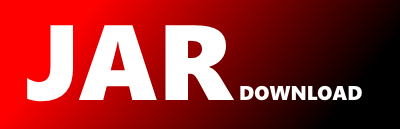
nl.vpro.domain.constraint.AbstractAnd Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-domain Show documentation
Show all versions of api-domain Show documentation
Contains the objects used by the Frontend API, like forms and result objects
/*
* Copyright (C) 2013 All rights reserved
* VPRO The Netherlands
*/
package nl.vpro.domain.constraint;
import java.util.*;
import java.util.stream.Collectors;
import javax.el.ELContext;
import javax.xml.bind.annotation.*;
import org.checkerframework.checker.nullness.qual.Nullable;
import static nl.vpro.domain.constraint.PredicateTestResult.FACTORY;
/**
* @author Roelof Jan Koekoek
* @since 2.0
*/
@XmlAccessorType(XmlAccessType.NONE)
@XmlTransient
public abstract class AbstractAnd implements Constraint {
protected List> constraints = new ArrayList<>();
protected AbstractAnd() {
}
@SafeVarargs
public AbstractAnd(Constraint... constraints) {
this.constraints = new ArrayList<>(Arrays.asList(constraints));
}
public AbstractAnd(List> constraints) {
this.constraints = constraints;
}
public List> getConstraints() {
return constraints;
}
public void setConstraint(List> constraints) {
this.constraints = constraints;
}
@Override
public boolean test(@Nullable T t) {
return constraints == null ||
constraints.stream()
.filter(Objects::nonNull)
.allMatch(p -> p.test(t));
}
@Override
public AndPredicateTestResult testWithReason(@Nullable T t) {
List clauses = constraints.stream().map(c -> c.testWithReason(t)).collect(Collectors.toList());
return DisplayablePredicates.and(this, t, clauses);
}
@Override
public void setELContext(ELContext ctx, Object value, Locale locale, PredicateTestResult result) {
Constraint.super.setELContext(ctx, value, locale, result);
AndPredicateTestResult andResult = (AndPredicateTestResult) result;
List failingClauses = andResult
.getClauses()
.stream()
.filter(p -> ! p.applies())
.map(p -> p.getDescription(locale))
.collect(Collectors.toList());
ResourceBundle bundle = DisplayablePredicates.getBundleForFalse(this, locale);
String joinedfailingclauses = failingClauses.stream()
.collect(Collectors.joining(" " + bundle.getString("AND") + " "));
ctx.getVariableMapper().setVariable("failingclauses",
FACTORY.createValueExpression(failingClauses, List.class));
ctx.getVariableMapper().setVariable("joinedfailingclauses",
FACTORY.createValueExpression(joinedfailingclauses, String.class));
}
@Override
public String toString() {
return constraints.stream().map(Object::toString).collect(Collectors.joining(" AND "));
}
@Override
public List getDefaultBundleKey() {
List result = Constraint.super.getDefaultBundleKey();
result.add(0, "And");
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy