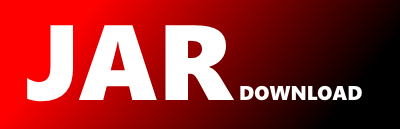
nl.vpro.domain.api.AbstractTextMatcherList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-domain Show documentation
Show all versions of api-domain Show documentation
Contains the objects used by the Frontend API, like forms and result objects
The newest version!
/*
* Copyright (C) 2016 Licensed under the Apache License, Version 2.0
* VPRO The Netherlands
*/
package nl.vpro.domain.api;
import java.util.*;
import java.util.function.Predicate;
import jakarta.validation.Valid;
import jakarta.xml.bind.annotation.XmlTransient;
/**
* @author rico
* @since 4.6
*/
@XmlTransient
public abstract class AbstractTextMatcherList, S extends MatchType>
extends MatcherList
implements Predicate {
@Valid
protected List matchers = new ArrayList<>();
protected AbstractTextMatcherList() {}
public AbstractTextMatcherList(List matchers) {
this.matchers = matchers;
}
public AbstractTextMatcherList(Match m, List matchers) {
super(m);
this.matchers = matchers;
}
@Override
public List asList() {
return matchers;
}
public List getMatchers() {
return matchers;
}
public void setMatchers(List matchers) {
this.matchers = matchers;
}
public boolean searchEquals(AbstractTextMatcherList b) {
if (b == null) {
return false;
}
if (size() != b.size()) {
return false;
}
for (int i = 0; i < size(); i++) {
T valuea = get(i);
T valueb = b.get(i);
if (valuea.getMatch() == Match.NOT || valueb.getMatch() == Match.NOT) {
return false;
}
if (! searchEquals(valuea, valueb)) {
return false;
}
}
return true;
}
protected boolean searchEquals(T a, T b) {
if (a == null || b == null) {
return a == b;
}
String aValue = a.getValue();
String bValue = b.getValue();
if (aValue == null || bValue == null) {
return Objects.equals(aValue, bValue);
}
if (a.isCaseSensitive() && b.isCaseSensitive()) {
return aValue.equals(bValue);
} else {
return aValue.equalsIgnoreCase(bValue);
}
}
public static , S extends MatchType> boolean searchEquals(AbstractTextMatcherList a, AbstractTextMatcherList b) {
if (a == null) {
return b == null;
}
return a.searchEquals(b);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AbstractTextMatcherList, ?> that = (AbstractTextMatcherList, ?>) o;
return matchers != null ? matchers.equals(that.matchers) : that.matchers == null;
}
@Override
public int hashCode() {
return matchers != null ? matchers.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy