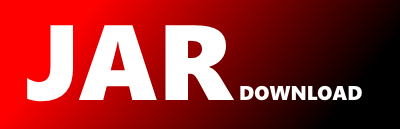
nl.reinkrul.nuts.crypto.CryptoApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Crypto Service API spec
* API specification for crypto services available within nuts node
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.crypto;
import nl.reinkrul.nuts.ApiClient;
import nl.reinkrul.nuts.ApiException;
import nl.reinkrul.nuts.ApiResponse;
import nl.reinkrul.nuts.Pair;
import nl.reinkrul.nuts.crypto.DecryptJwe200Response;
import nl.reinkrul.nuts.crypto.DecryptJweRequest;
import nl.reinkrul.nuts.crypto.EncryptJweRequest;
import nl.reinkrul.nuts.crypto.SignJwsRequest;
import nl.reinkrul.nuts.crypto.SignJwtDefaultResponse;
import nl.reinkrul.nuts.crypto.SignJwtRequest;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-05-12T15:13:44.888654+02:00[Europe/Amsterdam]")
public class CryptoApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public CryptoApi() {
this(new ApiClient());
}
public CryptoApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Decrypt a payload with the private key related to the KeyID in the header
* Decrypt a payload with the private key related to the KeyID in the header Note: this feature is experimental and might be changed in a future minor release without prior notice. error returns: * 400 - incorrect input
* @param decryptJweRequest (required)
* @return DecryptJwe200Response
* @throws ApiException if fails to make API call
*/
public DecryptJwe200Response decryptJwe(DecryptJweRequest decryptJweRequest) throws ApiException {
ApiResponse localVarResponse = decryptJweWithHttpInfo(decryptJweRequest);
return localVarResponse.getData();
}
/**
* Decrypt a payload with the private key related to the KeyID in the header
* Decrypt a payload with the private key related to the KeyID in the header Note: this feature is experimental and might be changed in a future minor release without prior notice. error returns: * 400 - incorrect input
* @param decryptJweRequest (required)
* @return ApiResponse<DecryptJwe200Response>
* @throws ApiException if fails to make API call
*/
public ApiResponse decryptJweWithHttpInfo(DecryptJweRequest decryptJweRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = decryptJweRequestBuilder(decryptJweRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("decryptJwe", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder decryptJweRequestBuilder(DecryptJweRequest decryptJweRequest) throws ApiException {
// verify the required parameter 'decryptJweRequest' is set
if (decryptJweRequest == null) {
throw new ApiException(400, "Missing the required parameter 'decryptJweRequest' when calling decryptJwe");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/crypto/v1/decrypt_jwe";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(decryptJweRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Encrypt a payload and headers with the public key of the given DID into a JWE object
* Encrypt a payload and headers with the public key of the given DID into a JWE object Note: this feature is experimental and might be changed in a future minor release without prior notice. error returns: * 400 - incorrect input
* @param encryptJweRequest (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String encryptJwe(EncryptJweRequest encryptJweRequest) throws ApiException {
ApiResponse localVarResponse = encryptJweWithHttpInfo(encryptJweRequest);
return localVarResponse.getData();
}
/**
* Encrypt a payload and headers with the public key of the given DID into a JWE object
* Encrypt a payload and headers with the public key of the given DID into a JWE object Note: this feature is experimental and might be changed in a future minor release without prior notice. error returns: * 400 - incorrect input
* @param encryptJweRequest (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse encryptJweWithHttpInfo(EncryptJweRequest encryptJweRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = encryptJweRequestBuilder(encryptJweRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("encryptJwe", localVarResponse);
}
// for plain text response
if (localVarResponse.headers().map().containsKey("Content-Type") &&
"text/plain".equalsIgnoreCase(localVarResponse.headers().map().get("Content-Type").get(0))) {
java.util.Scanner s = new java.util.Scanner(localVarResponse.body()).useDelimiter("\\A");
String responseBodyText = s.hasNext() ? s.next() : "";
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
responseBodyText
);
} else {
throw new RuntimeException("Error! The response Content-Type is supposed to be `text/plain` but it's not: " + localVarResponse);
}
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder encryptJweRequestBuilder(EncryptJweRequest encryptJweRequest) throws ApiException {
// verify the required parameter 'encryptJweRequest' is set
if (encryptJweRequest == null) {
throw new ApiException(400, "Missing the required parameter 'encryptJweRequest' when calling encryptJwe");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/crypto/v1/encrypt_jwe";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "text/plain, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(encryptJweRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* sign a payload and headers with the private key of the given kid into a JWS object
* Sign a payload and headers with the private key of the given kid into a JWS object error returns: * 400 - incorrect input
* @param signJwsRequest (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String signJws(SignJwsRequest signJwsRequest) throws ApiException {
ApiResponse localVarResponse = signJwsWithHttpInfo(signJwsRequest);
return localVarResponse.getData();
}
/**
* sign a payload and headers with the private key of the given kid into a JWS object
* Sign a payload and headers with the private key of the given kid into a JWS object error returns: * 400 - incorrect input
* @param signJwsRequest (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse signJwsWithHttpInfo(SignJwsRequest signJwsRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = signJwsRequestBuilder(signJwsRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("signJws", localVarResponse);
}
// for plain text response
if (localVarResponse.headers().map().containsKey("Content-Type") &&
"text/plain".equalsIgnoreCase(localVarResponse.headers().map().get("Content-Type").get(0))) {
java.util.Scanner s = new java.util.Scanner(localVarResponse.body()).useDelimiter("\\A");
String responseBodyText = s.hasNext() ? s.next() : "";
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
responseBodyText
);
} else {
throw new RuntimeException("Error! The response Content-Type is supposed to be `text/plain` but it's not: " + localVarResponse);
}
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder signJwsRequestBuilder(SignJwsRequest signJwsRequest) throws ApiException {
// verify the required parameter 'signJwsRequest' is set
if (signJwsRequest == null) {
throw new ApiException(400, "Missing the required parameter 'signJwsRequest' when calling signJws");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/crypto/v1/sign_jws";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "text/plain, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(signJwsRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* sign a JWT payload with the private key of the given kid
* Sign a JWT payload with the private key of the given kid error returns: * 400 - incorrect input
* @param signJwtRequest (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String signJwt(SignJwtRequest signJwtRequest) throws ApiException {
ApiResponse localVarResponse = signJwtWithHttpInfo(signJwtRequest);
return localVarResponse.getData();
}
/**
* sign a JWT payload with the private key of the given kid
* Sign a JWT payload with the private key of the given kid error returns: * 400 - incorrect input
* @param signJwtRequest (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse signJwtWithHttpInfo(SignJwtRequest signJwtRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = signJwtRequestBuilder(signJwtRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("signJwt", localVarResponse);
}
// for plain text response
if (localVarResponse.headers().map().containsKey("Content-Type") &&
"text/plain".equalsIgnoreCase(localVarResponse.headers().map().get("Content-Type").get(0))) {
java.util.Scanner s = new java.util.Scanner(localVarResponse.body()).useDelimiter("\\A");
String responseBodyText = s.hasNext() ? s.next() : "";
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
responseBodyText
);
} else {
throw new RuntimeException("Error! The response Content-Type is supposed to be `text/plain` but it's not: " + localVarResponse);
}
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder signJwtRequestBuilder(SignJwtRequest signJwtRequest) throws ApiException {
// verify the required parameter 'signJwtRequest' is set
if (signJwtRequest == null) {
throw new ApiException(400, "Missing the required parameter 'signJwtRequest' when calling signJwt");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/crypto/v1/sign_jwt";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "text/plain, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(signJwtRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy