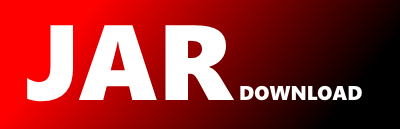
nl.talsmasoftware.context.servletrequest.ServletRequestContext Maven / Gradle / Ivy
package nl.talsmasoftware.context.servletrequest;
import nl.talsmasoftware.context.Context;
import nl.talsmasoftware.context.threadlocal.AbstractThreadLocalContext;
import javax.servlet.ServletRequest;
/**
* ThreadLocal context containing the current {@link ServletRequest}.
*
* @author Sjoerd Talsma
*/
final class ServletRequestContext extends AbstractThreadLocalContext {
/**
* The ThreadLocal context containing the {@link ServletRequest}.
*/
private static final ThreadLocal CONTEXT = threadLocalInstanceOf(ServletRequestContext.class);
/**
* Creates a new context with the specified request.
*
* The new context will be made the active context for the current thread.
*
* @param newValue The new value to become active in this new context
* (or null
to register a new context with 'no value').
*/
ServletRequestContext(ServletRequest newValue) {
super(newValue);
}
/**
* The current servlet request context.
*
* If no active {@link ServletRequest} is found, a 'dummy', already-closed context is returned.
*
* @return The context with the current ServletRequest.
* The context itself is non-null
, but may contain a null
value.
* @see #getValue()
*/
static Context current() {
final ServletRequestContext current = CONTEXT.get();
return current != null ? current : DummyContext.INSTANCE;
}
/**
* {@link #close()} restores the previous context, but clear() unconditionally removes the active context.
*
* This is useful for boundary filters, whose Threads may be returned to some threadpool.
*/
static void clear() {
CONTEXT.remove();
}
/**
* Dummy context containing.
*/
private static final class DummyContext implements Context {
private static final DummyContext INSTANCE = new DummyContext();
public ServletRequest getValue() {
return null;
}
public void close() {
}
@Override
public String toString() {
return "DummyContext";
}
}
}