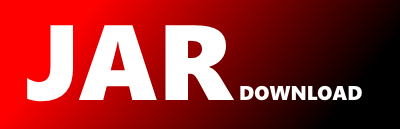
nl.reinkrul.nuts.auth.TokenIntrospectionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Auth Service API spec
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.auth;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* Token introspection response as described in RFC7662 section 2.2
*/
@JsonPropertyOrder({
TokenIntrospectionResponse.JSON_PROPERTY_ACTIVE,
TokenIntrospectionResponse.JSON_PROPERTY_SERVICE,
TokenIntrospectionResponse.JSON_PROPERTY_ISS,
TokenIntrospectionResponse.JSON_PROPERTY_SUB,
TokenIntrospectionResponse.JSON_PROPERTY_AUD,
TokenIntrospectionResponse.JSON_PROPERTY_VCS,
TokenIntrospectionResponse.JSON_PROPERTY_RESOLVED_V_CS,
TokenIntrospectionResponse.JSON_PROPERTY_OSI,
TokenIntrospectionResponse.JSON_PROPERTY_EXP,
TokenIntrospectionResponse.JSON_PROPERTY_IAT,
TokenIntrospectionResponse.JSON_PROPERTY_FAMILY_NAME,
TokenIntrospectionResponse.JSON_PROPERTY_PREFIX,
TokenIntrospectionResponse.JSON_PROPERTY_INITIALS,
TokenIntrospectionResponse.JSON_PROPERTY_EMAIL,
TokenIntrospectionResponse.JSON_PROPERTY_USERNAME,
TokenIntrospectionResponse.JSON_PROPERTY_ASSURANCE_LEVEL,
TokenIntrospectionResponse.JSON_PROPERTY_USER_ROLE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-05-12T15:13:45.138986+02:00[Europe/Amsterdam]")
public class TokenIntrospectionResponse {
public static final String JSON_PROPERTY_ACTIVE = "active";
private Boolean active;
public static final String JSON_PROPERTY_SERVICE = "service";
private String service;
public static final String JSON_PROPERTY_ISS = "iss";
private String iss;
public static final String JSON_PROPERTY_SUB = "sub";
private String sub;
public static final String JSON_PROPERTY_AUD = "aud";
private String aud;
public static final String JSON_PROPERTY_VCS = "vcs";
private List vcs;
public static final String JSON_PROPERTY_RESOLVED_V_CS = "resolvedVCs";
private List resolvedVCs;
public static final String JSON_PROPERTY_OSI = "osi";
private String osi;
public static final String JSON_PROPERTY_EXP = "exp";
private Integer exp;
public static final String JSON_PROPERTY_IAT = "iat";
private Integer iat;
public static final String JSON_PROPERTY_FAMILY_NAME = "family_name";
private String familyName;
public static final String JSON_PROPERTY_PREFIX = "prefix";
private String prefix;
public static final String JSON_PROPERTY_INITIALS = "initials";
private String initials;
public static final String JSON_PROPERTY_EMAIL = "email";
private String email;
public static final String JSON_PROPERTY_USERNAME = "username";
private String username;
/**
* Assurance level of the identity of the End-User.
*/
public enum AssuranceLevelEnum {
LOW("low"),
SUBSTANTIAL("substantial"),
HIGH("high");
private String value;
AssuranceLevelEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AssuranceLevelEnum fromValue(String value) {
for (AssuranceLevelEnum b : AssuranceLevelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_ASSURANCE_LEVEL = "assurance_level";
private AssuranceLevelEnum assuranceLevel;
public static final String JSON_PROPERTY_USER_ROLE = "user_role";
private String userRole;
public TokenIntrospectionResponse() {
}
public TokenIntrospectionResponse active(Boolean active) {
this.active = active;
return this;
}
/**
* True if the token is active, false if the token is expired, malformed etc.
* @return active
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ACTIVE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getActive() {
return active;
}
@JsonProperty(JSON_PROPERTY_ACTIVE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setActive(Boolean active) {
this.active = active;
}
public TokenIntrospectionResponse service(String service) {
this.service = service;
return this;
}
/**
* Get service
* @return service
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SERVICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getService() {
return service;
}
@JsonProperty(JSON_PROPERTY_SERVICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setService(String service) {
this.service = service;
}
public TokenIntrospectionResponse iss(String iss) {
this.iss = iss;
return this;
}
/**
* The subject (not a Nuts subject) contains the DID of the authorizer.
* @return iss
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ISS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIss() {
return iss;
}
@JsonProperty(JSON_PROPERTY_ISS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIss(String iss) {
this.iss = iss;
}
public TokenIntrospectionResponse sub(String sub) {
this.sub = sub;
return this;
}
/**
* The subject is always the acting party, thus the care organization requesting access to data.
* @return sub
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SUB)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSub() {
return sub;
}
@JsonProperty(JSON_PROPERTY_SUB)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSub(String sub) {
this.sub = sub;
}
public TokenIntrospectionResponse aud(String aud) {
this.aud = aud;
return this;
}
/**
* As per rfc7523 https://tools.ietf.org/html/rfc7523>, the aud must be the token endpoint. This can be taken from the Nuts registry.
* @return aud
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AUD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAud() {
return aud;
}
@JsonProperty(JSON_PROPERTY_AUD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAud(String aud) {
this.aud = aud;
}
public TokenIntrospectionResponse vcs(List vcs) {
this.vcs = vcs;
return this;
}
public TokenIntrospectionResponse addVcsItem(String vcsItem) {
if (this.vcs == null) {
this.vcs = new ArrayList<>();
}
this.vcs.add(vcsItem);
return this;
}
/**
* Get vcs
* @return vcs
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_VCS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getVcs() {
return vcs;
}
@JsonProperty(JSON_PROPERTY_VCS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVcs(List vcs) {
this.vcs = vcs;
}
public TokenIntrospectionResponse resolvedVCs(List resolvedVCs) {
this.resolvedVCs = resolvedVCs;
return this;
}
public TokenIntrospectionResponse addResolvedVCsItem(nl.reinkrul.nuts.common.VerifiableCredential resolvedVCsItem) {
if (this.resolvedVCs == null) {
this.resolvedVCs = new ArrayList<>();
}
this.resolvedVCs.add(resolvedVCsItem);
return this;
}
/**
* credentials resolved from `vcs` (VC IDs). It contains only those VCs that could be resolved.
* @return resolvedVCs
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RESOLVED_V_CS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getResolvedVCs() {
return resolvedVCs;
}
@JsonProperty(JSON_PROPERTY_RESOLVED_V_CS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResolvedVCs(List resolvedVCs) {
this.resolvedVCs = resolvedVCs;
}
public TokenIntrospectionResponse osi(String osi) {
this.osi = osi;
return this;
}
/**
* encoded ops signature. (TBD)
* @return osi
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OSI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOsi() {
return osi;
}
@JsonProperty(JSON_PROPERTY_OSI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOsi(String osi) {
this.osi = osi;
}
public TokenIntrospectionResponse exp(Integer exp) {
this.exp = exp;
return this;
}
/**
* Get exp
* @return exp
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EXP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getExp() {
return exp;
}
@JsonProperty(JSON_PROPERTY_EXP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExp(Integer exp) {
this.exp = exp;
}
public TokenIntrospectionResponse iat(Integer iat) {
this.iat = iat;
return this;
}
/**
* Get iat
* @return iat
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getIat() {
return iat;
}
@JsonProperty(JSON_PROPERTY_IAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIat(Integer iat) {
this.iat = iat;
}
public TokenIntrospectionResponse familyName(String familyName) {
this.familyName = familyName;
return this;
}
/**
* Surname(s) or last name(s) of the End-User.
* @return familyName
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FAMILY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFamilyName() {
return familyName;
}
@JsonProperty(JSON_PROPERTY_FAMILY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFamilyName(String familyName) {
this.familyName = familyName;
}
public TokenIntrospectionResponse prefix(String prefix) {
this.prefix = prefix;
return this;
}
/**
* Surname prefix
* @return prefix
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PREFIX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPrefix() {
return prefix;
}
@JsonProperty(JSON_PROPERTY_PREFIX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPrefix(String prefix) {
this.prefix = prefix;
}
public TokenIntrospectionResponse initials(String initials) {
this.initials = initials;
return this;
}
/**
* Initials of the End-User.
* @return initials
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_INITIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getInitials() {
return initials;
}
@JsonProperty(JSON_PROPERTY_INITIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInitials(String initials) {
this.initials = initials;
}
public TokenIntrospectionResponse email(String email) {
this.email = email;
return this;
}
/**
* End-User's preferred e-mail address. Should be a personal email and can be used to uniquely identify a user. Just like the email used for an account.
* @return email
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EMAIL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEmail() {
return email;
}
@JsonProperty(JSON_PROPERTY_EMAIL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEmail(String email) {
this.email = email;
}
public TokenIntrospectionResponse username(String username) {
this.username = username;
return this;
}
/**
* Identifier uniquely identifying the End-User's account in the issuing system.
* @return username
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUsername() {
return username;
}
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUsername(String username) {
this.username = username;
}
public TokenIntrospectionResponse assuranceLevel(AssuranceLevelEnum assuranceLevel) {
this.assuranceLevel = assuranceLevel;
return this;
}
/**
* Assurance level of the identity of the End-User.
* @return assuranceLevel
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ASSURANCE_LEVEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AssuranceLevelEnum getAssuranceLevel() {
return assuranceLevel;
}
@JsonProperty(JSON_PROPERTY_ASSURANCE_LEVEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAssuranceLevel(AssuranceLevelEnum assuranceLevel) {
this.assuranceLevel = assuranceLevel;
}
public TokenIntrospectionResponse userRole(String userRole) {
this.userRole = userRole;
return this;
}
/**
* Role of the End-User.
* @return userRole
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_USER_ROLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUserRole() {
return userRole;
}
@JsonProperty(JSON_PROPERTY_USER_ROLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserRole(String userRole) {
this.userRole = userRole;
}
/**
* Return true if this TokenIntrospectionResponse object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TokenIntrospectionResponse tokenIntrospectionResponse = (TokenIntrospectionResponse) o;
return Objects.equals(this.active, tokenIntrospectionResponse.active) &&
Objects.equals(this.service, tokenIntrospectionResponse.service) &&
Objects.equals(this.iss, tokenIntrospectionResponse.iss) &&
Objects.equals(this.sub, tokenIntrospectionResponse.sub) &&
Objects.equals(this.aud, tokenIntrospectionResponse.aud) &&
Objects.equals(this.vcs, tokenIntrospectionResponse.vcs) &&
Objects.equals(this.resolvedVCs, tokenIntrospectionResponse.resolvedVCs) &&
Objects.equals(this.osi, tokenIntrospectionResponse.osi) &&
Objects.equals(this.exp, tokenIntrospectionResponse.exp) &&
Objects.equals(this.iat, tokenIntrospectionResponse.iat) &&
Objects.equals(this.familyName, tokenIntrospectionResponse.familyName) &&
Objects.equals(this.prefix, tokenIntrospectionResponse.prefix) &&
Objects.equals(this.initials, tokenIntrospectionResponse.initials) &&
Objects.equals(this.email, tokenIntrospectionResponse.email) &&
Objects.equals(this.username, tokenIntrospectionResponse.username) &&
Objects.equals(this.assuranceLevel, tokenIntrospectionResponse.assuranceLevel) &&
Objects.equals(this.userRole, tokenIntrospectionResponse.userRole);
}
@Override
public int hashCode() {
return Objects.hash(active, service, iss, sub, aud, vcs, resolvedVCs, osi, exp, iat, familyName, prefix, initials, email, username, assuranceLevel, userRole);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TokenIntrospectionResponse {\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" service: ").append(toIndentedString(service)).append("\n");
sb.append(" iss: ").append(toIndentedString(iss)).append("\n");
sb.append(" sub: ").append(toIndentedString(sub)).append("\n");
sb.append(" aud: ").append(toIndentedString(aud)).append("\n");
sb.append(" vcs: ").append(toIndentedString(vcs)).append("\n");
sb.append(" resolvedVCs: ").append(toIndentedString(resolvedVCs)).append("\n");
sb.append(" osi: ").append(toIndentedString(osi)).append("\n");
sb.append(" exp: ").append(toIndentedString(exp)).append("\n");
sb.append(" iat: ").append(toIndentedString(iat)).append("\n");
sb.append(" familyName: ").append(toIndentedString(familyName)).append("\n");
sb.append(" prefix: ").append(toIndentedString(prefix)).append("\n");
sb.append(" initials: ").append(toIndentedString(initials)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" assuranceLevel: ").append(toIndentedString(assuranceLevel)).append("\n");
sb.append(" userRole: ").append(toIndentedString(userRole)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `active` to the URL query string
if (getActive() != null) {
joiner.add(String.format("%sactive%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getActive()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `service` to the URL query string
if (getService() != null) {
joiner.add(String.format("%sservice%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getService()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `iss` to the URL query string
if (getIss() != null) {
joiner.add(String.format("%siss%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIss()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `sub` to the URL query string
if (getSub() != null) {
joiner.add(String.format("%ssub%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSub()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `aud` to the URL query string
if (getAud() != null) {
joiner.add(String.format("%saud%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAud()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `vcs` to the URL query string
if (getVcs() != null) {
for (int i = 0; i < getVcs().size(); i++) {
joiner.add(String.format("%svcs%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getVcs().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `resolvedVCs` to the URL query string
if (getResolvedVCs() != null) {
for (int i = 0; i < getResolvedVCs().size(); i++) {
if (getResolvedVCs().get(i) != null) {
joiner.add(getResolvedVCs().get(i).toUrlQueryString(String.format("%sresolvedVCs%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `osi` to the URL query string
if (getOsi() != null) {
joiner.add(String.format("%sosi%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOsi()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `exp` to the URL query string
if (getExp() != null) {
joiner.add(String.format("%sexp%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExp()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `iat` to the URL query string
if (getIat() != null) {
joiner.add(String.format("%siat%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIat()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `family_name` to the URL query string
if (getFamilyName() != null) {
joiner.add(String.format("%sfamily_name%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFamilyName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `prefix` to the URL query string
if (getPrefix() != null) {
joiner.add(String.format("%sprefix%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getPrefix()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `initials` to the URL query string
if (getInitials() != null) {
joiner.add(String.format("%sinitials%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getInitials()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `email` to the URL query string
if (getEmail() != null) {
joiner.add(String.format("%semail%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getEmail()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `username` to the URL query string
if (getUsername() != null) {
joiner.add(String.format("%susername%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getUsername()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `assurance_level` to the URL query string
if (getAssuranceLevel() != null) {
joiner.add(String.format("%sassurance_level%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAssuranceLevel()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `user_role` to the URL query string
if (getUserRole() != null) {
joiner.add(String.format("%suser_role%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getUserRole()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy