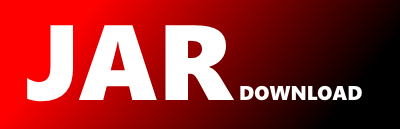
nl.reinkrul.nuts.vdr.VerificationMethodRelationship Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java client library for using the Nuts Node's REST API.
/*
* Nuts Verifiable Data Registry API spec
* API specification for the Verifiable Data Registry
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.reinkrul.nuts.vdr;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* VerificationMethodRelationship
*/
@JsonPropertyOrder({
VerificationMethodRelationship.JSON_PROPERTY_ASSERTION_METHOD,
VerificationMethodRelationship.JSON_PROPERTY_AUTHENTICATION,
VerificationMethodRelationship.JSON_PROPERTY_CAPABILITY_INVOCATION,
VerificationMethodRelationship.JSON_PROPERTY_CAPABILITY_DELEGATION,
VerificationMethodRelationship.JSON_PROPERTY_KEY_AGREEMENT,
VerificationMethodRelationship.JSON_PROPERTY_SELF_CONTROL
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-05-12T15:13:43.291870+02:00[Europe/Amsterdam]")
public class VerificationMethodRelationship {
public static final String JSON_PROPERTY_ASSERTION_METHOD = "assertionMethod";
private Boolean assertionMethod = true;
public static final String JSON_PROPERTY_AUTHENTICATION = "authentication";
private Boolean authentication = false;
public static final String JSON_PROPERTY_CAPABILITY_INVOCATION = "capabilityInvocation";
private Boolean capabilityInvocation;
public static final String JSON_PROPERTY_CAPABILITY_DELEGATION = "capabilityDelegation";
private Boolean capabilityDelegation = true;
public static final String JSON_PROPERTY_KEY_AGREEMENT = "keyAgreement";
private Boolean keyAgreement = true;
public static final String JSON_PROPERTY_SELF_CONTROL = "selfControl";
private Boolean selfControl = true;
public VerificationMethodRelationship() {
}
public VerificationMethodRelationship assertionMethod(Boolean assertionMethod) {
this.assertionMethod = assertionMethod;
return this;
}
/**
* indicates if the generated key pair can be used for assertions.
* @return assertionMethod
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ASSERTION_METHOD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAssertionMethod() {
return assertionMethod;
}
@JsonProperty(JSON_PROPERTY_ASSERTION_METHOD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAssertionMethod(Boolean assertionMethod) {
this.assertionMethod = assertionMethod;
}
public VerificationMethodRelationship authentication(Boolean authentication) {
this.authentication = authentication;
return this;
}
/**
* indicates if the generated key pair can be used for authentication.
* @return authentication
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AUTHENTICATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAuthentication() {
return authentication;
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthentication(Boolean authentication) {
this.authentication = authentication;
}
public VerificationMethodRelationship capabilityInvocation(Boolean capabilityInvocation) {
this.capabilityInvocation = capabilityInvocation;
return this;
}
/**
* indicates if the generated key pair can be used for altering DID Documents. In combination with selfControl = true, the key can be used to alter the new DID Document. Defaults to true when not given. default: true
* @return capabilityInvocation
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CAPABILITY_INVOCATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCapabilityInvocation() {
return capabilityInvocation;
}
@JsonProperty(JSON_PROPERTY_CAPABILITY_INVOCATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCapabilityInvocation(Boolean capabilityInvocation) {
this.capabilityInvocation = capabilityInvocation;
}
public VerificationMethodRelationship capabilityDelegation(Boolean capabilityDelegation) {
this.capabilityDelegation = capabilityDelegation;
return this;
}
/**
* indicates if the generated key pair can be used for capability delegations.
* @return capabilityDelegation
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CAPABILITY_DELEGATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCapabilityDelegation() {
return capabilityDelegation;
}
@JsonProperty(JSON_PROPERTY_CAPABILITY_DELEGATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCapabilityDelegation(Boolean capabilityDelegation) {
this.capabilityDelegation = capabilityDelegation;
}
public VerificationMethodRelationship keyAgreement(Boolean keyAgreement) {
this.keyAgreement = keyAgreement;
return this;
}
/**
* indicates if the generated key pair can be used for Key agreements.
* @return keyAgreement
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_KEY_AGREEMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getKeyAgreement() {
return keyAgreement;
}
@JsonProperty(JSON_PROPERTY_KEY_AGREEMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setKeyAgreement(Boolean keyAgreement) {
this.keyAgreement = keyAgreement;
}
public VerificationMethodRelationship selfControl(Boolean selfControl) {
this.selfControl = selfControl;
return this;
}
/**
* whether the generated DID Document can be altered with its own capabilityInvocation key.
* @return selfControl
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SELF_CONTROL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getSelfControl() {
return selfControl;
}
@JsonProperty(JSON_PROPERTY_SELF_CONTROL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSelfControl(Boolean selfControl) {
this.selfControl = selfControl;
}
/**
* Return true if this VerificationMethodRelationship object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
VerificationMethodRelationship verificationMethodRelationship = (VerificationMethodRelationship) o;
return Objects.equals(this.assertionMethod, verificationMethodRelationship.assertionMethod) &&
Objects.equals(this.authentication, verificationMethodRelationship.authentication) &&
Objects.equals(this.capabilityInvocation, verificationMethodRelationship.capabilityInvocation) &&
Objects.equals(this.capabilityDelegation, verificationMethodRelationship.capabilityDelegation) &&
Objects.equals(this.keyAgreement, verificationMethodRelationship.keyAgreement) &&
Objects.equals(this.selfControl, verificationMethodRelationship.selfControl);
}
@Override
public int hashCode() {
return Objects.hash(assertionMethod, authentication, capabilityInvocation, capabilityDelegation, keyAgreement, selfControl);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class VerificationMethodRelationship {\n");
sb.append(" assertionMethod: ").append(toIndentedString(assertionMethod)).append("\n");
sb.append(" authentication: ").append(toIndentedString(authentication)).append("\n");
sb.append(" capabilityInvocation: ").append(toIndentedString(capabilityInvocation)).append("\n");
sb.append(" capabilityDelegation: ").append(toIndentedString(capabilityDelegation)).append("\n");
sb.append(" keyAgreement: ").append(toIndentedString(keyAgreement)).append("\n");
sb.append(" selfControl: ").append(toIndentedString(selfControl)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `assertionMethod` to the URL query string
if (getAssertionMethod() != null) {
joiner.add(String.format("%sassertionMethod%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAssertionMethod()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `authentication` to the URL query string
if (getAuthentication() != null) {
joiner.add(String.format("%sauthentication%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAuthentication()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `capabilityInvocation` to the URL query string
if (getCapabilityInvocation() != null) {
joiner.add(String.format("%scapabilityInvocation%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCapabilityInvocation()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `capabilityDelegation` to the URL query string
if (getCapabilityDelegation() != null) {
joiner.add(String.format("%scapabilityDelegation%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCapabilityDelegation()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `keyAgreement` to the URL query string
if (getKeyAgreement() != null) {
joiner.add(String.format("%skeyAgreement%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getKeyAgreement()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `selfControl` to the URL query string
if (getSelfControl() != null) {
joiner.add(String.format("%sselfControl%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSelfControl()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy