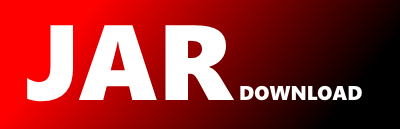
nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureWaits Maven / Gradle / Ivy
The newest version!
package nl.psek.fitnesse.fixtures.selenium;
import nl.psek.fitnesse.ConditionalException;
import nl.psek.fitnesse.fixtures.util.FixtureOperations;
import org.openqa.selenium.*;
import org.openqa.selenium.support.ui.FluentWait;
import org.openqa.selenium.support.ui.Wait;
import java.time.Duration;
import java.util.function.Function;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureCommon.*;
import static nl.psek.fitnesse.fixtures.selenium.SeleniumFixtureVerifyElements.*;
/**
* Methods used for waiting on elements
*/
public class SeleniumFixtureWaits {
protected static Duration DEFAULTFLUENTWAITTIMEOUT = Duration.ofSeconds(30);
protected static Duration DEFAULTFLUENTWAITINTERVAL = Duration.ofSeconds(1);
static boolean waitForElementPresentWithFluentWait(WebDriver driver, String locator) {
WebElement element = (WebElement) newFluentWait(driver).until(new Function() {
public WebElement apply(WebDriver driver) {
return driver.findElement(constructLocatorFromString(locator));
}
});
return notNullOrEmpty(element);
}
static boolean waitForElementNotPresentWithFluentWait(WebDriver driver, String locator) {
if (!verifyElementPresent(locator)) {
return true;
} else {
Boolean present = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return driver.findElements(constructLocatorFromString(locator)).isEmpty();
}
});
return present;
}
}
static boolean waitForPossibleElementNotPresent(String locator) {
if (driver.findElements(constructLocatorFromString(locator)).isEmpty()) {
return true;
} else {
Duration originalValue = DEFAULTFLUENTWAITTIMEOUT;
DEFAULTFLUENTWAITTIMEOUT = Duration.ofSeconds(5);
return (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver ignored) {
try {
return driver.findElements(constructLocatorFromString(locator)).isEmpty();
} catch (NoSuchElementException e) {
// Returns true because the element is not present in DOM. The
// try block checks if the element is present but is invisible.
return true;
} catch (StaleElementReferenceException e) {
// Returns true because stale element reference implies that element
// is no longer visible.
return true;
} finally {
DEFAULTFLUENTWAITTIMEOUT = originalValue;
LOG.info("reset fluentwait timeout to " + originalValue.getSeconds() + " seconds");
}
}
});
}
}
static void waitForAlertWithFluentWait(WebDriver driver) {
try {
Alert alert = (Alert) newFluentWait(driver).until(new Function() {
public Alert apply(WebDriver driver) {
try {
return driver.switchTo().alert();
} catch (NoAlertPresentException e) {
return null;
}
}
});
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Timeout while waiting for message dialog.");
} catch (WebDriverException webdriverException) {
throw new ConditionalException("WebDriver exception while waiting for message dialog.");
}
}
static void waitForAlertAndAcceptWithFluentWait(WebDriver driver) {
try {
Alert alert = (Alert) newFluentWait(driver).until(new Function() {
public Alert apply(WebDriver driver) {
try {
return driver.switchTo().alert();
} catch (NoAlertPresentException e) {
return null;
}
}
});
alert.accept();
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Timeout while waiting for message dialog.");
} catch (WebDriverException webdriverException) {
throw new ConditionalException("WebDriver exception while waiting for message dialog.");
}
}
static void waitForPossibleAlertAndAcceptWithFluentWait(WebDriver driver) {
try {
Alert alert = (Alert) newFluentWait(driver).until(new Function() {
public Alert apply(WebDriver driver) {
try {
return driver.switchTo().alert();
} catch (NoAlertPresentException e) {
return null;
}
}
});
alert.accept();
} catch (TimeoutException timeoutException) {
LOG.info("No alert during wait time, continuing");
} catch (WebDriverException webdriverException) {
throw new ConditionalException("WebDriver exception while waiting for message dialog.");
}
}
static void waitForAlertAndDismissWithFluentWait(WebDriver driver) {
try {
Alert alert = (Alert) newFluentWait(driver).until(new Function() {
public Alert apply(WebDriver driver) {
try {
return driver.switchTo().alert();
} catch (NoAlertPresentException e) {
return null;
}
}
});
alert.dismiss();
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Timeout while waiting for message dialog.");
} catch (WebDriverException webdriverException) {
throw new ConditionalException("WebDriver exception while waiting for message dialog.");
}
}
static void waitForPossibleAlertAndDismissWithFluentWait(WebDriver driver) {
try {
Alert alert = (Alert) newFluentWait(driver).until(new Function() {
public Alert apply(WebDriver driver) {
try {
return driver.switchTo().alert();
} catch (NoAlertPresentException e) {
return null;
}
}
});
alert.dismiss();
} catch (TimeoutException timeoutException) {
LOG.info("No alert during wait time, continuing");
} catch (WebDriverException webdriverException) {
throw new ConditionalException("WebDriver exception while waiting for message dialog.");
}
}
static void waitForEditableWithFluentWait(String locator) {
WebElement element = findElement(locator);
if (element != null && element.isDisplayed() && element.isEnabled()) {
LOG.info("Element " + locator + "is now editable");
} else try {
element = (WebElement) newFluentWait(driver).until(new Function() {
public WebElement apply(WebDriver driver) {
WebElement webElement = driver.findElement(constructLocatorFromString(locator));
if (webElement != null && webElement.isDisplayed() && webElement.isEnabled()) {
return webElement;
} else {
return null;
}
}
});
if (element == null) {
throw new ConditionalException("Element with locator '%s' did not become editable within '%d' seconds.", locator);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Element with locator '%s' did not become editable within '%d' seconds, a timeout occurred.", locator, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
}
static void waitForNotEditableWithFluentWait(String locator) {
WebElement element = findElement(locator);
if (element != null && element.isDisplayed() && element.isEnabled()) {
LOG.info("Element " + locator + "is now editable");
} else try {
element = (WebElement) newFluentWait(driver).until(new Function() {
public WebElement apply(WebDriver driver) {
WebElement webElement = driver.findElement(constructLocatorFromString(locator));
if (webElement != null && webElement.isDisplayed() && !webElement.isEnabled()) {
return webElement;
} else {
return null;
}
}
});
if (element == null) {
throw new ConditionalException("Element with locator '%s' did not become not editable within '%d' seconds.", locator);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Element with locator '%s' did not become not editable within '%d' seconds, a timeout occurred.", locator, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
}
static void waitForVisibleWithFluentWait(String locator) {
WebElement element = findElement(locator);
if (element != null && element.isDisplayed()) {
LOG.info("Element " + locator + "is now visible");
} else try {
element = (WebElement) newFluentWait(driver).until(new Function() {
public WebElement apply(WebDriver driver) {
WebElement webElement = driver.findElement(constructLocatorFromString(locator));
if (webElement != null && webElement.isDisplayed()) {
return webElement;
} else {
return null;
}
}
});
if (element == null) {
throw new ConditionalException("Element with locator '%s' did not become visible within '%d' seconds.", locator);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Element with locator '%s' did not become visible within '%d' seconds, a timeout occurred.", locator, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
}
static void waitForNotVisibleWithFluentWait(String locator) {
WebElement element = findElement(locator);
if (element != null && !element.isDisplayed()) {
LOG.info("Element " + locator + "is now not visible");
} else try {
element = (WebElement) newFluentWait(driver).until(new Function() {
public WebElement apply(WebDriver driver) {
WebElement webElement = driver.findElement(constructLocatorFromString(locator));
if (webElement != null && !webElement.isDisplayed()) {
return webElement;
} else {
return null;
}
}
});
if (element == null) {
throw new ConditionalException("Element with locator '%s' did not become not visible within '%d' seconds.", locator);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("Element with locator '%s' did not become not visible within '%d' seconds, a timeout occurred.", locator, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
}
static boolean waitForTextPresent(String pattern) {
if (verifyPatternPresent(pattern)) {
return true;
} else try {
Boolean result = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return FixtureOperations.containsPatternNoInputLogging(driver.getPageSource(), pattern);
}
}
);
} catch (TimeoutException timeoutException) {
throw new ConditionalException("The text '%s' did not become present within '%d' seconds, a timeout occurred.", pattern, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
return false;
}
static boolean waitForTextNotPresent(String pattern) {
if (!verifyPatternPresent(pattern)) {
return true;
} else try {
Boolean result = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return !FixtureOperations.containsPatternNoInputLogging(driver.getPageSource(), pattern);
}
}
);
} catch (TimeoutException timeoutException) {
throw new ConditionalException("The text '%s' did not become not present (i.e. absent) within '%d' seconds, a timeout occurred.", pattern, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
return false;
}
static boolean waitForText(String locator, String pattern) {
if (verifyText(locator, pattern)) {
return true;
} else try {
Boolean present = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return verifyText(locator, pattern);
}
}
);
if (!present) {
throw new ConditionalException("The element with locator '%s' did not have the text '%s'.", locator, pattern);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("The element with locator '%s' did not have the text '%s', a timeout occurred.", locator, pattern);
}
return true;
}
static boolean waitForNotText(String locator, String pattern) {
if (!verifyText(locator, pattern)) {
return true;
} else try {
Boolean present = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return !verifyText(locator, pattern);
}
}
);
if (!present) {
throw new ConditionalException("The element with locator '%s' still has the text '%s'.", locator, pattern);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("The element with locator '%s' still has the text '%s', a timeout occurred.", locator, pattern);
}
return true;
}
static boolean waitForValue(String locator, String value) {
if (verifyValue(locator, value)) {
return true;
} else try {
Boolean present = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return verifyValue(locator, value);
}
}
);
if (!present) {
throw new ConditionalException("The element with locator '%s' did not have the value '%s'.", locator, value);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("The element with locator '%s' did not have the value '%s', a timeout occurred.", locator, value);
}
return true;
}
static boolean waitForValueNotEmpty(String locator) {
if (verifyValueNotEmpty(locator)) {
return true;
} else try {
Boolean notEmpty = (Boolean) newFluentWait(driver).until(new Function() {
public Boolean apply(WebDriver driver) {
return verifyValueNotEmpty(locator);
}
}
);
if (!notEmpty) {
throw new ConditionalException("The element at the locator '%s' did not have a value.", locator);
}
} catch (TimeoutException timeoutException) {
throw new ConditionalException("The element with locator '%s' still not has a value after '%d' seconds, a timeout occurred.", locator, DEFAULTFLUENTWAITTIMEOUT.getSeconds());
}
return true;
}
static Wait newFluentWait(WebDriver driver) {
return new FluentWait(driver)
.withTimeout(DEFAULTFLUENTWAITTIMEOUT)
.pollingEvery(DEFAULTFLUENTWAITINTERVAL)
.ignoring(Exception.class);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy