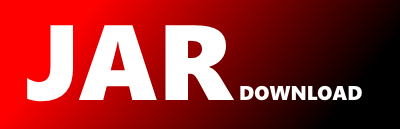
nl.trivento.albero.model.builders.AbstractBuildable Maven / Gradle / Ivy
/* Copyright 2011-2012 Profict Holding
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nl.trivento.albero.model.builders;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Simplifies implementing the {@link Buildable buildable interface}.
*
*/
abstract class AbstractBuildable implements Buildable {
private Map> properties;
/**
* Creates an abstract buildable object.
*/
public AbstractBuildable() {
properties = new HashMap>();
}
/**
* Adds an optional property.
*
* @param the type of the property
* @param name the property name
* @param type the property type
*/
protected void addProperty(String name, Class type) {
addProperty(name, type, null);
}
/**
* Adds an optional property.
*
* @param the type of the property
* @param name the property name
* @param type the property type
* @param defaultValue the default value of the property
*/
protected void addProperty(String name, Class type, T defaultValue) {
addProperty(name, type, false, defaultValue);
}
/**
* Adds a required property.
*
* @param the type of the property
* @param name the property name
* @param type the property type
*/
protected void addRequiredProperty(String name, Class type) {
addRequiredProperty(name, type, null);
}
/**
* Adds a required property.
*
* @param the type of the property
* @param name the property name
* @param type the property type
* @param defaultValue the default value of the property
*/
protected void addRequiredProperty(String name, Class type, T defaultValue) {
addProperty(name, type, true, defaultValue);
}
/**
* Adds a list property.
*
* @param the type of the list
* @param name the property name
* @param elementType the property element type
*/
protected void addListProperty(String name, Class elementType) {
addProperty(name, List.class, false, new ArrayList());
}
/**
* Adds a map property.
*
* @param the type of the key
* @param the type of the value
* @param name the property name
* @param keyType the property key type
* @param valueType the property value type
*/
protected void addMapProperty(String name, Class keyType, Class valueType) {
addProperty(name, Map.class, false, new HashMap());
}
private void addProperty(String name, Class type, boolean required, T defaultValue) {
properties.put(name, new Property(type, required, defaultValue));
}
/**
* Returns the value of a property.
*
* @param the type of the property
* @param name the property name
* @param type the property type
* @return the value of the property
* @throws BuilderException when no property with the given characteristics can be found
*/
public T getValue(String name, Class type) throws BuilderException {
return getProperty(name, type).value;
}
/**
* Sets the value of a property.
*
* @param the type of the property
* @param name the property name
* @param type the property type
* @param value the value for the property
* @throws BuilderException when no property with the given characteristics can be found
*/
public void setValue(String name, Class type, T value) throws BuilderException {
getProperty(name, type).value = value;
}
/**
* Returns a list property.
*
* @param the type of the elements
* @param name the property name
* @param elementType the property element type
* @return the list property
* @throws BuilderException when no list property with the given characteristics can be found
*/
public List getList(String name, Class elementType) throws BuilderException {
List> list = getValue(name, List.class);
@SuppressWarnings("unchecked")
List typedList = (List) list;
return typedList;
}
/**
* Adds a value to a list property.
*
* @param the type of the element
* @param name the property name
* @param elementType the property element type
* @param element the value to add
* @throws BuilderException when no list property with the given characteristics can be found
*/
public void addValue(String name, Class elementType, E element) throws BuilderException {
getList(name, elementType).add(element);
}
/**
* Returns a map property
*
* @param the type of the key
* @param the type of the value
* @param name the property name
* @param keyType the property key type
* @param valueType the property value type
* @return the map property
* @throws BuilderException when no map property with the given characteristics can be found
*/
public Map getMap(String name, Class keyType, Class valueType) throws BuilderException {
Map, ?> map = getValue(name, Map.class);
@SuppressWarnings("unchecked")
Map typedMap = (Map) map;
return typedMap;
}
/**
* Adds a value to a map property.
*
* @param the type of the key
* @param the type of the value
* @param name the property name
* @param keyType the property key type
* @param valueType the property value type
* @param key the key of the value
* @param value the value to add
* @throws BuilderException when no map property with the given characteristics can be found
*/
public void addValue(
String name, Class keyType, Class valueType, K key, V value) throws BuilderException {
getMap(name, keyType, valueType).put(key, value);
}
private Property getProperty(String name, Class type) throws BuilderException {
Property> property = properties.get(name);
if (property == null) {
throw new BuilderException("can't find property '", name, "'");
} else if (property.type != type) {
throw new BuilderException("property '", name, "' is of type ", property.type.getName(), ", ",
"not of type ", type.getName());
}
@SuppressWarnings("unchecked")
Property typedProperty = (Property) property;
return typedProperty;
}
public void checkValues() throws BuilderException {
Iterator>> it = properties.entrySet().iterator();
while (it.hasNext()) {
Map.Entry> namedProperty = it.next();
String name = namedProperty.getKey();
Property> property = namedProperty.getValue();
if (property.required) {
Object value = property.value;
if ((property.type == List.class) && ((List>) value).isEmpty()) {
throw new BuilderException("list '", name, "' should not be empty");
} else if ((property.type == Map.class) && ((Map, ?>) value).isEmpty()) {
throw new BuilderException("map '", name, "' should not be empty");
} else if (value == null) {
throw new BuilderException("property '", name, "' is required");
}
}
}
}
private static class Property {
public Class type;
public boolean required;
public T value;
public Property(Class type, boolean required, T value) {
this.type = type;
this.required = required;
this.value = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy