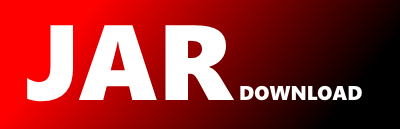
nl.vpro.domain.api.media.MediaFacetsResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-domain Show documentation
Show all versions of api-domain Show documentation
Contains the objects used by the Frontend API, like forms and result objects
/*
* Copyright (C) 2013 All rights reserved
* VPRO The Netherlands
*/
package nl.vpro.domain.api.media;
import lombok.Getter;
import lombok.Setter;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import nl.vpro.domain.api.*;
/**
* @author Roelof Jan Koekoek
* @since 2.0
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "mediaFacetsResultType", propOrder = {
"titles",
"types",
"avTypes",
"sortDates",
"broadcasters",
"genres",
"geoLocations",
"tags",
"durations",
"descendantOf",
"episodeOf",
"memberOf",
"relations",
"ageRatings",
"contentRatings"
})
@Getter
@Setter
public class MediaFacetsResult {
private List titles;
private List types;
private List avTypes;
private List sortDates;
private List broadcasters;
private List genres;
private List tags;
private List durations;
private List descendantOf;
private List episodeOf;
private List memberOf;
private List relations;
private List ageRatings;
private List contentRatings;
private List geoLocations;
List getMemberOf(MediaSearch search) {
if (search != null && search.getMemberOf() != null && memberOf == null) {
memberOf = new ArrayList<>();
}
return memberOf;
}
List getTypes(MediaSearch search) {
if (search != null && search.getTypes() != null && types == null) {
types = new ArrayList<>();
}
return types;
}
List getAvTypes(MediaSearch search) {
if (search != null && search.getAvTypes() != null && avTypes == null) {
avTypes = new ArrayList<>();
}
return avTypes;
}
List getSortDates(MediaSearch search) {
if (search != null && search.getSortDates() != null && sortDates == null) {
sortDates = new ArrayList<>();
}
return sortDates;
}
List getBroadcasters(MediaSearch search) {
if (search != null && search.getBroadcasters() != null && broadcasters == null) {
broadcasters = new ArrayList<>();
}
return broadcasters;
}
List getGenres(MediaSearch search) {
if (search != null && search.getGenres() != null && genres == null) {
genres = new ArrayList<>();
}
return genres;
}
List getTags(MediaSearch search) {
if (search != null && search.getTags() != null && tags == null) {
tags = new ArrayList<>();
}
return tags;
}
List getDurations(MediaSearch search) {
if (search != null && search.getDurations() != null && durations == null) {
durations = new ArrayList<>();
}
return durations;
}
List getDescendantOf(MediaSearch search) {
if (search != null && search.getDescendantOf() != null && descendantOf == null) {
descendantOf = new ArrayList<>();
}
return descendantOf;
}
List getEpisodeOf(MediaSearch search) {
if (search != null && search.getEpisodeOf() != null && memberOf == null) {
memberOf = new ArrayList<>();
}
return memberOf;
}
List getRelations(MediaSearch search) {
if (search != null && search.getRelations() != null && relations == null) {
relations = new ArrayList<>();
}
return relations;
}
List getAgeRating(MediaSearch search) {
if (search != null && search.getAgeRatings() != null && ageRatings == null) {
ageRatings = new ArrayList<>();
}
return ageRatings;
}
List getContentRatings(MediaSearch search) {
if (search != null && search.getContentRatings() != null && contentRatings == null) {
contentRatings = new ArrayList<>();
}
return contentRatings;
}
List getTitles(MediaSearch search) {
if (search != null && search.getTitles() != null && titles == null) {
titles = new ArrayList<>();
}
return titles;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy