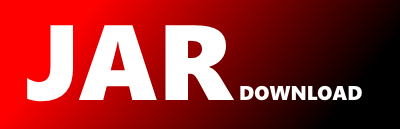
nl.reinkrul.nuts.vcr.v2.CreateVPRequest Maven / Gradle / Ivy
/*
* Nuts Verifiable Credential API spec
* API specification for common operations on Verifiable credentials. It allows the three roles, issuer, holder and verifier to issue, revoke, search, present and verify credentials.
*
* The version of the OpenAPI document: 2.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.vcr.v2;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import nl.reinkrul.nuts.vcr.v2.VerifiableCredential;
/**
* A request for creating a new Verifiable Presentation for a set of Verifiable Credentials.
*/
@ApiModel(description = "A request for creating a new Verifiable Presentation for a set of Verifiable Credentials.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:34.811142+02:00[Europe/Amsterdam]")
public class CreateVPRequest {
public static final String SERIALIZED_NAME_VERIFIABLE_CREDENTIALS = "verifiableCredentials";
@SerializedName(SERIALIZED_NAME_VERIFIABLE_CREDENTIALS)
private List verifiableCredentials = new ArrayList();
public static final String SERIALIZED_NAME_SIGNER_D_I_D = "signerDID";
@SerializedName(SERIALIZED_NAME_SIGNER_D_I_D)
private URI signerDID;
public static final String SERIALIZED_NAME_PROOF_PURPOSE = "proofPurpose";
@SerializedName(SERIALIZED_NAME_PROOF_PURPOSE)
private String proofPurpose;
public static final String SERIALIZED_NAME_CHALLENGE = "challenge";
@SerializedName(SERIALIZED_NAME_CHALLENGE)
private String challenge;
public static final String SERIALIZED_NAME_DOMAIN = "domain";
@SerializedName(SERIALIZED_NAME_DOMAIN)
private String domain;
public static final String SERIALIZED_NAME_EXPIRES = "expires";
@SerializedName(SERIALIZED_NAME_EXPIRES)
private String expires;
public CreateVPRequest verifiableCredentials(List verifiableCredentials) {
this.verifiableCredentials = verifiableCredentials;
return this;
}
public CreateVPRequest addVerifiableCredentialsItem(VerifiableCredential verifiableCredentialsItem) {
this.verifiableCredentials.add(verifiableCredentialsItem);
return this;
}
/**
* Get verifiableCredentials
* @return verifiableCredentials
**/
@ApiModelProperty(required = true, value = "")
public List getVerifiableCredentials() {
return verifiableCredentials;
}
public void setVerifiableCredentials(List verifiableCredentials) {
this.verifiableCredentials = verifiableCredentials;
}
public CreateVPRequest signerDID(URI signerDID) {
this.signerDID = signerDID;
return this;
}
/**
* Specifies the DID of the signing party that must be used to create the digital signature. If not specified, it is derived from the given Verifiable Credentials' subjectCredential ID. It can only be derived if all given Verifiable Credentials have the same, single subjectCredential.
* @return signerDID
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the DID of the signing party that must be used to create the digital signature. If not specified, it is derived from the given Verifiable Credentials' subjectCredential ID. It can only be derived if all given Verifiable Credentials have the same, single subjectCredential. ")
public URI getSignerDID() {
return signerDID;
}
public void setSignerDID(URI signerDID) {
this.signerDID = signerDID;
}
public CreateVPRequest proofPurpose(String proofPurpose) {
this.proofPurpose = proofPurpose;
return this;
}
/**
* The specific intent for the proof, the reason why an entity created it. Acts as a safeguard to prevent the proof from being misused for a purpose other than the one it was intended for.
* @return proofPurpose
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The specific intent for the proof, the reason why an entity created it. Acts as a safeguard to prevent the proof from being misused for a purpose other than the one it was intended for. ")
public String getProofPurpose() {
return proofPurpose;
}
public void setProofPurpose(String proofPurpose) {
this.proofPurpose = proofPurpose;
}
public CreateVPRequest challenge(String challenge) {
this.challenge = challenge;
return this;
}
/**
* A random or pseudo-random value used by some authentication protocols to mitigate replay attacks.
* @return challenge
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A random or pseudo-random value used by some authentication protocols to mitigate replay attacks. ")
public String getChallenge() {
return challenge;
}
public void setChallenge(String challenge) {
this.challenge = challenge;
}
public CreateVPRequest domain(String domain) {
this.domain = domain;
return this;
}
/**
* A string value that specifies the operational domain of a digital proof. This could be an Internet domain name like example.com, an ad-hoc value such as mycorp-level3-access, or a very specific transaction value like 8zF6T$mqP. A signer could include a domain in its digital proof to restrict its use to particular target, identified by the specified domain.
* @return domain
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A string value that specifies the operational domain of a digital proof. This could be an Internet domain name like example.com, an ad-hoc value such as mycorp-level3-access, or a very specific transaction value like 8zF6T$mqP. A signer could include a domain in its digital proof to restrict its use to particular target, identified by the specified domain. ")
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public CreateVPRequest expires(String expires) {
this.expires = expires;
return this;
}
/**
* Date and time at which proof will expire. If omitted, the proof does not have an end date.
* @return expires
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2021-12-20T09:00:00Z", value = "Date and time at which proof will expire. If omitted, the proof does not have an end date.")
public String getExpires() {
return expires;
}
public void setExpires(String expires) {
this.expires = expires;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateVPRequest createVPRequest = (CreateVPRequest) o;
return Objects.equals(this.verifiableCredentials, createVPRequest.verifiableCredentials) &&
Objects.equals(this.signerDID, createVPRequest.signerDID) &&
Objects.equals(this.proofPurpose, createVPRequest.proofPurpose) &&
Objects.equals(this.challenge, createVPRequest.challenge) &&
Objects.equals(this.domain, createVPRequest.domain) &&
Objects.equals(this.expires, createVPRequest.expires);
}
@Override
public int hashCode() {
return Objects.hash(verifiableCredentials, signerDID, proofPurpose, challenge, domain, expires);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateVPRequest {\n");
sb.append(" verifiableCredentials: ").append(toIndentedString(verifiableCredentials)).append("\n");
sb.append(" signerDID: ").append(toIndentedString(signerDID)).append("\n");
sb.append(" proofPurpose: ").append(toIndentedString(proofPurpose)).append("\n");
sb.append(" challenge: ").append(toIndentedString(challenge)).append("\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" expires: ").append(toIndentedString(expires)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy