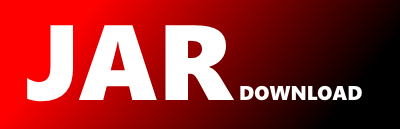
nl.tweeenveertig.openstack.command.identity.access.AccessImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of joss Show documentation
Show all versions of joss Show documentation
Java Client library for OpenStack Storage (Swift)
package nl.tweeenveertig.openstack.command.identity.access;
import nl.tweeenveertig.openstack.exception.CommandExceptionError;
import nl.tweeenveertig.openstack.exception.HttpStatusExceptionUtil;
import nl.tweeenveertig.openstack.model.Access;
import org.apache.http.HttpStatus;
import org.codehaus.jackson.annotate.JsonIgnore;
import org.codehaus.jackson.map.annotate.JsonRootName;
import java.util.ArrayList;
import java.util.List;
@JsonRootName(value="access")
public class AccessImpl implements Access {
public static final String SERVICE_CATALOG_OBJECT_STORE = "object-store";
public Token token;
public List serviceCatalog = new ArrayList();
public User user;
@JsonIgnore
private EndPoint currentEndPoint;
public void setPreferredRegion(String preferredRegion) {
this.currentEndPoint = getObjectStoreCatalog().getRegion(preferredRegion);
}
public String getToken() {
return token == null ? null : token.id;
}
public ServiceCatalog getObjectStoreCatalog() {
for (ServiceCatalog catalog : serviceCatalog) {
if (SERVICE_CATALOG_OBJECT_STORE.equals(catalog.type)) {
return catalog;
}
}
return null;
}
@SuppressWarnings("ConstantConditions")
public AccessImpl initCurrentEndPoint() {
ServiceCatalog objectStoreCatalog = getObjectStoreCatalog();
if (objectStoreCatalog == null) {
HttpStatusExceptionUtil.throwException(HttpStatus.SC_NOT_FOUND, CommandExceptionError.NO_SERVICE_CATALOG_FOUND);
}
this.currentEndPoint = objectStoreCatalog.getRegion(null);
if (this.currentEndPoint == null) {
HttpStatusExceptionUtil.throwException(HttpStatus.SC_NOT_FOUND, CommandExceptionError.NO_END_POINT_FOUND);
}
return this;
}
public String getInternalURL() {
return currentEndPoint.internalURL;
}
public String getPublicURL() {
return currentEndPoint.publicURL;
}
}