent)) {
click(driver, elementToClick);
}
}
static void type(String locator, String value) {
if (SYSTEMISMACOS) {
typeWithClear(locator, value);
return;
} else {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT) || tagName.equalsIgnoreCase(ELEMENT_TEXTAREA)) {
element.sendKeys(Keys.chord(CtrlOrCmd, "a"), value);
return;
}
throw new SeleniumFixtureException("Element found using the locator '%s' is not an input or textarea element but a '%s' element.", locator, tagName);
}
}
static void typeWithClear(String locator, String value) {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT) || tagName.equalsIgnoreCase(ELEMENT_TEXTAREA)) {
element.clear();
element.sendKeys(value);
return;
}
throw new SeleniumFixtureException("Element found using the locator '%s' is not an input or textarea element but a '%s' element.", locator, tagName);
}
static void typeUsingJavascript(String locator, String value) {
WebElement element = findElement(locator);
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("arguments[0].setAttribute(\"value\",\"" + value + "\");", element);
}
static void typeIfElementPresent(String locator, String value) {
Boolean valueBefore = Boolean.getBoolean(System.getProperty("FSTOPONEXCEPTION"));
System.setProperty("FSTOPONEXCEPTION", "false");
try {
if (isElementVisible(locator) && isElementEnabled(locator)) {
type(locator, value);
LOG.info("Element " + locator + " was visible, value " + value + " was entered");
}
} catch (Exception ignored) {
LOG.info("Element " + locator + " was not visible or present, no action was taken");
}
System.setProperty("FSTOPONEXCEPTION", valueBefore.toString());
}
static void typeWithoutClear(String locator, String value) {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT) || tagName.equalsIgnoreCase(ELEMENT_TEXTAREA)) {
element.sendKeys(value);
return;
}
throw new ConditionalException("Element found using the locator '%s' is not an input or textarea element but a '%s' element.", locator, tagName);
}
static void typeKeyCodeWithoutClear(String locator, Keys keycode) {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT) || tagName.equalsIgnoreCase(ELEMENT_TEXTAREA)) {
element.sendKeys(keycode);
return;
}
throw new ConditionalException("Element found using the locator '%s' is not an input or textarea element but a '%s' element.", locator, tagName);
}
static void typeKeyCodeUsingActions(Keys keycode) {
Actions action = new Actions(driver);
action.sendKeys(keycode).build().perform();
}
static void tab(String locator) {
WebElement element = findElement(locator);
element.sendKeys(Keys.TAB);
}
static String getText(String locator) {
WebElement element = findElement(locator);
return element.getText();
}
static String getValue(String locator) {
WebElement element = findElement(locator);
return getValueElement(element);
}
static String getAttribute(String locator, String attribute) {
WebElement element = findElement(locator);
return element.getAttribute(attribute);
}
static String getCssValue(String locator, String style) {
WebElement element = findElement(locator);
return element.getCssValue(style);
}
static String getOptionSelected(String locator) {
Select select = findSelect(locator);
WebElement option = select.getFirstSelectedOption();
return option.getText();
}
static void select(String locator, String value) {
Select select = findSelect(locator);
WebElement option = findOptionInSelectByValue(select, value);
if (option.isSelected()) {
throw new SeleniumFixtureException("The option with value '%s' cannot be selected since the option was already selected.", value);
}
select.selectByValue(value);
}
static void deselect(String locator, String value) {
Select select = findSelect(locator);
WebElement option = findOptionInSelectByValue(select, value);
if (!option.isSelected()) {
throw new SeleniumFixtureException("The option with value '%s' cannot be deselected since the option was not selected.", value);
}
select.deselectByValue(value);
}
static void selectAllOptions(String locator) {
Select select = findSelect(locator);
for (WebElement option : select.getOptions()) {
if (!option.isSelected()) {
select.selectByVisibleText(option.getText());
}
}
}
static void deselectAllOptions(String locator) {
Select select = findSelect(locator);
select.deselectAll();
}
static void selectUsingLabel(String locator, String label) {
Select select = findSelect(locator);
WebElement option = findOptionInSelectByLabel(select, label);
if (option.isSelected()) {
quitDriverIfRequired();
throw new SeleniumFixtureException("The option with label '%s' cannot be selected since the option was already selected. Add 'allowDefault' to prevent this exception.", label);
}
select.selectByVisibleText(label);
}
static void selectUsingLabel(String locator, String label, String keywordAllow) {
Boolean allowDefault = false;
if (keywordAllow.equalsIgnoreCase("allowdefault")) {
allowDefault = true;
}
Select select = findSelect(locator);
WebElement option = findOptionInSelectByLabel(select, label);
if (!allowDefault && option.isSelected()) {
throw new SeleniumFixtureException("The option with label '%s' cannot be selected since the option was already selected and the keyword 'allowDefault' was not added to the command. Instead '%s' was added to the command.", label, keywordAllow);
}
select.selectByVisibleText(label);
}
static void selectUsingLabelIfElementPresent(String locator, String label) {
Boolean valueBefore = Boolean.valueOf(System.getProperty("FSTOPONEXCEPTION"));
System.setProperty("FSTOPONEXCEPTION", "false");
try {
Select select = findSelect(locator);
WebElement option = findOptionInSelectByLabel(select, label);
if (select != null && option != null) {
select.selectByVisibleText(label);
}
} catch (Exception ignored) {
}
System.setProperty("FSTOPONEXCEPTION", String.valueOf(valueBefore));
}
static void deselectUsingLabel(String locator, String label) {
Select select = findSelect(locator);
WebElement option = findOptionInSelectByLabel(select, label);
if (!option.isSelected()) {
throw new SeleniumFixtureException("The option with label '%s' cannot be deselected since the option was not selected.", label);
}
select.deselectByVisibleText(label);
}
static void makeChecked(String locator) {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT)) {
String type = element.getAttribute(ATTR_TYPE);
if (type.equalsIgnoreCase(TYPE_CHECKBOX) || type.equalsIgnoreCase(TYPE_RADIO)) {
if (!element.isSelected()) {
element.click();
}
} else {
throw new SeleniumFixtureException("Element found using the locator '%s' is not a checkbox or a radio button, makeChecked only works on a checkbox or radio button.");
}
} else {
throw new SeleniumFixtureException("Element found using the locator '%s' is not an input element but a '%s' element.", locator, tagName);
}
}
static void makeNotChecked(String locator) {
WebElement element = findElement(locator);
String tagName = element.getTagName();
if (tagName.equalsIgnoreCase(ELEMENT_INPUT)) {
String type = element.getAttribute(ATTR_TYPE);
if (type.equalsIgnoreCase(TYPE_CHECKBOX) || type.equalsIgnoreCase(TYPE_RADIO)) {
if (element.isSelected()) {
element.click();
}
} else {
throw new SeleniumFixtureException("Element found using the locator '%s' is not a checkbox, makeNotChecked only works on a checkbox.");
}
} else {
throw new SeleniumFixtureException("Element found using the locator '%s' is not an input element but a '%s' element.", locator, tagName);
}
}
static String getAlertText() {
String mainWindow = driver.getWindowHandle();
String alertText = driver.switchTo().alert().getText();
driver.switchTo().window(mainWindow);
return alertText;
}
static void acceptAlert() {
driver.switchTo().alert().accept();
}
static void dismissAlert() {
driver.switchTo().alert().dismiss();
}
static String getCurrentUrl() {
return driver.getCurrentUrl();
}
static String getTitle() {
return driver.getTitle();
}
static String getAllCookies() {
return driver.manage().getCookies().toString();
}
static void deleteAllCookies() {
driver.manage().deleteAllCookies();
LOG.info("Deleted all cookies");
}
static void deleteCookieByName(String name) {
driver.manage().deleteCookieNamed(name);
LOG.info("Deleted cookie with name: " + name);
}
static boolean checkIfCookieExists(String name) {
boolean found = false;
for (Cookie cookie : driver.manage().getCookies()) {
if (cookie.getName().equalsIgnoreCase(name)) {
found = true;
}
}
if (found) {
return true;
}
throw new SeleniumFixtureException("Cookie with name '%s' does not exist.", name);
}
static boolean verifyValueOfCookie(String name, String value) {
for (Cookie cookie : driver.manage().getCookies()) {
if (cookie.getName().equalsIgnoreCase(name)) {
if (cookie.getValue().equals(value)) {
return true;
} else {
throw new SeleniumFixtureException("The value of the cookie with the name '%s' is '%s', but we expected '%s'.", name, cookie.getValue(), value);
}
}
}
throw new SeleniumFixtureException("Cookie with name '%s' does not exist.", name);
}
static void setCookieForThisDomain(String name, String value) {
Cookie cookie = new Cookie(name, value);
driver.manage().addCookie(cookie);
LOG.info("Set cookie with name: " + name + " to value: " + value);
}
static void resizeWindow(int pixelsWidth, int pixelsHeight) {
Dimension dimension = new Dimension(pixelsWidth, pixelsHeight);
driver.manage().window().setSize(dimension);
}
static void moveWindow(int horizontal, int vertical) {
//get position
int x = driver.manage().window().getPosition().getX();
int y = driver.manage().window().getPosition().getY();
int xPos = x + horizontal;
int yPos = y + vertical;
//set new position
driver.manage().window().setPosition(new Point(xPos, yPos));
}
static void setWindowPosition(int x, int y) {
driver.manage().window().setPosition(new Point(x, y));
}
static void switchToFrameUsingLocator(String locator) {
WebDriver drv = driver.switchTo().frame(findElement(locator));
if (drv == null) {
throw new SeleniumFixtureException("No frame found matching the locator '%s'.", locator);
}
driver = drv;
}
static void switchToFrameUsingIndex(int index) {
WebDriver drv = driver.switchTo().frame(index);
if (drv == null) {
throw new SeleniumFixtureException("No frame found matching the index '%s'.", index);
}
driver = drv;
}
static void switchToStart() {
driver = driver.switchTo().defaultContent();
}
static void switchToWindow(String nameOrHandle) {
WebDriver drv = driver.switchTo().window(nameOrHandle);
if (drv == null) {
throw new SeleniumFixtureException("No window found where the name or handle corresponds with the value '%s'", nameOrHandle);
}
driver = drv;
}
static void switchToWindowByTitle(String pattern) {
// Store the current window handle- the parent one
String winHandleBefore = driver.getWindowHandle();
boolean foundCorrectWindow = false;
// store all the windows
Set handle = driver.getWindowHandles();
LOG.info("Set of found handles: " + handle.toString());
// iterate over window handles
for (String mywindows : handle) {
// store window title
String windowTitleForHandle = driver.switchTo().window(mywindows).getTitle();
LOG.info("Now trying windows with windowHandle '" + mywindows + "' and title/pattern '" + pattern + "'.");
// if a REGEXP pattern was supplied use as a regular expression and match against the window title of the current window handle
if (pattern.startsWith(REGEXPI_SYNTAX)) {
Pattern compiledPattern = Pattern.compile(pattern.substring(REGEXPI_SYNTAX.length()), Pattern.CASE_INSENSITIVE + Pattern.DOTALL);
foundCorrectWindow = compiledPattern.matcher(windowTitleForHandle).matches();
} else if (pattern.startsWith(REGEX_SYNTAX)) {
Pattern compiledPattern = Pattern.compile(pattern.substring(REGEX_SYNTAX.length()), Pattern.DOTALL);
foundCorrectWindow = compiledPattern.matcher(windowTitleForHandle).matches();
}
// otherwise do a direct comparison
else {
foundCorrectWindow = windowTitleForHandle.equals(pattern);
}
//Stop if the window was found, otherwise go back to the original
if (foundCorrectWindow) {
LOG.info("Found window with title '" + windowTitleForHandle + "' and handle '" + mywindows + "'.");
break;
} else {
LOG.info(" Window with title/pattern '" + pattern + "' not found (yet). Switching back to the original window.");
driver.switchTo().window(winHandleBefore);
}
}
}
static void switchToUnfocussedWindow() {
String currentHandle = driver.getWindowHandle();
Set allHandles = driver.getWindowHandles();
allHandles.remove(currentHandle);
if (!allHandles.isEmpty()) {
driver.switchTo().window(allHandles.iterator().next());
}
}
static String getWindowHandle() {
return driver.getWindowHandle();
}
static void scrollTo(String locator) {
WebElement element = findElement(locator);
JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver;
try {
javascriptExecutor.executeScript("arguments[0].scrollIntoView(true);", element);
isElementVisible(locator);
} catch (ConditionalException e) {
javascriptExecutor.executeScript("arguments[0].scrollIntoView(false);", element);
isElementVisible(locator);
}
}
static void scrollUpTo(String locator) {
WebElement element = findElement(locator);
JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver;
javascriptExecutor.executeScript("arguments[0].scrollIntoView(false);", element);
}
static void scrollDownTo(String locator) {
WebElement element = findElement(locator);
JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver;
javascriptExecutor.executeScript("arguments[0].scrollIntoView(true);", element);
}
static void enableTextbox(String locator) {
JavascriptExecutor javascript = (JavascriptExecutor) driver;
String toenable = "document.getElementsByName('lname')[0].removeAttribute('disabled');";
javascript.executeScript(toenable);
}
static void disableTextbox(String locator) {
JavascriptExecutor javascript = (JavascriptExecutor) driver;
String todisable = "document.getElementsByName('fname')[0].setAttribute('disabled', '');";
javascript.executeScript(todisable);
}
static void executeJavaScript(String script) {
try {
JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver;
javascriptExecutor.executeScript(script);
} catch (Exception e) {
throw new ConditionalException("Failed to execute javascript.");
}
}
static String executeJavaScriptWithReturnValue(String script) {
JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver;
return javascriptExecutor.executeScript(script).toString();
}
static void uploadFile(String browser, final String locator, final String fileName) {
WebElement element = findElement(locator);
if (browser.equalsIgnoreCase("remote")) {
((RemoteWebElement) element).setFileDetector(new LocalFileDetector());
element.sendKeys(fileName);
} else {
element.sendKeys(fileName);
}
}
static void uploadFile(String browser, final String locator, String fileLocation, String fileName) {
final String fileToUpload = getWorkingDir() + fileLocation + fileName;
WebElement element = findElement(locator);
if (browser.equalsIgnoreCase("remote")) {
((RemoteWebElement) element).setFileDetector(new LocalFileDetector());
element.sendKeys(fileToUpload);
} else {
element.sendKeys(fileToUpload);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy