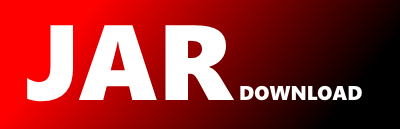
nl.vpro.domain.api.SearchResultItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-domain Show documentation
Show all versions of api-domain Show documentation
Contains the objects used by the Frontend API, like forms and result objects
/*
* Copyright (C) 2012 All rights reserved
* VPRO The Netherlands
*/
package nl.vpro.domain.api;
import lombok.Getter;
import lombok.Setter;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import com.fasterxml.jackson.annotation.*;
import nl.vpro.domain.media.Group;
import nl.vpro.domain.media.Program;
import nl.vpro.domain.media.Segment;
import nl.vpro.domain.page.Page;
/**
* @author rico
* @author Michiel Meeuwissen
*/
@XmlAccessorType(XmlAccessType.FIELD)
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"score", "highlights", "result"})
public class SearchResultItem {
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "objectType")
@JsonSubTypes({
@JsonSubTypes.Type(value = Program.class, name = "program"),
@JsonSubTypes.Type(value = Group.class, name = "group"),
@JsonSubTypes.Type(value = Segment.class, name = "segment"),
@JsonSubTypes.Type(value = Page.class, name = "page")
})
@Getter
@Setter
private T result;
@XmlAttribute
@Getter
@Setter
private Float score;
@XmlElement(name = "highlight")
@JsonProperty(value = "highlights")
private List highlights;
public SearchResultItem() {
}
public SearchResultItem(T item) {
this.result = item;
}
public SearchResultItem(T result, Float score, List highlights) {
this.result = result;
this.score = score;
this.highlights = highlights;
}
public List getHighlights() {
if(highlights == null) {
highlights = new ArrayList<>();
}
return highlights;
}
public void setHighlights(List highlights) {
this.highlights = highlights;
}
@Override
public String toString() {
return getResult() + ":" + getScore();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy