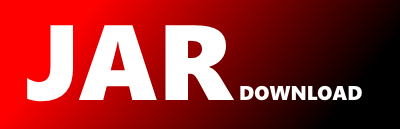
nl.reinkrul.nuts.vdr.DidApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Verifiable Data Registry API spec
* API specification for the Verifiable Data Registry
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.vdr;
import nl.reinkrul.nuts.ApiClient;
import nl.reinkrul.nuts.ApiException;
import nl.reinkrul.nuts.ApiResponse;
import nl.reinkrul.nuts.Pair;
import nl.reinkrul.nuts.vdr.CreateDIDDefaultResponse;
import nl.reinkrul.nuts.vdr.DIDCreateRequest;
import nl.reinkrul.nuts.vdr.DIDResolutionResult;
import nl.reinkrul.nuts.vdr.DIDUpdateRequest;
import nl.reinkrul.nuts.vdr.VerificationMethodRelationship;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-05-12T15:13:43.291870+02:00[Europe/Amsterdam]")
public class DidApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public DidApi() {
this(new ApiClient());
}
public DidApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Creates and adds a new verificationMethod to the DID document.
* It creates a new private public keypair. The public key is wrapped in verificationMethod. This method is added to the DID Document. By default, the key usage (verificationMethod relationships) is the same as when creating a new DID document. To alter this, provide a body specifying the key usage. error returns: * 403 - Verification method could not be added because the DID is not managed by this node * 404 - Corresponding DID document could not be found * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param verificationMethodRelationship (optional)
* @return nl.reinkrul.nuts.common.VerificationMethod
* @throws ApiException if fails to make API call
*/
public nl.reinkrul.nuts.common.VerificationMethod addNewVerificationMethod(String did, VerificationMethodRelationship verificationMethodRelationship) throws ApiException {
ApiResponse localVarResponse = addNewVerificationMethodWithHttpInfo(did, verificationMethodRelationship);
return localVarResponse.getData();
}
/**
* Creates and adds a new verificationMethod to the DID document.
* It creates a new private public keypair. The public key is wrapped in verificationMethod. This method is added to the DID Document. By default, the key usage (verificationMethod relationships) is the same as when creating a new DID document. To alter this, provide a body specifying the key usage. error returns: * 403 - Verification method could not be added because the DID is not managed by this node * 404 - Corresponding DID document could not be found * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param verificationMethodRelationship (optional)
* @return ApiResponse<nl.reinkrul.nuts.common.VerificationMethod>
* @throws ApiException if fails to make API call
*/
public ApiResponse addNewVerificationMethodWithHttpInfo(String did, VerificationMethodRelationship verificationMethodRelationship) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addNewVerificationMethodRequestBuilder(did, verificationMethodRelationship);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addNewVerificationMethod", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder addNewVerificationMethodRequestBuilder(String did, VerificationMethodRelationship verificationMethodRelationship) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling addNewVerificationMethod");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did/{did}/verificationmethod"
.replace("{did}", ApiClient.urlEncode(did.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(verificationMethodRelationship);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Retrieve the list of conflicted DID documents
* Resolves DID documents with a conflict. It returns both the DID Document and metadata of the DID Document. error returns: * 500 - An error occurred while processing the request
* @return List<DIDResolutionResult>
* @throws ApiException if fails to make API call
*/
public List conflictedDIDs() throws ApiException {
ApiResponse> localVarResponse = conflictedDIDsWithHttpInfo();
return localVarResponse.getData();
}
/**
* Retrieve the list of conflicted DID documents
* Resolves DID documents with a conflict. It returns both the DID Document and metadata of the DID Document. error returns: * 500 - An error occurred while processing the request
* @return ApiResponse<List<DIDResolutionResult>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> conflictedDIDsWithHttpInfo() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = conflictedDIDsRequestBuilder();
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("conflictedDIDs", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder conflictedDIDsRequestBuilder() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did/conflicted";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Creates a new Nuts DID
* The DID Document will be created according to the given request. If a combination of options is not allowed, a 400 is returned. The default values for selfControl, assertionMethod, keyAgreement, and capabilityInvocation are true. The default for controllers is an empty list. All other options default to false. Only a single keypair will be generated. All enabled methods will reuse the same key pair. A seperate keypair will be generated to generate the DID if SelfControl is false. error returns: * 400 - Invalid (combination of) options * 500 - An error occurred while processing the request
* @param diDCreateRequest (required)
* @return nl.reinkrul.nuts.common.DIDDocument
* @throws ApiException if fails to make API call
*/
public nl.reinkrul.nuts.common.DIDDocument createDID(DIDCreateRequest diDCreateRequest) throws ApiException {
ApiResponse localVarResponse = createDIDWithHttpInfo(diDCreateRequest);
return localVarResponse.getData();
}
/**
* Creates a new Nuts DID
* The DID Document will be created according to the given request. If a combination of options is not allowed, a 400 is returned. The default values for selfControl, assertionMethod, keyAgreement, and capabilityInvocation are true. The default for controllers is an empty list. All other options default to false. Only a single keypair will be generated. All enabled methods will reuse the same key pair. A seperate keypair will be generated to generate the DID if SelfControl is false. error returns: * 400 - Invalid (combination of) options * 500 - An error occurred while processing the request
* @param diDCreateRequest (required)
* @return ApiResponse<nl.reinkrul.nuts.common.DIDDocument>
* @throws ApiException if fails to make API call
*/
public ApiResponse createDIDWithHttpInfo(DIDCreateRequest diDCreateRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createDIDRequestBuilder(diDCreateRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createDID", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createDIDRequestBuilder(DIDCreateRequest diDCreateRequest) throws ApiException {
// verify the required parameter 'diDCreateRequest' is set
if (diDCreateRequest == null) {
throw new ApiException(400, "Missing the required parameter 'diDCreateRequest' when calling createDID");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(diDCreateRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Deactivates a Nuts DID document according to the specification.
* Updates a Nuts DID document. error returns: * 400 - DID document could not be deleted because the DID param was malformed * 403 - DID document could not be deleted because the DID is not managed by this node * 404 - Corresponding DID document could not be found * 409 - DID document could not be deactivated because the the document was already deactivated * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @throws ApiException if fails to make API call
*/
public void deactivateDID(String did) throws ApiException {
deactivateDIDWithHttpInfo(did);
}
/**
* Deactivates a Nuts DID document according to the specification.
* Updates a Nuts DID document. error returns: * 400 - DID document could not be deleted because the DID param was malformed * 403 - DID document could not be deleted because the DID is not managed by this node * 404 - Corresponding DID document could not be found * 409 - DID document could not be deactivated because the the document was already deactivated * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deactivateDIDWithHttpInfo(String did) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deactivateDIDRequestBuilder(did);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deactivateDID", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deactivateDIDRequestBuilder(String did) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling deactivateDID");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did/{did}"
.replace("{did}", ApiClient.urlEncode(did.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/problem+json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Delete a specific verification method
* Removes the verification method from the DID Document. Revokes the public key with the corresponding key-id. Note: Other verification methods with different key-ids with the same private key will still be valid. error returns: * 403 - Verification method could not be deleted because the DID is not managed by this node * 404 - Corresponding DID document or verification method could not be found * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param kid URL encoded DID identifying the verification method. (required)
* @throws ApiException if fails to make API call
*/
public void deleteVerificationMethod(String did, String kid) throws ApiException {
deleteVerificationMethodWithHttpInfo(did, kid);
}
/**
* Delete a specific verification method
* Removes the verification method from the DID Document. Revokes the public key with the corresponding key-id. Note: Other verification methods with different key-ids with the same private key will still be valid. error returns: * 403 - Verification method could not be deleted because the DID is not managed by this node * 404 - Corresponding DID document or verification method could not be found * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param kid URL encoded DID identifying the verification method. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteVerificationMethodWithHttpInfo(String did, String kid) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteVerificationMethodRequestBuilder(did, kid);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteVerificationMethod", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteVerificationMethodRequestBuilder(String did, String kid) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling deleteVerificationMethod");
}
// verify the required parameter 'kid' is set
if (kid == null) {
throw new ApiException(400, "Missing the required parameter 'kid' when calling deleteVerificationMethod");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did/{did}/verificationmethod/{kid}"
.replace("{did}", ApiClient.urlEncode(did.toString()))
.replace("{kid}", ApiClient.urlEncode(kid.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/problem+json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Resolves a Nuts DID document
* Resolves a Nuts DID document. It also resolves deactivated documents. error returns: * 400 - Returned in case of malformed DID * 404 - Corresponding DID document could not be found * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param versionId If a versionId parameter is provided, the DID resolution algorithm returns a specific version of the DID document. The version is the Sha256 hash of the document. The DID parameters versionId and versionTime are mutually exclusive. See [the did resolution spec about versioning](https://w3c-ccg.github.io/did-resolution/#versioning) (optional)
* @param versionTime If a versionTime parameter is provided, the DID resolution algorithm returns a specific version of the DID document. The DID parameters versionId and versionTime are mutually exclusive. See [the did resolution spec about versioning](https://w3c-ccg.github.io/did-resolution/#versioning) (optional)
* @return DIDResolutionResult
* @throws ApiException if fails to make API call
*/
public DIDResolutionResult getDID(String did, String versionId, String versionTime) throws ApiException {
ApiResponse localVarResponse = getDIDWithHttpInfo(did, versionId, versionTime);
return localVarResponse.getData();
}
/**
* Resolves a Nuts DID document
* Resolves a Nuts DID document. It also resolves deactivated documents. error returns: * 400 - Returned in case of malformed DID * 404 - Corresponding DID document could not be found * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param versionId If a versionId parameter is provided, the DID resolution algorithm returns a specific version of the DID document. The version is the Sha256 hash of the document. The DID parameters versionId and versionTime are mutually exclusive. See [the did resolution spec about versioning](https://w3c-ccg.github.io/did-resolution/#versioning) (optional)
* @param versionTime If a versionTime parameter is provided, the DID resolution algorithm returns a specific version of the DID document. The DID parameters versionId and versionTime are mutually exclusive. See [the did resolution spec about versioning](https://w3c-ccg.github.io/did-resolution/#versioning) (optional)
* @return ApiResponse<DIDResolutionResult>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDIDWithHttpInfo(String did, String versionId, String versionTime) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDIDRequestBuilder(did, versionId, versionTime);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDID", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getDIDRequestBuilder(String did, String versionId, String versionTime) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling getDID");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did/{did}"
.replace("{did}", ApiClient.urlEncode(did.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "versionId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("versionId", versionId));
localVarQueryParameterBaseName = "versionTime";
localVarQueryParams.addAll(ApiClient.parameterToPairs("versionTime", versionTime));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Updates a Nuts DID document.
* Updates a Nuts DID document. error returns: * 400 - DID document could not be updated because the DID param was malformed or the DID document is invalid * 403 - DID document could not be updated because the DID is not managed by this node * 404 - Corresponding DID document could not be found * 409 - DID document could not be updated because the document is deactivated or its controllers are deactivated * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param diDUpdateRequest (required)
* @return nl.reinkrul.nuts.common.DIDDocument
* @throws ApiException if fails to make API call
*/
public nl.reinkrul.nuts.common.DIDDocument updateDID(String did, DIDUpdateRequest diDUpdateRequest) throws ApiException {
ApiResponse localVarResponse = updateDIDWithHttpInfo(did, diDUpdateRequest);
return localVarResponse.getData();
}
/**
* Updates a Nuts DID document.
* Updates a Nuts DID document. error returns: * 400 - DID document could not be updated because the DID param was malformed or the DID document is invalid * 403 - DID document could not be updated because the DID is not managed by this node * 404 - Corresponding DID document could not be found * 409 - DID document could not be updated because the document is deactivated or its controllers are deactivated * 500 - An error occurred while processing the request
* @param did URL encoded DID. (required)
* @param diDUpdateRequest (required)
* @return ApiResponse<nl.reinkrul.nuts.common.DIDDocument>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateDIDWithHttpInfo(String did, DIDUpdateRequest diDUpdateRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateDIDRequestBuilder(did, diDUpdateRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateDID", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder updateDIDRequestBuilder(String did, DIDUpdateRequest diDUpdateRequest) throws ApiException {
// verify the required parameter 'did' is set
if (did == null) {
throw new ApiException(400, "Missing the required parameter 'did' when calling updateDID");
}
// verify the required parameter 'diDUpdateRequest' is set
if (diDUpdateRequest == null) {
throw new ApiException(400, "Missing the required parameter 'diDUpdateRequest' when calling updateDID");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/internal/vdr/v1/did/{did}"
.replace("{did}", ApiClient.urlEncode(did.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json, application/problem+json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(diDUpdateRequest);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy