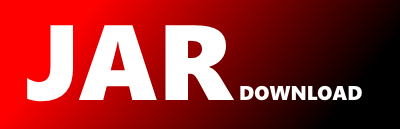
nl.tweeenveertig.openstack.client.core.AbstractContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of joss Show documentation
Show all versions of joss Show documentation
Java Client library for OpenStack Storage (Swift)
package nl.tweeenveertig.openstack.client.core;
import nl.tweeenveertig.openstack.instructions.SegmentationPlan;
import nl.tweeenveertig.openstack.instructions.UploadInstructions;
import nl.tweeenveertig.openstack.model.Account;
import nl.tweeenveertig.openstack.model.Container;
import nl.tweeenveertig.openstack.model.StoredObject;
import nl.tweeenveertig.openstack.exception.CommandException;
import nl.tweeenveertig.openstack.headers.Metadata;
import nl.tweeenveertig.openstack.headers.container.ContainerMetadata;
import nl.tweeenveertig.openstack.information.ContainerInformation;
import java.io.IOException;
import java.io.InputStream;
public abstract class AbstractContainer extends AbstractObjectStoreEntity implements Container {
protected String name;
private Account account;
public AbstractContainer(Account account, String name, boolean allowCaching) {
super(allowCaching);
this.name = name;
this.account = account;
this.info = new ContainerInformation();
}
public StoredObject getObjectSegment(String name, int part) {
return getObject(name + "/" + String.format("%08d", part));
}
public int getObjectCount() {
checkForInfo();
return info.getObjectCount();
}
public long getBytesUsed() {
checkForInfo();
return info.getBytesUsed();
}
public boolean isPublic() {
checkForInfo();
return info.isPublicContainer();
}
public String getName() {
return name;
}
public Account getAccount() {
return account;
}
public int hashCode() {
return getName().hashCode();
}
@SuppressWarnings("ConstantConditions")
public boolean equals(Object o) {
return o instanceof Container && getName().equals(((Container) o).getName());
}
@SuppressWarnings("ConstantConditions")
public int compareTo(Container o) {
return getName().compareTo(o.getName());
}
protected Metadata createMetadataEntry(String name, String value) {
return new ContainerMetadata(name, value);
}
public void uploadSegmentedObjects(String name, UploadInstructions uploadInstructions) {
try {
SegmentationPlan plan = uploadInstructions.getSegmentationPlan();
InputStream segmentStream = plan.getNextSegment();
while (segmentStream != null) {
StoredObject segment = getObjectSegment(name, plan.getSegmentNumber().intValue());
segment.uploadObject(segmentStream);
segmentStream.close();
segmentStream = plan.getNextSegment();
}
} catch (IOException err) {
throw new CommandException("Unable to upload segments", err);
}
}
}