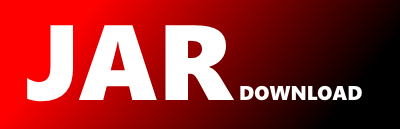
nl.reinkrul.nuts.auth.Contract Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Auth Service API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.auth;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Contract
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:35.626800+02:00[Europe/Amsterdam]")
public class Contract {
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public static final String SERIALIZED_NAME_LANGUAGE = "language";
@SerializedName(SERIALIZED_NAME_LANGUAGE)
private String language;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private String version;
public static final String SERIALIZED_NAME_SIGNER_ATTRIBUTES = "signer_attributes";
@SerializedName(SERIALIZED_NAME_SIGNER_ATTRIBUTES)
private List signerAttributes = null;
public static final String SERIALIZED_NAME_TEMPLATE = "template";
@SerializedName(SERIALIZED_NAME_TEMPLATE)
private String template;
public static final String SERIALIZED_NAME_TEMPLATE_ATTRIBUTES = "template_attributes";
@SerializedName(SERIALIZED_NAME_TEMPLATE_ATTRIBUTES)
private List templateAttributes = null;
public Contract type(String type) {
this.type = type;
return this;
}
/**
* Type of which contract to sign.
* @return type
**/
@ApiModelProperty(example = "BehandelaarLogin", required = true, value = "Type of which contract to sign.")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Contract language(String language) {
this.language = language;
return this;
}
/**
* Language of the contract in all caps.
* @return language
**/
@ApiModelProperty(example = "NL", required = true, value = "Language of the contract in all caps.")
public String getLanguage() {
return language;
}
public void setLanguage(String language) {
this.language = language;
}
public Contract version(String version) {
this.version = version;
return this;
}
/**
* Version of the contract.
* @return version
**/
@ApiModelProperty(example = "v1", required = true, value = "Version of the contract.")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public Contract signerAttributes(List signerAttributes) {
this.signerAttributes = signerAttributes;
return this;
}
public Contract addSignerAttributesItem(String signerAttributesItem) {
if (this.signerAttributes == null) {
this.signerAttributes = new ArrayList();
}
this.signerAttributes.add(signerAttributesItem);
return this;
}
/**
* Get signerAttributes
* @return signerAttributes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getSignerAttributes() {
return signerAttributes;
}
public void setSignerAttributes(List signerAttributes) {
this.signerAttributes = signerAttributes;
}
public Contract template(String template) {
this.template = template;
return this;
}
/**
* Get template
* @return template
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "ik verklaar dat ${acting_party} namens mij request mag maken", value = "")
public String getTemplate() {
return template;
}
public void setTemplate(String template) {
this.template = template;
}
public Contract templateAttributes(List templateAttributes) {
this.templateAttributes = templateAttributes;
return this;
}
public Contract addTemplateAttributesItem(String templateAttributesItem) {
if (this.templateAttributes == null) {
this.templateAttributes = new ArrayList();
}
this.templateAttributes.add(templateAttributesItem);
return this;
}
/**
* Get templateAttributes
* @return templateAttributes
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"irma-demo.MijnOverheid.ageLower.over12\",\"irma-demo.MijnOverheid.fullName\"]", value = "")
public List getTemplateAttributes() {
return templateAttributes;
}
public void setTemplateAttributes(List templateAttributes) {
this.templateAttributes = templateAttributes;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Contract contract = (Contract) o;
return Objects.equals(this.type, contract.type) &&
Objects.equals(this.language, contract.language) &&
Objects.equals(this.version, contract.version) &&
Objects.equals(this.signerAttributes, contract.signerAttributes) &&
Objects.equals(this.template, contract.template) &&
Objects.equals(this.templateAttributes, contract.templateAttributes);
}
@Override
public int hashCode() {
return Objects.hash(type, language, version, signerAttributes, template, templateAttributes);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Contract {\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" language: ").append(toIndentedString(language)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" signerAttributes: ").append(toIndentedString(signerAttributes)).append("\n");
sb.append(" template: ").append(toIndentedString(template)).append("\n");
sb.append(" templateAttributes: ").append(toIndentedString(templateAttributes)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy