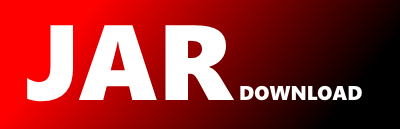
nl.reinkrul.nuts.didman.ServicesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts DID Manager API spec
* API specification for DID management helper APIs. The goal of this API is to help administrative interfaces to manage DIDs.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.didman;
import nl.reinkrul.nuts.ApiClient;
import nl.reinkrul.nuts.ApiException;
import nl.reinkrul.nuts.ApiResponse;
import nl.reinkrul.nuts.Pair;
import nl.reinkrul.nuts.didman.CompoundService;
import nl.reinkrul.nuts.didman.CompoundServiceProperties;
import nl.reinkrul.nuts.didman.Endpoint;
import nl.reinkrul.nuts.didman.EndpointProperties;
import nl.reinkrul.nuts.didman.EndpointResponse;
import nl.reinkrul.nuts.didman.GetContactInformationDefaultResponse;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-05-12T15:13:44.240661+02:00[Europe/Amsterdam]")
public class ServicesApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public ServicesApi() {
this(new ApiClient());
}
public ServicesApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Add a compound service to a DID Document.
* Add a service to a DID Document that references one or more endpoints using a map. The keys of the map indicate the service name/type, the values contain the references to the endpoints. The endpoints may be in the same or in another DID Document, but they must be resolvable when the service is created. The references must follow the format for service references as specified by Nuts RFC006. This API will also check if an endpoint with the same type already exists. This API is not meant to add endpoints. error returns: * 400 - incorrect input * 404 - unknown DID * 409 - a service with the same type already exists
* @param did URL encoded DID. (required)
* @param compoundServiceProperties (required)
* @return CompoundService
* @throws ApiException if fails to make API call
*/
public CompoundService addCompoundService(String did, CompoundServiceProperties compoundServiceProperties) throws ApiException {
ApiResponse localVarResponse = addCompoundServiceWithHttpInfo(did, compoundServiceProperties);
return localVarResponse.getData();
}
/**
* Add a compound service to a DID Document.
* Add a service to a DID Document that references one or more endpoints using a map. The keys of the map indicate the service name/type, the values contain the references to the endpoints. The endpoints may be in the same or in another DID Document, but they must be resolvable when the service is created. The references must follow the format for service references as specified by Nuts RFC006. This API will also check if an endpoint with the same type already exists. This API is not meant to add endpoints. error returns: * 400 - incorrect input * 404 - unknown DID * 409 - a service with the same type already exists
* @param did URL encoded DID. (required)
* @param compoundServiceProperties (required)
* @return ApiResponse<CompoundService>
* @throws ApiException if fails to make API call
*/
public ApiResponse addCompoundServiceWithHttpInfo(String did, CompoundServiceProperties compoundServiceProperties) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addCompoundServiceRequestBuilder(did, compoundServiceProperties);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addCompoundService", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder addCompoundServiceRequestBuilder(String did, CompoundServiceProperties compoundServiceProperties) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling addCompoundService");
}
// verify the required parameter 'compoundServiceProperties' is set
if (compoundServiceProperties == null) {
throw new ApiException(400, "Missing the required parameter 'compoundServiceProperties' when calling addCompoundService");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/compoundservice"
.replace("{did}", ApiClient.urlEncode(did.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(compoundServiceProperties);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Add a service endpoint or a reference to a service.
* To distinguish from compound services, this type of service will be called an endpoint. In the Nuts specs this type of service is called a concrete service. Add an endpoint with a type and URL to a DID Service in a Document. The API will convert it to a DID service with the serviceEndpoint set to a URL. This API will also check if an endpoint with the same type already exists. This API is not meant to add compound services. The URL can either be an Endpoint or a reference to another service. error returns: * 400 - incorrect input * 404 - unknown DID * 409 - a service with the same type already exists
* @param did URL encoded DID. (required)
* @param endpointProperties (required)
* @return Endpoint
* @throws ApiException if fails to make API call
*/
public Endpoint addEndpoint(String did, EndpointProperties endpointProperties) throws ApiException {
ApiResponse localVarResponse = addEndpointWithHttpInfo(did, endpointProperties);
return localVarResponse.getData();
}
/**
* Add a service endpoint or a reference to a service.
* To distinguish from compound services, this type of service will be called an endpoint. In the Nuts specs this type of service is called a concrete service. Add an endpoint with a type and URL to a DID Service in a Document. The API will convert it to a DID service with the serviceEndpoint set to a URL. This API will also check if an endpoint with the same type already exists. This API is not meant to add compound services. The URL can either be an Endpoint or a reference to another service. error returns: * 400 - incorrect input * 404 - unknown DID * 409 - a service with the same type already exists
* @param did URL encoded DID. (required)
* @param endpointProperties (required)
* @return ApiResponse<Endpoint>
* @throws ApiException if fails to make API call
*/
public ApiResponse addEndpointWithHttpInfo(String did, EndpointProperties endpointProperties) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addEndpointRequestBuilder(did, endpointProperties);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addEndpoint", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder addEndpointRequestBuilder(String did, EndpointProperties endpointProperties) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling addEndpoint");
}
// verify the required parameter 'endpointProperties' is set
if (endpointProperties == null) {
throw new ApiException(400, "Missing the required parameter 'endpointProperties' when calling addEndpoint");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/endpoint"
.replace("{did}", ApiClient.urlEncode(did.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(endpointProperties);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Delete all endpoints with the provided type from the DID Document. error returns: * 400 - malformatted input, like the DID or the endpoint type. * 404 - DID or service with this type not found. * 409 - the service is referenced by other services
* @param did URL encoded DID. (required)
* @param type Type of the service (required)
* @throws ApiException if fails to make API call
*/
public void deleteEndpointsByType(String did, String type) throws ApiException {
deleteEndpointsByTypeWithHttpInfo(did, type);
}
/**
*
* Delete all endpoints with the provided type from the DID Document. error returns: * 400 - malformatted input, like the DID or the endpoint type. * 404 - DID or service with this type not found. * 409 - the service is referenced by other services
* @param did URL encoded DID. (required)
* @param type Type of the service (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteEndpointsByTypeWithHttpInfo(String did, String type) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteEndpointsByTypeRequestBuilder(did, type);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteEndpointsByType", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteEndpointsByTypeRequestBuilder(String did, String type) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling deleteEndpointsByType");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(400, "Missing the required parameter 'type' when calling deleteEndpointsByType");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/endpoint/{type}"
.replace("{did}", ApiClient.urlEncode(did.toString()))
.replace("{type}", ApiClient.urlEncode(type.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/problem+json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Remove a service from a DID Document.
* Remove a service from a DID Document. error returns: * 400 - incorrect input * 404 - unknown DID * 409 - the service is referenced by other services
* @param id URL encoded service ID. (required)
* @throws ApiException if fails to make API call
*/
public void deleteService(String id) throws ApiException {
deleteServiceWithHttpInfo(id);
}
/**
* Remove a service from a DID Document.
* Remove a service from a DID Document. error returns: * 400 - incorrect input * 404 - unknown DID * 409 - the service is referenced by other services
* @param id URL encoded service ID. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteServiceWithHttpInfo(String id) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteServiceRequestBuilder(id);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteService", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteServiceRequestBuilder(String id) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteService");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/service/{id}"
.replace("{id}", ApiClient.urlEncode(id.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/problem+json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Retrieves the endpoint with the specified endpointType from the specified compound service.
* Retrieves the endpoint with the specified endpointType from the specified compound service. It returns the serviceEndpoint of the specified service (which must be an absolute URL endpoint). error responses: * 400 - incorrect input (e.g. the given service type isn't a compound service) * 404 - unknown DID, compound service or endpoint * 406 - service references are nested too deep or reference is invalid in other ways
* @param did URL encoded DID. (required)
* @param compoundServiceType Service type of the compound service containing the endpoint to be resolved. (required)
* @param endpointType Entry in the compound service to be resolved as endpoint. (required)
* @param accept The requested return type, defaults to application/json. (optional)
* @param resolve Whether to resolve references. When true and the given endpoint is a reference it returns the endpoint of the referenced service. If false it returns the reference itself. Defaults to true. (optional)
* @return EndpointResponse
* @throws ApiException if fails to make API call
*/
public EndpointResponse getCompoundServiceEndpoint(String did, String compoundServiceType, String endpointType, String accept, Boolean resolve) throws ApiException {
ApiResponse localVarResponse = getCompoundServiceEndpointWithHttpInfo(did, compoundServiceType, endpointType, accept, resolve);
return localVarResponse.getData();
}
/**
* Retrieves the endpoint with the specified endpointType from the specified compound service.
* Retrieves the endpoint with the specified endpointType from the specified compound service. It returns the serviceEndpoint of the specified service (which must be an absolute URL endpoint). error responses: * 400 - incorrect input (e.g. the given service type isn't a compound service) * 404 - unknown DID, compound service or endpoint * 406 - service references are nested too deep or reference is invalid in other ways
* @param did URL encoded DID. (required)
* @param compoundServiceType Service type of the compound service containing the endpoint to be resolved. (required)
* @param endpointType Entry in the compound service to be resolved as endpoint. (required)
* @param accept The requested return type, defaults to application/json. (optional)
* @param resolve Whether to resolve references. When true and the given endpoint is a reference it returns the endpoint of the referenced service. If false it returns the reference itself. Defaults to true. (optional)
* @return ApiResponse<EndpointResponse>
* @throws ApiException if fails to make API call
*/
public ApiResponse getCompoundServiceEndpointWithHttpInfo(String did, String compoundServiceType, String endpointType, String accept, Boolean resolve) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getCompoundServiceEndpointRequestBuilder(did, compoundServiceType, endpointType, accept, resolve);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getCompoundServiceEndpoint", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getCompoundServiceEndpointRequestBuilder(String did, String compoundServiceType, String endpointType, String accept, Boolean resolve) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling getCompoundServiceEndpoint");
}
// verify the required parameter 'compoundServiceType' is set
if (compoundServiceType == null) {
throw new ApiException(400, "Missing the required parameter 'compoundServiceType' when calling getCompoundServiceEndpoint");
}
// verify the required parameter 'endpointType' is set
if (endpointType == null) {
throw new ApiException(400, "Missing the required parameter 'endpointType' when calling getCompoundServiceEndpoint");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/compoundservice/{compoundServiceType}/endpoint/{endpointType}"
.replace("{did}", ApiClient.urlEncode(did.toString()))
.replace("{compoundServiceType}", ApiClient.urlEncode(compoundServiceType.toString()))
.replace("{endpointType}", ApiClient.urlEncode(endpointType.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "resolve";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resolve", resolve));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
if (accept != null) {
localVarRequestBuilder.header("accept", accept.toString());
}
localVarRequestBuilder.header("Accept", "application/json, text/plain, application/problem+json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get a list of compound services for a DID document. error responses: * 400 - incorrect input * 404 - unknown DID
*
* @param did URL encoded DID. (required)
* @return List<CompoundService>
* @throws ApiException if fails to make API call
*/
public List getCompoundServices(String did) throws ApiException {
ApiResponse> localVarResponse = getCompoundServicesWithHttpInfo(did);
return localVarResponse.getData();
}
/**
* Get a list of compound services for a DID document. error responses: * 400 - incorrect input * 404 - unknown DID
*
* @param did URL encoded DID. (required)
* @return ApiResponse<List<CompoundService>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getCompoundServicesWithHttpInfo(String did) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getCompoundServicesRequestBuilder(did);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getCompoundServices", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getCompoundServicesRequestBuilder(String did) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling getCompoundServices");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/compoundservice"
.replace("{did}", ApiClient.urlEncode(did.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Update a compound service.
* Update a compound service in a DID Document. It follows the same requirements as when adding a compound service. It updates all endpoints of the compound service (no partial updates). Updating the type is not supported. error returns: * 400 - incorrect input * 404 - unknown DID or service with this type
* @param did URL encoded DID. (required)
* @param type Type of the compound service (required)
* @param compoundServiceProperties (required)
* @return CompoundService
* @throws ApiException if fails to make API call
*/
public CompoundService updateCompoundService(String did, String type, CompoundServiceProperties compoundServiceProperties) throws ApiException {
ApiResponse localVarResponse = updateCompoundServiceWithHttpInfo(did, type, compoundServiceProperties);
return localVarResponse.getData();
}
/**
* Update a compound service.
* Update a compound service in a DID Document. It follows the same requirements as when adding a compound service. It updates all endpoints of the compound service (no partial updates). Updating the type is not supported. error returns: * 400 - incorrect input * 404 - unknown DID or service with this type
* @param did URL encoded DID. (required)
* @param type Type of the compound service (required)
* @param compoundServiceProperties (required)
* @return ApiResponse<CompoundService>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateCompoundServiceWithHttpInfo(String did, String type, CompoundServiceProperties compoundServiceProperties) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateCompoundServiceRequestBuilder(did, type, compoundServiceProperties);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateCompoundService", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder updateCompoundServiceRequestBuilder(String did, String type, CompoundServiceProperties compoundServiceProperties) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling updateCompoundService");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(400, "Missing the required parameter 'type' when calling updateCompoundService");
}
// verify the required parameter 'compoundServiceProperties' is set
if (compoundServiceProperties == null) {
throw new ApiException(400, "Missing the required parameter 'compoundServiceProperties' when calling updateCompoundService");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/compoundservice/{type}"
.replace("{did}", ApiClient.urlEncode(did.toString()))
.replace("{type}", ApiClient.urlEncode(type.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(compoundServiceProperties);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Update a service endpoint or a reference to a service.
* Update an endpoint's URL or reference in a DID document. The endpoint to be updated is selected by type. The URL can either be an Endpoint or a reference to another service. Updating the type is not supported. error returns: * 400 - incorrect input * 404 - unknown DID or service with this type
* @param did URL encoded DID. (required)
* @param type Type of the service (required)
* @param endpointProperties (required)
* @return Endpoint
* @throws ApiException if fails to make API call
*/
public Endpoint updateEndpoint(String did, String type, EndpointProperties endpointProperties) throws ApiException {
ApiResponse localVarResponse = updateEndpointWithHttpInfo(did, type, endpointProperties);
return localVarResponse.getData();
}
/**
* Update a service endpoint or a reference to a service.
* Update an endpoint's URL or reference in a DID document. The endpoint to be updated is selected by type. The URL can either be an Endpoint or a reference to another service. Updating the type is not supported. error returns: * 400 - incorrect input * 404 - unknown DID or service with this type
* @param did URL encoded DID. (required)
* @param type Type of the service (required)
* @param endpointProperties (required)
* @return ApiResponse<Endpoint>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateEndpointWithHttpInfo(String did, String type, EndpointProperties endpointProperties) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateEndpointRequestBuilder(did, type, endpointProperties);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateEndpoint", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder updateEndpointRequestBuilder(String did, String type, EndpointProperties endpointProperties) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling updateEndpoint");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(400, "Missing the required parameter 'type' when calling updateEndpoint");
}
// verify the required parameter 'endpointProperties' is set
if (endpointProperties == null) {
throw new ApiException(400, "Missing the required parameter 'endpointProperties' when calling updateEndpoint");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/didman/v1/did/{did}/endpoint/{type}"
.replace("{did}", ApiClient.urlEncode(did.toString()))
.replace("{type}", ApiClient.urlEncode(type.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(endpointProperties);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy