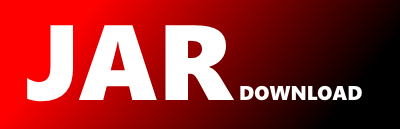
nl.reinkrul.nuts.auth.VerifiableCredential Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Auth Service API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.auth;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* A credential according to the W3C and Nuts specs.
*/
@ApiModel(description = "A credential according to the W3C and Nuts specs.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:35.626800+02:00[Europe/Amsterdam]")
public class VerifiableCredential {
public static final String SERIALIZED_NAME_AT_CONTEXT = "@context";
@SerializedName(SERIALIZED_NAME_AT_CONTEXT)
private Object atContext = null;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private List type = new ArrayList();
public static final String SERIALIZED_NAME_ISSUER = "issuer";
@SerializedName(SERIALIZED_NAME_ISSUER)
private String issuer;
public static final String SERIALIZED_NAME_ISSUANCE_DATE = "issuanceDate";
@SerializedName(SERIALIZED_NAME_ISSUANCE_DATE)
private String issuanceDate;
public static final String SERIALIZED_NAME_EXPIRATION_DATE = "expirationDate";
@SerializedName(SERIALIZED_NAME_EXPIRATION_DATE)
private String expirationDate;
public static final String SERIALIZED_NAME_CREDENTIAL_SUBJECT = "credentialSubject";
@SerializedName(SERIALIZED_NAME_CREDENTIAL_SUBJECT)
private Object credentialSubject = null;
public static final String SERIALIZED_NAME_PROOF = "proof";
@SerializedName(SERIALIZED_NAME_PROOF)
private Object proof = null;
public VerifiableCredential atContext(Object atContext) {
this.atContext = atContext;
return this;
}
/**
* List of URIs of JSON-LD contexts of the VC.
* @return atContext
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "List of URIs of JSON-LD contexts of the VC.")
public Object getAtContext() {
return atContext;
}
public void setAtContext(Object atContext) {
this.atContext = atContext;
}
public VerifiableCredential id(String id) {
this.id = id;
return this;
}
/**
* Credential ID. An URI wich uniquely identifies the credential e.g. the issuers DID concatenated with an uuid.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "did:nuts:123#B8PUHs2AUHbFF1xLLK4eZjgErEcMXHxs68FteY7NDtCY", value = "Credential ID. An URI wich uniquely identifies the credential e.g. the issuers DID concatenated with an uuid.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public VerifiableCredential type(List type) {
this.type = type;
return this;
}
public VerifiableCredential addTypeItem(String typeItem) {
this.type.add(typeItem);
return this;
}
/**
* A single string or array of strings. The value(s) indicate the type of credential. It should contain `VerifiableCredential`. Each type should be defined in the @context.
* @return type
**/
@ApiModelProperty(required = true, value = "A single string or array of strings. The value(s) indicate the type of credential. It should contain `VerifiableCredential`. Each type should be defined in the @context.")
public List getType() {
return type;
}
public void setType(List type) {
this.type = type;
}
public VerifiableCredential issuer(String issuer) {
this.issuer = issuer;
return this;
}
/**
* DID according to Nuts specification
* @return issuer
**/
@ApiModelProperty(example = "did:nuts:B8PUHs2AUHbFF1xLLK4eZjgErEcMXHxs68FteY7NDtCY", required = true, value = "DID according to Nuts specification")
public String getIssuer() {
return issuer;
}
public void setIssuer(String issuer) {
this.issuer = issuer;
}
public VerifiableCredential issuanceDate(String issuanceDate) {
this.issuanceDate = issuanceDate;
return this;
}
/**
* rfc3339 time string when the credential was issued.
* @return issuanceDate
**/
@ApiModelProperty(example = "2012-01-02T12:00:00Z", required = true, value = "rfc3339 time string when the credential was issued.")
public String getIssuanceDate() {
return issuanceDate;
}
public void setIssuanceDate(String issuanceDate) {
this.issuanceDate = issuanceDate;
}
public VerifiableCredential expirationDate(String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
/**
* rfc3339 time string until when the credential is valid.
* @return expirationDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2012-01-02T12:00:00Z", value = "rfc3339 time string until when the credential is valid.")
public String getExpirationDate() {
return expirationDate;
}
public void setExpirationDate(String expirationDate) {
this.expirationDate = expirationDate;
}
public VerifiableCredential credentialSubject(Object credentialSubject) {
this.credentialSubject = credentialSubject;
return this;
}
/**
* Subject of a Verifiable Credential identifying the holder and expressing claims.
* @return credentialSubject
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "Subject of a Verifiable Credential identifying the holder and expressing claims.")
public Object getCredentialSubject() {
return credentialSubject;
}
public void setCredentialSubject(Object credentialSubject) {
this.credentialSubject = credentialSubject;
}
public VerifiableCredential proof(Object proof) {
this.proof = proof;
return this;
}
/**
* one or multiple cryptographic proofs
* @return proof
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "one or multiple cryptographic proofs")
public Object getProof() {
return proof;
}
public void setProof(Object proof) {
this.proof = proof;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
VerifiableCredential verifiableCredential = (VerifiableCredential) o;
return Objects.equals(this.atContext, verifiableCredential.atContext) &&
Objects.equals(this.id, verifiableCredential.id) &&
Objects.equals(this.type, verifiableCredential.type) &&
Objects.equals(this.issuer, verifiableCredential.issuer) &&
Objects.equals(this.issuanceDate, verifiableCredential.issuanceDate) &&
Objects.equals(this.expirationDate, verifiableCredential.expirationDate) &&
Objects.equals(this.credentialSubject, verifiableCredential.credentialSubject) &&
Objects.equals(this.proof, verifiableCredential.proof);
}
@Override
public int hashCode() {
return Objects.hash(atContext, id, type, issuer, issuanceDate, expirationDate, credentialSubject, proof);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class VerifiableCredential {\n");
sb.append(" atContext: ").append(toIndentedString(atContext)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" issuer: ").append(toIndentedString(issuer)).append("\n");
sb.append(" issuanceDate: ").append(toIndentedString(issuanceDate)).append("\n");
sb.append(" expirationDate: ").append(toIndentedString(expirationDate)).append("\n");
sb.append(" credentialSubject: ").append(toIndentedString(credentialSubject)).append("\n");
sb.append(" proof: ").append(toIndentedString(proof)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy