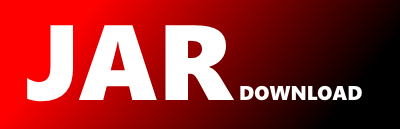
nl.robfaber.serial.SerialUtil Maven / Gradle / Ivy
package nl.robfaber.serial;
import gnu.io.CommPortIdentifier;
import gnu.io.NoSuchPortException;
import lombok.extern.slf4j.Slf4j;
import lombok.val;
import org.slf4j.MarkerFactory;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
@Slf4j
public class SerialUtil {
public static SerialPort setupSerialPort(String name, String port, String matchString, Integer baudrate, SerialPortMessageListener listener) throws Exception {
val marker = MarkerFactory.getMarker(name);
log.info(marker, "Setting up "+name);
if (port == null || port.length() == 0) {
port = SerialUtil.autodiscoverPort(name, matchString);
log.info(marker, "Autodiscovery returned "+port);
}
if (port == null) {
log.warn("Failed to determine port");
} else {
try {
SerialPort serialPort = new SerialPort(port, baudrate, listener);
serialPort.connect();
log.info(marker, "Done");
return serialPort;
} catch (NoSuchPortException e) {
log.error(marker, "Failed to connect port", e);
}
}
return null;
}
public static SerialPortStream setupSerialStream(String name, String port, String matchString, int baudrate) throws Exception {
val marker = MarkerFactory.getMarker(name);
log.info(marker, "Setting up "+name);
if (port == null || port.length() == 0) {
port = SerialUtil.autodiscoverPort(name, matchString);
log.info(marker, "Autodiscovery returned "+port);
}
if (port == null) {
log.warn(marker, "Failed to determine port");
} else {
try {
SerialPortStream serialPort = new SerialPortStream(port, baudrate);
serialPort.connect();
log.info(marker, "Done");
return serialPort;
} catch (NoSuchPortException e) {
log.error(marker, "Failed to connect port", e);
}
}
return null;
}
private static String autodiscoverPort(String modemName, String matchString) {
val marker = MarkerFactory.getMarker(modemName);
log.info(marker, "Trying to autodiscover port for "+modemName);
Process p;
try {
String[] cmd = {
"/bin/sh",
"-c",
"ls -l /dev/serial/by-id | grep "+matchString
};
p = Runtime.getRuntime().exec(cmd);
p.waitFor();
BufferedReader reader = new BufferedReader(new InputStreamReader(p.getInputStream()));
StringBuffer sb = new StringBuffer();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line + "\n");
}
String output = sb.toString();
log.info(output);
if (output != null) {
if (output.contains("ttyUSB0")) {
return "/dev/ttyUSB0";
} else if (output.contains("ttyUSB1")) {
return "/dev/ttyUSB1";
} else if (output.contains("ttyUSB2")) {
return "/dev/ttyUSB2";
} else if (output.contains("ttyUSB3")) {
return "/dev/ttyUSB3";
} else if (output.contains("ttyUSB4")) {
return "/dev/ttyUSB4";
} else if (output.contains("ttyUSB5")) {
return "/dev/ttyUSB5";
}
}
} catch (Exception e) {
log.error(marker, "Failed to autodiscover", e);
}
return null;
}
public static List listPorts(String prefix) {
List ports = new ArrayList<>();
Enumeration e = CommPortIdentifier.getPortIdentifiers();
while (e.hasMoreElements()) {
CommPortIdentifier port = (CommPortIdentifier)e.nextElement();
log.info("Port {}", port.getName());
if (port.getName().startsWith(prefix)) {
ports.add(port.getName());
}
}
return ports;
}
public static void main(String[] args) {
listPorts("/dev/tty.usb").get(0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy