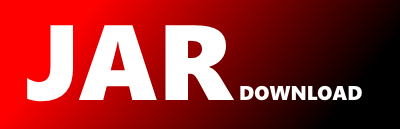
nl.reinkrul.nuts.didman.DIDDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts DID Manager API spec
* API specification for DID management helper APIs. The goal of this API is to help administrative interfaces to manage DIDs.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.didman;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import nl.reinkrul.nuts.didman.Service;
import nl.reinkrul.nuts.didman.VerificationMethod;
/**
* A DID document according to the W3C spec following the Nuts Method rules as defined in [Nuts RFC006]
*/
@ApiModel(description = "A DID document according to the W3C spec following the Nuts Method rules as defined in [Nuts RFC006]")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:35.164179+02:00[Europe/Amsterdam]")
public class DIDDocument {
public static final String SERIALIZED_NAME_AT_CONTEXT = "@context";
@SerializedName(SERIALIZED_NAME_AT_CONTEXT)
private Object atContext = null;
public static final String SERIALIZED_NAME_CONTROLLER = "controller";
@SerializedName(SERIALIZED_NAME_CONTROLLER)
private Object controller = null;
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_KEY_AGREEMENT = "keyAgreement";
@SerializedName(SERIALIZED_NAME_KEY_AGREEMENT)
private List keyAgreement = null;
public static final String SERIALIZED_NAME_SERVICE = "service";
@SerializedName(SERIALIZED_NAME_SERVICE)
private List service = null;
public static final String SERIALIZED_NAME_VERIFICATION_METHOD = "verificationMethod";
@SerializedName(SERIALIZED_NAME_VERIFICATION_METHOD)
private List verificationMethod = null;
public static final String SERIALIZED_NAME_ASSERTION_METHOD = "assertionMethod";
@SerializedName(SERIALIZED_NAME_ASSERTION_METHOD)
private List assertionMethod = null;
public static final String SERIALIZED_NAME_AUTHENTICATION = "authentication";
@SerializedName(SERIALIZED_NAME_AUTHENTICATION)
private List authentication = null;
public static final String SERIALIZED_NAME_CAPABILITY_DELEGATION = "capabilityDelegation";
@SerializedName(SERIALIZED_NAME_CAPABILITY_DELEGATION)
private List capabilityDelegation = null;
public static final String SERIALIZED_NAME_CAPABILITY_INVOCATION = "capabilityInvocation";
@SerializedName(SERIALIZED_NAME_CAPABILITY_INVOCATION)
private List capabilityInvocation = null;
public DIDDocument atContext(Object atContext) {
this.atContext = atContext;
return this;
}
/**
* The JSON-LD contexts that define the types used in this document. Can be a single string, or a list of strings.
* @return atContext
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The JSON-LD contexts that define the types used in this document. Can be a single string, or a list of strings.")
public Object getAtContext() {
return atContext;
}
public void setAtContext(Object atContext) {
this.atContext = atContext;
}
public DIDDocument controller(Object controller) {
this.controller = controller;
return this;
}
/**
* Single DID (as string) or List of DIDs that have control over the DID document
* @return controller
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Single DID (as string) or List of DIDs that have control over the DID document")
public Object getController() {
return controller;
}
public void setController(Object controller) {
this.controller = controller;
}
public DIDDocument id(String id) {
this.id = id;
return this;
}
/**
* DID according to Nuts specification
* @return id
**/
@ApiModelProperty(example = "did:nuts:1", required = true, value = "DID according to Nuts specification")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public DIDDocument keyAgreement(List keyAgreement) {
this.keyAgreement = keyAgreement;
return this;
}
public DIDDocument addKeyAgreementItem(String keyAgreementItem) {
if (this.keyAgreement == null) {
this.keyAgreement = new ArrayList();
}
this.keyAgreement.add(keyAgreementItem);
return this;
}
/**
* List of KIDs that can be used for encryption
* @return keyAgreement
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of KIDs that can be used for encryption")
public List getKeyAgreement() {
return keyAgreement;
}
public void setKeyAgreement(List keyAgreement) {
this.keyAgreement = keyAgreement;
}
public DIDDocument service(List service) {
this.service = service;
return this;
}
public DIDDocument addServiceItem(Service serviceItem) {
if (this.service == null) {
this.service = new ArrayList();
}
this.service.add(serviceItem);
return this;
}
/**
* List of supported services by the DID subject
* @return service
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of supported services by the DID subject")
public List getService() {
return service;
}
public void setService(List service) {
this.service = service;
}
public DIDDocument verificationMethod(List verificationMethod) {
this.verificationMethod = verificationMethod;
return this;
}
public DIDDocument addVerificationMethodItem(VerificationMethod verificationMethodItem) {
if (this.verificationMethod == null) {
this.verificationMethod = new ArrayList();
}
this.verificationMethod.add(verificationMethodItem);
return this;
}
/**
* list of keys
* @return verificationMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "list of keys")
public List getVerificationMethod() {
return verificationMethod;
}
public void setVerificationMethod(List verificationMethod) {
this.verificationMethod = verificationMethod;
}
public DIDDocument assertionMethod(List assertionMethod) {
this.assertionMethod = assertionMethod;
return this;
}
public DIDDocument addAssertionMethodItem(String assertionMethodItem) {
if (this.assertionMethod == null) {
this.assertionMethod = new ArrayList();
}
this.assertionMethod.add(assertionMethodItem);
return this;
}
/**
* List of KIDs that may sign JWTs, JWSs and VCs
* @return assertionMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of KIDs that may sign JWTs, JWSs and VCs")
public List getAssertionMethod() {
return assertionMethod;
}
public void setAssertionMethod(List assertionMethod) {
this.assertionMethod = assertionMethod;
}
public DIDDocument authentication(List authentication) {
this.authentication = authentication;
return this;
}
public DIDDocument addAuthenticationItem(String authenticationItem) {
if (this.authentication == null) {
this.authentication = new ArrayList();
}
this.authentication.add(authenticationItem);
return this;
}
/**
* List of KIDs that may alter DID documents that they control
* @return authentication
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of KIDs that may alter DID documents that they control")
public List getAuthentication() {
return authentication;
}
public void setAuthentication(List authentication) {
this.authentication = authentication;
}
public DIDDocument capabilityDelegation(List capabilityDelegation) {
this.capabilityDelegation = capabilityDelegation;
return this;
}
public DIDDocument addCapabilityDelegationItem(String capabilityDelegationItem) {
if (this.capabilityDelegation == null) {
this.capabilityDelegation = new ArrayList();
}
this.capabilityDelegation.add(capabilityDelegationItem);
return this;
}
/**
* List of KIDs that can be used to delegate capabilities that can be invoked using the DID document.
* @return capabilityDelegation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of KIDs that can be used to delegate capabilities that can be invoked using the DID document.")
public List getCapabilityDelegation() {
return capabilityDelegation;
}
public void setCapabilityDelegation(List capabilityDelegation) {
this.capabilityDelegation = capabilityDelegation;
}
public DIDDocument capabilityInvocation(List capabilityInvocation) {
this.capabilityInvocation = capabilityInvocation;
return this;
}
public DIDDocument addCapabilityInvocationItem(String capabilityInvocationItem) {
if (this.capabilityInvocation == null) {
this.capabilityInvocation = new ArrayList();
}
this.capabilityInvocation.add(capabilityInvocationItem);
return this;
}
/**
* List of KIDs that can be used for signing
* @return capabilityInvocation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of KIDs that can be used for signing")
public List getCapabilityInvocation() {
return capabilityInvocation;
}
public void setCapabilityInvocation(List capabilityInvocation) {
this.capabilityInvocation = capabilityInvocation;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DIDDocument diDDocument = (DIDDocument) o;
return Objects.equals(this.atContext, diDDocument.atContext) &&
Objects.equals(this.controller, diDDocument.controller) &&
Objects.equals(this.id, diDDocument.id) &&
Objects.equals(this.keyAgreement, diDDocument.keyAgreement) &&
Objects.equals(this.service, diDDocument.service) &&
Objects.equals(this.verificationMethod, diDDocument.verificationMethod) &&
Objects.equals(this.assertionMethod, diDDocument.assertionMethod) &&
Objects.equals(this.authentication, diDDocument.authentication) &&
Objects.equals(this.capabilityDelegation, diDDocument.capabilityDelegation) &&
Objects.equals(this.capabilityInvocation, diDDocument.capabilityInvocation);
}
@Override
public int hashCode() {
return Objects.hash(atContext, controller, id, keyAgreement, service, verificationMethod, assertionMethod, authentication, capabilityDelegation, capabilityInvocation);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DIDDocument {\n");
sb.append(" atContext: ").append(toIndentedString(atContext)).append("\n");
sb.append(" controller: ").append(toIndentedString(controller)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" keyAgreement: ").append(toIndentedString(keyAgreement)).append("\n");
sb.append(" service: ").append(toIndentedString(service)).append("\n");
sb.append(" verificationMethod: ").append(toIndentedString(verificationMethod)).append("\n");
sb.append(" assertionMethod: ").append(toIndentedString(assertionMethod)).append("\n");
sb.append(" authentication: ").append(toIndentedString(authentication)).append("\n");
sb.append(" capabilityDelegation: ").append(toIndentedString(capabilityDelegation)).append("\n");
sb.append(" capabilityInvocation: ").append(toIndentedString(capabilityInvocation)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy