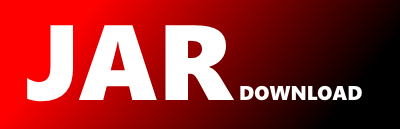
nl.topicus.jdbc.shaded.io.grpc.internal.ChannelTracer Maven / Gradle / Ivy
/*
* Copyright 2018 The gRPC Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nl.topicus.jdbc.shaded.io.grpc.internal;
import static nl.topicus.jdbc.shaded.com.google.common.base.Preconditions.checkArgument;
import static nl.topicus.jdbc.shaded.com.google.common.base.Preconditions.checkNotNull;
import nl.topicus.jdbc.shaded.io.grpc.internal.Channelz.ChannelStats;
import nl.topicus.jdbc.shaded.io.grpc.internal.Channelz.ChannelTrace;
import nl.topicus.jdbc.shaded.io.grpc.internal.Channelz.ChannelTrace.Event;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import nl.topicus.jdbc.shaded.javax.annotation.concurrent.GuardedBy;
/**
* Tracks a collections of channel tracing events for a channel/subchannel.
*/
final class ChannelTracer {
private final Object lock = new Object();
@GuardedBy("lock")
private final Collection events;
private final long channelCreationTimeNanos;
@GuardedBy("lock")
private int eventsLogged;
/**
* Creates a channel tracer and log the creation event of the underlying channel.
*
* @param channelType Chennel, Subchannel, or OobChannel
*/
ChannelTracer(final int maxEvents, long channelCreationTimeNanos, String channelType) {
checkArgument(maxEvents > 0, "maxEvents must be greater than zero");
checkNotNull(channelType, "channelType");
events = new ArrayDeque() {
@GuardedBy("lock")
@Override
public boolean add(Event event) {
if (size() == maxEvents) {
removeFirst();
}
eventsLogged++;
return super.add(event);
}
};
this.channelCreationTimeNanos = channelCreationTimeNanos;
reportEvent(new ChannelTrace.Event.Builder()
.setDescription(channelType + " created")
.setSeverity(ChannelTrace.Event.Severity.CT_INFO)
.setTimestampNanos(channelCreationTimeNanos)
.build());
}
void reportEvent(Event event) {
synchronized (lock) {
events.add(event);
}
}
void updateBuilder(ChannelStats.Builder builder) {
List eventsSnapshot;
int eventsLoggedSnapshot;
synchronized (lock) {
eventsLoggedSnapshot = eventsLogged;
eventsSnapshot = new ArrayList(events);
}
builder.setChannelTrace(new ChannelTrace.Builder()
.setNumEventsLogged(eventsLoggedSnapshot)
.setCreationTimeNanos(channelCreationTimeNanos)
.setEvents(eventsSnapshot)
.build());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy