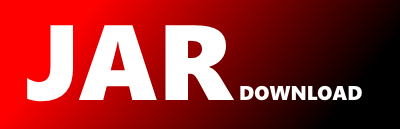
nl.topicus.jdbc.statement.CloudSpannerStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spanner-jdbc Show documentation
Show all versions of spanner-jdbc Show documentation
JDBC Driver for Google Cloud Spanner
package nl.topicus.jdbc.statement;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.regex.Pattern;
import nl.topicus.jdbc.shaded.com.google.cloud.spanner.DatabaseClient;
import nl.topicus.jdbc.shaded.com.google.cloud.spanner.ReadContext;
import nl.topicus.jdbc.shaded.com.google.rpc.Code;
import nl.topicus.jdbc.shaded.net.sf.jsqlparser.JSQLParserException;
import nl.topicus.jdbc.shaded.net.sf.jsqlparser.parser.CCJSqlParserUtil;
import nl.topicus.jdbc.shaded.net.sf.jsqlparser.parser.TokenMgrError;
import nl.topicus.jdbc.shaded.net.sf.jsqlparser.statement.Statement;
import nl.topicus.jdbc.shaded.net.sf.jsqlparser.statement.select.Select;
import nl.topicus.jdbc.CloudSpannerConnection;
import nl.topicus.jdbc.exception.CloudSpannerSQLException;
import nl.topicus.jdbc.resultset.CloudSpannerResultSet;
/**
*
* @author loite
*
*/
public class CloudSpannerStatement extends AbstractCloudSpannerStatement
{
protected ResultSet lastResultSet = null;
protected int lastUpdateCount = -1;
private Pattern nl.topicus.jdbc.shaded.com.entPattern = Pattern.nl.topicus.jdbc.shaded.com.ile("//.*|/\\*((.|\\n)(?!=*/))+\\*/|--.*(?=\\n)", Pattern.DOTALL);
public CloudSpannerStatement(CloudSpannerConnection connection, DatabaseClient dbClient)
{
super(connection, dbClient);
}
@Override
public ResultSet executeQuery(String sql) throws SQLException
{
try (ReadContext context = getReadContext())
{
nl.topicus.jdbc.shaded.com.google.cloud.spanner.ResultSet rs = context.executeQuery(nl.topicus.jdbc.shaded.com.google.cloud.spanner.Statement.of(sql));
return new CloudSpannerResultSet(this, rs);
}
}
@Override
public int executeUpdate(String sql) throws SQLException
{
PreparedStatement ps = getConnection().prepareStatement(sql);
return ps.executeUpdate();
}
@Override
public boolean execute(String sql) throws SQLException
{
Statement statement = null;
boolean ddl = isDDLStatement(sql);
if (!ddl)
{
try
{
statement = CCJSqlParserUtil.parse(sanitizeSQL(sql));
}
catch (JSQLParserException | TokenMgrError e)
{
throw new CloudSpannerSQLException(
"Error while parsing sql statement " + sql + ": " + e.getLocalizedMessage(),
Code.INVALID_ARGUMENT, e);
}
}
if (!ddl && statement instanceof Select)
{
lastResultSet = executeQuery(sql);
lastUpdateCount = -1;
return true;
}
else
{
lastUpdateCount = executeUpdate(sql);
lastResultSet = null;
return false;
}
}
private static final String[] DDL_STATEMENTS = { "CREATE", "ALTER", "DROP" };
/**
* Do a quick check if this SQL statement is a DDL statement
*
* @param sql
* The statement to check
* @return true if the SQL statement is a DDL statement
*/
protected boolean isDDLStatement(String sql)
{
String ddl = removeComments(sql);
ddl = ddl.substring(0, Math.min(8, ddl.length())).toUpperCase();
for (String statement : DDL_STATEMENTS)
{
if (ddl.startsWith(statement))
return true;
}
return false;
}
protected String removeComments(String sql)
{
return nl.topicus.jdbc.shaded.com.entPattern.matcher(sql).replaceAll("").trim();
}
protected boolean isSelectStatement(String sql)
{
String select = removeComments(sql);
select = select.substring(0, Math.min(6, select.length())).toUpperCase();
if (select.startsWith("SELECT"))
return true;
return false;
}
@Override
public ResultSet getResultSet() throws SQLException
{
return lastResultSet;
}
@Override
public int getUpdateCount() throws SQLException
{
return lastUpdateCount;
}
@Override
public boolean getMoreResults() throws SQLException
{
moveToNextResult(CLOSE_CURRENT_RESULT);
return false;
}
@Override
public boolean getMoreResults(int current) throws SQLException
{
moveToNextResult(current);
return false;
}
private void moveToNextResult(int current) throws SQLException
{
if (current != java.sql.Statement.KEEP_CURRENT_RESULT && lastResultSet != null)
lastResultSet.close();
lastResultSet = null;
lastUpdateCount = -1;
}
@Override
public int executeUpdate(String sql, int autoGeneratedKeys) throws SQLException
{
throw new SQLFeatureNotSupportedException();
}
@Override
public int executeUpdate(String sql, int[] columnIndexes) throws SQLException
{
throw new SQLFeatureNotSupportedException();
}
@Override
public int executeUpdate(String sql, String[] columnNames) throws SQLException
{
throw new SQLFeatureNotSupportedException();
}
@Override
public boolean execute(String sql, int autoGeneratedKeys) throws SQLException
{
throw new SQLFeatureNotSupportedException();
}
@Override
public boolean execute(String sql, int[] columnIndexes) throws SQLException
{
throw new SQLFeatureNotSupportedException();
}
@Override
public boolean execute(String sql, String[] columnNames) throws SQLException
{
throw new SQLFeatureNotSupportedException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy