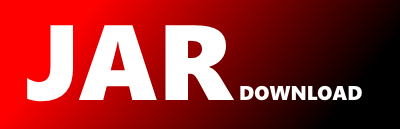
nl.reinkrul.nuts.vcr.v2.Revocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Verifiable Credential API spec
* API specification for common operations on Verifiable credentials. It allows the three roles, issuer, holder and verifier to issue, revoke, search, present and verify credentials.
*
* The version of the OpenAPI document: 2.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.vcr.v2;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Credential revocation record
*/
@ApiModel(description = "Credential revocation record")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:34.811142+02:00[Europe/Amsterdam]")
public class Revocation {
public static final String SERIALIZED_NAME_ISSUER = "issuer";
@SerializedName(SERIALIZED_NAME_ISSUER)
private String issuer;
public static final String SERIALIZED_NAME_SUBJECT = "subject";
@SerializedName(SERIALIZED_NAME_SUBJECT)
private String subject;
public static final String SERIALIZED_NAME_REASON = "reason";
@SerializedName(SERIALIZED_NAME_REASON)
private String reason;
public static final String SERIALIZED_NAME_DATE = "date";
@SerializedName(SERIALIZED_NAME_DATE)
private String date;
public static final String SERIALIZED_NAME_PROOF = "proof";
@SerializedName(SERIALIZED_NAME_PROOF)
private Object proof;
public Revocation issuer(String issuer) {
this.issuer = issuer;
return this;
}
/**
* DID according to Nuts specification
* @return issuer
**/
@ApiModelProperty(example = "did:nuts:B8PUHs2AUHbFF1xLLK4eZjgErEcMXHxs68FteY7NDtCY", required = true, value = "DID according to Nuts specification")
public String getIssuer() {
return issuer;
}
public void setIssuer(String issuer) {
this.issuer = issuer;
}
public Revocation subject(String subject) {
this.subject = subject;
return this;
}
/**
* subject refers to the credential identifier that is revoked (not the credential subject)
* @return subject
**/
@ApiModelProperty(required = true, value = "subject refers to the credential identifier that is revoked (not the credential subject)")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public Revocation reason(String reason) {
this.reason = reason;
return this;
}
/**
* reason describes why the VC has been revoked
* @return reason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "reason describes why the VC has been revoked")
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public Revocation date(String date) {
this.date = date;
return this;
}
/**
* date is a rfc3339 formatted datetime.
* @return date
**/
@ApiModelProperty(required = true, value = "date is a rfc3339 formatted datetime.")
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public Revocation proof(Object proof) {
this.proof = proof;
return this;
}
/**
* Proof contains the cryptographic proof(s).
* @return proof
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Proof contains the cryptographic proof(s).")
public Object getProof() {
return proof;
}
public void setProof(Object proof) {
this.proof = proof;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Revocation revocation = (Revocation) o;
return Objects.equals(this.issuer, revocation.issuer) &&
Objects.equals(this.subject, revocation.subject) &&
Objects.equals(this.reason, revocation.reason) &&
Objects.equals(this.date, revocation.date) &&
Objects.equals(this.proof, revocation.proof);
}
@Override
public int hashCode() {
return Objects.hash(issuer, subject, reason, date, proof);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Revocation {\n");
sb.append(" issuer: ").append(toIndentedString(issuer)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" proof: ").append(toIndentedString(proof)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy