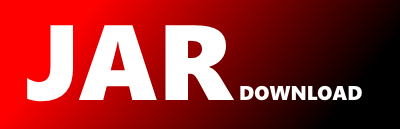
nl.reinkrul.nuts.vcr.v2.IssueVCRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Verifiable Credential API spec
* API specification for common operations on Verifiable credentials. It allows the three roles, issuer, holder and verifier to issue, revoke, search, present and verify credentials.
*
* The version of the OpenAPI document: 2.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.vcr.v2;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* A request for issuing a new Verifiable Credential.
*/
@ApiModel(description = "A request for issuing a new Verifiable Credential.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:34.811142+02:00[Europe/Amsterdam]")
public class IssueVCRequest {
public static final String SERIALIZED_NAME_AT_CONTEXT = "@context";
@SerializedName(SERIALIZED_NAME_AT_CONTEXT)
private String atContext = "https://nuts.nl/credentials/v1";
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public static final String SERIALIZED_NAME_ISSUER = "issuer";
@SerializedName(SERIALIZED_NAME_ISSUER)
private String issuer;
public static final String SERIALIZED_NAME_EXPIRATION_DATE = "expirationDate";
@SerializedName(SERIALIZED_NAME_EXPIRATION_DATE)
private String expirationDate;
public static final String SERIALIZED_NAME_PUBLISH_TO_NETWORK = "publishToNetwork";
@SerializedName(SERIALIZED_NAME_PUBLISH_TO_NETWORK)
private Boolean publishToNetwork = true;
/**
* When publishToNetwork is true, the credential can be published publicly or privately to the holder. This field is mandatory if publishToNetwork is true to prevent accidents. It defaults to \"private\".
*/
@JsonAdapter(VisibilityEnum.Adapter.class)
public enum VisibilityEnum {
PUBLIC("public"),
PRIVATE("private");
private String value;
VisibilityEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static VisibilityEnum fromValue(String value) {
for (VisibilityEnum b : VisibilityEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final VisibilityEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public VisibilityEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return VisibilityEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_VISIBILITY = "visibility";
@SerializedName(SERIALIZED_NAME_VISIBILITY)
private VisibilityEnum visibility = VisibilityEnum.PRIVATE;
public static final String SERIALIZED_NAME_CREDENTIAL_SUBJECT = "credentialSubject";
@SerializedName(SERIALIZED_NAME_CREDENTIAL_SUBJECT)
private Object credentialSubject = null;
public IssueVCRequest atContext(String atContext) {
this.atContext = atContext;
return this;
}
/**
* The resolvable context of the credentialSubject as URI. If omitted, the \"https://nuts.nl/credentials/v1\" context is used. Note: it is not needed to provide the \"https://www.w3.org/2018/credentials/v1\" context here.
* @return atContext
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "http://schema.org", value = "The resolvable context of the credentialSubject as URI. If omitted, the \"https://nuts.nl/credentials/v1\" context is used. Note: it is not needed to provide the \"https://www.w3.org/2018/credentials/v1\" context here. ")
public String getAtContext() {
return atContext;
}
public void setAtContext(String atContext) {
this.atContext = atContext;
}
public IssueVCRequest type(String type) {
this.type = type;
return this;
}
/**
* Type definition for the credential.
* @return type
**/
@ApiModelProperty(example = "NutsOrganizationCredential", required = true, value = "Type definition for the credential.")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public IssueVCRequest issuer(String issuer) {
this.issuer = issuer;
return this;
}
/**
* DID according to Nuts specification.
* @return issuer
**/
@ApiModelProperty(example = "did:nuts:B8PUHs2AUHbFF1xLLK4eZjgErEcMXHxs68FteY7NDtCY", required = true, value = "DID according to Nuts specification.")
public String getIssuer() {
return issuer;
}
public void setIssuer(String issuer) {
this.issuer = issuer;
}
public IssueVCRequest expirationDate(String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
/**
* rfc3339 time string until when the credential is valid.
* @return expirationDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2012-01-02T12:00:00Z", value = "rfc3339 time string until when the credential is valid.")
public String getExpirationDate() {
return expirationDate;
}
public void setExpirationDate(String expirationDate) {
this.expirationDate = expirationDate;
}
public IssueVCRequest publishToNetwork(Boolean publishToNetwork) {
this.publishToNetwork = publishToNetwork;
return this;
}
/**
* If set, the node publishes this credential to the network. This is the default behaviour. When set to false, the caller is responsible for distributing the VC to a holder. When the issuer is also the holder, it then can be used to directly create a presentation (self issued). Note: a not published credential can still be publicly revoked.
* @return publishToNetwork
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If set, the node publishes this credential to the network. This is the default behaviour. When set to false, the caller is responsible for distributing the VC to a holder. When the issuer is also the holder, it then can be used to directly create a presentation (self issued). Note: a not published credential can still be publicly revoked. ")
public Boolean getPublishToNetwork() {
return publishToNetwork;
}
public void setPublishToNetwork(Boolean publishToNetwork) {
this.publishToNetwork = publishToNetwork;
}
public IssueVCRequest visibility(VisibilityEnum visibility) {
this.visibility = visibility;
return this;
}
/**
* When publishToNetwork is true, the credential can be published publicly or privately to the holder. This field is mandatory if publishToNetwork is true to prevent accidents. It defaults to \"private\".
* @return visibility
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "When publishToNetwork is true, the credential can be published publicly or privately to the holder. This field is mandatory if publishToNetwork is true to prevent accidents. It defaults to \"private\". ")
public VisibilityEnum getVisibility() {
return visibility;
}
public void setVisibility(VisibilityEnum visibility) {
this.visibility = visibility;
}
public IssueVCRequest credentialSubject(Object credentialSubject) {
this.credentialSubject = credentialSubject;
return this;
}
/**
* Subject of a Verifiable Credential identifying the holder and expressing claims.
* @return credentialSubject
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "Subject of a Verifiable Credential identifying the holder and expressing claims.")
public Object getCredentialSubject() {
return credentialSubject;
}
public void setCredentialSubject(Object credentialSubject) {
this.credentialSubject = credentialSubject;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IssueVCRequest issueVCRequest = (IssueVCRequest) o;
return Objects.equals(this.atContext, issueVCRequest.atContext) &&
Objects.equals(this.type, issueVCRequest.type) &&
Objects.equals(this.issuer, issueVCRequest.issuer) &&
Objects.equals(this.expirationDate, issueVCRequest.expirationDate) &&
Objects.equals(this.publishToNetwork, issueVCRequest.publishToNetwork) &&
Objects.equals(this.visibility, issueVCRequest.visibility) &&
Objects.equals(this.credentialSubject, issueVCRequest.credentialSubject);
}
@Override
public int hashCode() {
return Objects.hash(atContext, type, issuer, expirationDate, publishToNetwork, visibility, credentialSubject);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IssueVCRequest {\n");
sb.append(" atContext: ").append(toIndentedString(atContext)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" issuer: ").append(toIndentedString(issuer)).append("\n");
sb.append(" expirationDate: ").append(toIndentedString(expirationDate)).append("\n");
sb.append(" publishToNetwork: ").append(toIndentedString(publishToNetwork)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append(" credentialSubject: ").append(toIndentedString(credentialSubject)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy