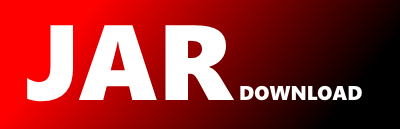
nl.reinkrul.nuts.vdr.DIDCreateRequest Maven / Gradle / Ivy
/*
* Nuts Verifiable Data Registry API spec
* API specification for the Verifiable Data Registry
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.vdr;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* DIDCreateRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-09-14T14:56:34.319584+02:00[Europe/Amsterdam]")
public class DIDCreateRequest {
public static final String SERIALIZED_NAME_CONTROLLERS = "controllers";
@SerializedName(SERIALIZED_NAME_CONTROLLERS)
private List controllers = null;
public static final String SERIALIZED_NAME_ASSERTION_METHOD = "assertionMethod";
@SerializedName(SERIALIZED_NAME_ASSERTION_METHOD)
private Boolean assertionMethod = true;
public static final String SERIALIZED_NAME_AUTHENTICATION = "authentication";
@SerializedName(SERIALIZED_NAME_AUTHENTICATION)
private Boolean authentication = false;
public static final String SERIALIZED_NAME_CAPABILITY_INVOCATION = "capabilityInvocation";
@SerializedName(SERIALIZED_NAME_CAPABILITY_INVOCATION)
private Boolean capabilityInvocation;
public static final String SERIALIZED_NAME_CAPABILITY_DELEGATION = "capabilityDelegation";
@SerializedName(SERIALIZED_NAME_CAPABILITY_DELEGATION)
private Boolean capabilityDelegation = true;
public static final String SERIALIZED_NAME_KEY_AGREEMENT = "keyAgreement";
@SerializedName(SERIALIZED_NAME_KEY_AGREEMENT)
private Boolean keyAgreement = false;
public static final String SERIALIZED_NAME_SELF_CONTROL = "selfControl";
@SerializedName(SERIALIZED_NAME_SELF_CONTROL)
private Boolean selfControl = true;
public DIDCreateRequest controllers(List controllers) {
this.controllers = controllers;
return this;
}
public DIDCreateRequest addControllersItem(String controllersItem) {
if (this.controllers == null) {
this.controllers = new ArrayList();
}
this.controllers.add(controllersItem);
return this;
}
/**
* List of DIDs that can control the new DID Document. If selfControl = true and controllers is not empty, the newly generated DID will be added to the list of controllers.
* @return controllers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of DIDs that can control the new DID Document. If selfControl = true and controllers is not empty, the newly generated DID will be added to the list of controllers. ")
public List getControllers() {
return controllers;
}
public void setControllers(List controllers) {
this.controllers = controllers;
}
public DIDCreateRequest assertionMethod(Boolean assertionMethod) {
this.assertionMethod = assertionMethod;
return this;
}
/**
* indicates if the generated key pair can be used for assertions.
* @return assertionMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "indicates if the generated key pair can be used for assertions.")
public Boolean getAssertionMethod() {
return assertionMethod;
}
public void setAssertionMethod(Boolean assertionMethod) {
this.assertionMethod = assertionMethod;
}
public DIDCreateRequest authentication(Boolean authentication) {
this.authentication = authentication;
return this;
}
/**
* indicates if the generated key pair can be used for authentication.
* @return authentication
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "indicates if the generated key pair can be used for authentication.")
public Boolean getAuthentication() {
return authentication;
}
public void setAuthentication(Boolean authentication) {
this.authentication = authentication;
}
public DIDCreateRequest capabilityInvocation(Boolean capabilityInvocation) {
this.capabilityInvocation = capabilityInvocation;
return this;
}
/**
* indicates if the generated key pair can be used for altering DID Documents. In combination with selfControl = true, the key can be used to alter the new DID Document. Defaults to true when not given. default: true
* @return capabilityInvocation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "indicates if the generated key pair can be used for altering DID Documents. In combination with selfControl = true, the key can be used to alter the new DID Document. Defaults to true when not given. default: true ")
public Boolean getCapabilityInvocation() {
return capabilityInvocation;
}
public void setCapabilityInvocation(Boolean capabilityInvocation) {
this.capabilityInvocation = capabilityInvocation;
}
public DIDCreateRequest capabilityDelegation(Boolean capabilityDelegation) {
this.capabilityDelegation = capabilityDelegation;
return this;
}
/**
* indicates if the generated key pair can be used for capability delegations.
* @return capabilityDelegation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "indicates if the generated key pair can be used for capability delegations.")
public Boolean getCapabilityDelegation() {
return capabilityDelegation;
}
public void setCapabilityDelegation(Boolean capabilityDelegation) {
this.capabilityDelegation = capabilityDelegation;
}
public DIDCreateRequest keyAgreement(Boolean keyAgreement) {
this.keyAgreement = keyAgreement;
return this;
}
/**
* indicates if the generated key pair can be used for Key agreements.
* @return keyAgreement
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "indicates if the generated key pair can be used for Key agreements.")
public Boolean getKeyAgreement() {
return keyAgreement;
}
public void setKeyAgreement(Boolean keyAgreement) {
this.keyAgreement = keyAgreement;
}
public DIDCreateRequest selfControl(Boolean selfControl) {
this.selfControl = selfControl;
return this;
}
/**
* whether the generated DID Document can be altered with its own capabilityInvocation key.
* @return selfControl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "whether the generated DID Document can be altered with its own capabilityInvocation key.")
public Boolean getSelfControl() {
return selfControl;
}
public void setSelfControl(Boolean selfControl) {
this.selfControl = selfControl;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DIDCreateRequest diDCreateRequest = (DIDCreateRequest) o;
return Objects.equals(this.controllers, diDCreateRequest.controllers) &&
Objects.equals(this.assertionMethod, diDCreateRequest.assertionMethod) &&
Objects.equals(this.authentication, diDCreateRequest.authentication) &&
Objects.equals(this.capabilityInvocation, diDCreateRequest.capabilityInvocation) &&
Objects.equals(this.capabilityDelegation, diDCreateRequest.capabilityDelegation) &&
Objects.equals(this.keyAgreement, diDCreateRequest.keyAgreement) &&
Objects.equals(this.selfControl, diDCreateRequest.selfControl);
}
@Override
public int hashCode() {
return Objects.hash(controllers, assertionMethod, authentication, capabilityInvocation, capabilityDelegation, keyAgreement, selfControl);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DIDCreateRequest {\n");
sb.append(" controllers: ").append(toIndentedString(controllers)).append("\n");
sb.append(" assertionMethod: ").append(toIndentedString(assertionMethod)).append("\n");
sb.append(" authentication: ").append(toIndentedString(authentication)).append("\n");
sb.append(" capabilityInvocation: ").append(toIndentedString(capabilityInvocation)).append("\n");
sb.append(" capabilityDelegation: ").append(toIndentedString(capabilityDelegation)).append("\n");
sb.append(" keyAgreement: ").append(toIndentedString(keyAgreement)).append("\n");
sb.append(" selfControl: ").append(toIndentedString(selfControl)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy