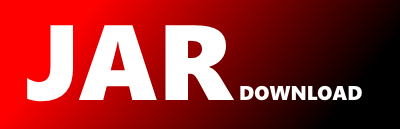
nl.reinkrul.nuts.auth.TokenIntrospectionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Auth Service API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.auth;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import nl.reinkrul.nuts.auth.VerifiableCredential;
/**
* Token introspection response as described in RFC7662 section 2.2
*/
@ApiModel(description = "Token introspection response as described in RFC7662 section 2.2")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:35.626800+02:00[Europe/Amsterdam]")
public class TokenIntrospectionResponse {
public static final String SERIALIZED_NAME_ACTIVE = "active";
@SerializedName(SERIALIZED_NAME_ACTIVE)
private Boolean active;
public static final String SERIALIZED_NAME_SERVICE = "service";
@SerializedName(SERIALIZED_NAME_SERVICE)
private String service;
public static final String SERIALIZED_NAME_ISS = "iss";
@SerializedName(SERIALIZED_NAME_ISS)
private String iss;
public static final String SERIALIZED_NAME_SUB = "sub";
@SerializedName(SERIALIZED_NAME_SUB)
private String sub;
public static final String SERIALIZED_NAME_AUD = "aud";
@SerializedName(SERIALIZED_NAME_AUD)
private String aud;
public static final String SERIALIZED_NAME_VCS = "vcs";
@SerializedName(SERIALIZED_NAME_VCS)
private List vcs = null;
public static final String SERIALIZED_NAME_RESOLVED_V_CS = "resolvedVCs";
@SerializedName(SERIALIZED_NAME_RESOLVED_V_CS)
private List resolvedVCs = null;
public static final String SERIALIZED_NAME_OSI = "osi";
@SerializedName(SERIALIZED_NAME_OSI)
private String osi;
public static final String SERIALIZED_NAME_EXP = "exp";
@SerializedName(SERIALIZED_NAME_EXP)
private Integer exp;
public static final String SERIALIZED_NAME_IAT = "iat";
@SerializedName(SERIALIZED_NAME_IAT)
private Integer iat;
public static final String SERIALIZED_NAME_FAMILY_NAME = "family_name";
@SerializedName(SERIALIZED_NAME_FAMILY_NAME)
private String familyName;
public static final String SERIALIZED_NAME_PREFIX = "prefix";
@SerializedName(SERIALIZED_NAME_PREFIX)
private String prefix;
public static final String SERIALIZED_NAME_INITIALS = "initials";
@SerializedName(SERIALIZED_NAME_INITIALS)
private String initials;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public TokenIntrospectionResponse active(Boolean active) {
this.active = active;
return this;
}
/**
* True if the token is active, false if the token is expired, malformed etc.
* @return active
**/
@ApiModelProperty(required = true, value = "True if the token is active, false if the token is expired, malformed etc. ")
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public TokenIntrospectionResponse service(String service) {
this.service = service;
return this;
}
/**
* Get service
* @return service
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getService() {
return service;
}
public void setService(String service) {
this.service = service;
}
public TokenIntrospectionResponse iss(String iss) {
this.iss = iss;
return this;
}
/**
* The subject (not a Nuts subject) contains the DID of the authorizer.
* @return iss
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "did:nuts:128903fjgfslcnmgpe84", value = "The subject (not a Nuts subject) contains the DID of the authorizer. ")
public String getIss() {
return iss;
}
public void setIss(String iss) {
this.iss = iss;
}
public TokenIntrospectionResponse sub(String sub) {
this.sub = sub;
return this;
}
/**
* The subject is always the acting party, thus the care organization requesting access to data.
* @return sub
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "did:nuts:128903fjgfslcnmgpe84", value = "The subject is always the acting party, thus the care organization requesting access to data. ")
public String getSub() {
return sub;
}
public void setSub(String sub) {
this.sub = sub;
}
public TokenIntrospectionResponse aud(String aud) {
this.aud = aud;
return this;
}
/**
* As per rfc7523 https://tools.ietf.org/html/rfc7523>, the aud must be the token endpoint. This can be taken from the Nuts registry.
* @return aud
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://target_token_endpoint", value = "As per rfc7523 https://tools.ietf.org/html/rfc7523>, the aud must be the token endpoint. This can be taken from the Nuts registry. ")
public String getAud() {
return aud;
}
public void setAud(String aud) {
this.aud = aud;
}
public TokenIntrospectionResponse vcs(List vcs) {
this.vcs = vcs;
return this;
}
public TokenIntrospectionResponse addVcsItem(String vcsItem) {
if (this.vcs == null) {
this.vcs = new ArrayList();
}
this.vcs.add(vcsItem);
return this;
}
/**
* Get vcs
* @return vcs
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getVcs() {
return vcs;
}
public void setVcs(List vcs) {
this.vcs = vcs;
}
public TokenIntrospectionResponse resolvedVCs(List resolvedVCs) {
this.resolvedVCs = resolvedVCs;
return this;
}
public TokenIntrospectionResponse addResolvedVCsItem(VerifiableCredential resolvedVCsItem) {
if (this.resolvedVCs == null) {
this.resolvedVCs = new ArrayList();
}
this.resolvedVCs.add(resolvedVCsItem);
return this;
}
/**
* credentials resolved from `vcs` (VC IDs). It contains only those VCs that could be resolved.
* @return resolvedVCs
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "credentials resolved from `vcs` (VC IDs). It contains only those VCs that could be resolved.")
public List getResolvedVCs() {
return resolvedVCs;
}
public void setResolvedVCs(List resolvedVCs) {
this.resolvedVCs = resolvedVCs;
}
public TokenIntrospectionResponse osi(String osi) {
this.osi = osi;
return this;
}
/**
* encoded ops signature. (TBD)
* @return osi
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "encoded ops signature. (TBD)")
public String getOsi() {
return osi;
}
public void setOsi(String osi) {
this.osi = osi;
}
public TokenIntrospectionResponse exp(Integer exp) {
this.exp = exp;
return this;
}
/**
* Get exp
* @return exp
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getExp() {
return exp;
}
public void setExp(Integer exp) {
this.exp = exp;
}
public TokenIntrospectionResponse iat(Integer iat) {
this.iat = iat;
return this;
}
/**
* Get iat
* @return iat
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getIat() {
return iat;
}
public void setIat(Integer iat) {
this.iat = iat;
}
public TokenIntrospectionResponse familyName(String familyName) {
this.familyName = familyName;
return this;
}
/**
* Surname(s) or last name(s) of the End-User.
* @return familyName
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Bruijn", value = "Surname(s) or last name(s) of the End-User.")
public String getFamilyName() {
return familyName;
}
public void setFamilyName(String familyName) {
this.familyName = familyName;
}
public TokenIntrospectionResponse prefix(String prefix) {
this.prefix = prefix;
return this;
}
/**
* Surname prefix
* @return prefix
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "de", value = "Surname prefix")
public String getPrefix() {
return prefix;
}
public void setPrefix(String prefix) {
this.prefix = prefix;
}
public TokenIntrospectionResponse initials(String initials) {
this.initials = initials;
return this;
}
/**
* Initials of the End-User.
* @return initials
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "I.", value = "Initials of the End-User.")
public String getInitials() {
return initials;
}
public void setInitials(String initials) {
this.initials = initials;
}
public TokenIntrospectionResponse email(String email) {
this.email = email;
return this;
}
/**
* End-User's preferred e-mail address. Should be a personal email and can be used to uniquely identify a user. Just like the email used for an account.
* @return email
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "End-User's preferred e-mail address. Should be a personal email and can be used to uniquely identify a user. Just like the email used for an account.")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TokenIntrospectionResponse tokenIntrospectionResponse = (TokenIntrospectionResponse) o;
return Objects.equals(this.active, tokenIntrospectionResponse.active) &&
Objects.equals(this.service, tokenIntrospectionResponse.service) &&
Objects.equals(this.iss, tokenIntrospectionResponse.iss) &&
Objects.equals(this.sub, tokenIntrospectionResponse.sub) &&
Objects.equals(this.aud, tokenIntrospectionResponse.aud) &&
Objects.equals(this.vcs, tokenIntrospectionResponse.vcs) &&
Objects.equals(this.resolvedVCs, tokenIntrospectionResponse.resolvedVCs) &&
Objects.equals(this.osi, tokenIntrospectionResponse.osi) &&
Objects.equals(this.exp, tokenIntrospectionResponse.exp) &&
Objects.equals(this.iat, tokenIntrospectionResponse.iat) &&
Objects.equals(this.familyName, tokenIntrospectionResponse.familyName) &&
Objects.equals(this.prefix, tokenIntrospectionResponse.prefix) &&
Objects.equals(this.initials, tokenIntrospectionResponse.initials) &&
Objects.equals(this.email, tokenIntrospectionResponse.email);
}
@Override
public int hashCode() {
return Objects.hash(active, service, iss, sub, aud, vcs, resolvedVCs, osi, exp, iat, familyName, prefix, initials, email);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TokenIntrospectionResponse {\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" service: ").append(toIndentedString(service)).append("\n");
sb.append(" iss: ").append(toIndentedString(iss)).append("\n");
sb.append(" sub: ").append(toIndentedString(sub)).append("\n");
sb.append(" aud: ").append(toIndentedString(aud)).append("\n");
sb.append(" vcs: ").append(toIndentedString(vcs)).append("\n");
sb.append(" resolvedVCs: ").append(toIndentedString(resolvedVCs)).append("\n");
sb.append(" osi: ").append(toIndentedString(osi)).append("\n");
sb.append(" exp: ").append(toIndentedString(exp)).append("\n");
sb.append(" iat: ").append(toIndentedString(iat)).append("\n");
sb.append(" familyName: ").append(toIndentedString(familyName)).append("\n");
sb.append(" prefix: ").append(toIndentedString(prefix)).append("\n");
sb.append(" initials: ").append(toIndentedString(initials)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy