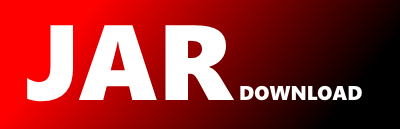
nl.vpro.nep.sam.api.StreamApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of media-nep Show documentation
Show all versions of media-nep Show documentation
Support for the several APIs of NEP that POMS is integrating with
package nl.vpro.nep.sam.api;
import nl.vpro.nep.sam.invoker.ApiException;
import nl.vpro.nep.sam.invoker.ApiClient;
import nl.vpro.nep.sam.invoker.Configuration;
import nl.vpro.nep.sam.invoker.Pair;
import jakarta.ws.rs.core.GenericType;
import nl.vpro.nep.sam.model.Errors;
import nl.vpro.nep.sam.model.StreamInputItem;
import nl.vpro.nep.sam.model.StreamOutputItem;
import nl.vpro.nep.sam.model.StreamPatchItem;
import nl.vpro.nep.sam.model.StreamResponseCollection;
import nl.vpro.nep.sam.model.StreamTypeEnum;
import nl.vpro.nep.sam.model.TechnicalStatusEnum;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-18T10:26:20.602909888Z[Etc/UTC]", comments = "Generator version: 7.6.0")
public class StreamApi {
private ApiClient apiClient;
public StreamApi() {
this(Configuration.getDefaultApiClient());
}
public StreamApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get streams
* Returns a list of streams that are available to the current user
* @param order Comma-separated fields to order by. Prefix with \"-\" to reverse order. (optional)
* @param search Used to search by streamId (optional)
* @param page Page number (optional, default to 1)
* @param limit Amount of items per page (optional, default to 30)
* @param provider Filter by provider (id) (optional)
* @param streamType Filter by stream type (name) (optional)
* @param status Filter by status (optional)
* @param technicalStatus Filter by technical status (optional)
* @param edgePool Filter by edge pool (id) (optional)
* @param streamId Filter by stream id (optional)
* @param archived If 1, only archived streams are returned. If 0, only non-archived streams are returned. If empty, all streams are returned. (optional)
* @return a {@code StreamResponseCollection}
* @throws ApiException if fails to make API call
*/
public StreamResponseCollection v2StreamsGet(String order, String search, Integer page, Integer limit, Integer provider, StreamTypeEnum streamType, String status, TechnicalStatusEnum technicalStatus, Integer edgePool, String streamId, Integer archived) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v2/streams".replaceAll("\\{format\\}","json");
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "order", order));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "search", search));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page", page));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "provider", provider));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "streamType", streamType));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "status", status));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "technicalStatus", technicalStatus));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "edgePool", edgePool));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "streamId", streamId));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "archived", archived));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "bearerAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new stream
* Create a new stream
* @param streamInputItem stream (required)
* @return a {@code StreamOutputItem}
* @throws ApiException if fails to make API call
*/
public StreamOutputItem v2StreamsPost(StreamInputItem streamInputItem) throws ApiException {
Object localVarPostBody = streamInputItem;
// verify the required parameter 'streamInputItem' is set
if (streamInputItem == null) {
throw new ApiException(400, "Missing the required parameter 'streamInputItem' when calling v2StreamsPost");
}
// create path and map variables
String localVarPath = "/v2/streams".replaceAll("\\{format\\}","json");
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "bearerAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete stream
* End point used internally to delete the stream
* @param streamId The identifier of the stream (required)
* @param forceDelete Whether or not to force delete the stream (optional)
* @throws ApiException if fails to make API call
*/
public void v2StreamsStreamIdDelete(String streamId, Boolean forceDelete) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'streamId' is set
if (streamId == null) {
throw new ApiException(400, "Missing the required parameter 'streamId' when calling v2StreamsStreamIdDelete");
}
// create path and map variables
String localVarPath = "/v2/streams/{streamId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "streamId" + "\\}", apiClient.escapeString(streamId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "forceDelete", forceDelete));
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "bearerAuth" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* A single stream
* Returns a single stream
* @param streamId The identifier of the stream (required)
* @return a {@code StreamOutputItem}
* @throws ApiException if fails to make API call
*/
public StreamOutputItem v2StreamsStreamIdGet(String streamId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'streamId' is set
if (streamId == null) {
throw new ApiException(400, "Missing the required parameter 'streamId' when calling v2StreamsStreamIdGet");
}
// create path and map variables
String localVarPath = "/v2/streams/{streamId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "streamId" + "\\}", apiClient.escapeString(streamId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "bearerAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Partially update a stream
* End point used internally to update the stream from poms (When creating a stream with ROLE_PROVIDER and type=\"channel\" only startTime and stopTime can be updated).
* @param streamId The identifier of the stream (required)
* @param streamPatchItem The stream object (required)
* @throws ApiException if fails to make API call
*/
public void v2StreamsStreamIdPatch(String streamId, StreamPatchItem streamPatchItem) throws ApiException {
Object localVarPostBody = streamPatchItem;
// verify the required parameter 'streamId' is set
if (streamId == null) {
throw new ApiException(400, "Missing the required parameter 'streamId' when calling v2StreamsStreamIdPatch");
}
// verify the required parameter 'streamPatchItem' is set
if (streamPatchItem == null) {
throw new ApiException(400, "Missing the required parameter 'streamPatchItem' when calling v2StreamsStreamIdPatch");
}
// create path and map variables
String localVarPath = "/v2/streams/{streamId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "streamId" + "\\}", apiClient.escapeString(streamId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "bearerAuth" };
apiClient.invokeAPI(localVarPath, "PATCH", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Update stream
* Replaces all existing stream properties
* @param streamId The identifier of the stream (required)
* @param streamPatchItem The stream object (required)
* @throws ApiException if fails to make API call
*/
public void v2StreamsStreamIdPut(String streamId, StreamPatchItem streamPatchItem) throws ApiException {
Object localVarPostBody = streamPatchItem;
// verify the required parameter 'streamId' is set
if (streamId == null) {
throw new ApiException(400, "Missing the required parameter 'streamId' when calling v2StreamsStreamIdPut");
}
// verify the required parameter 'streamPatchItem' is set
if (streamPatchItem == null) {
throw new ApiException(400, "Missing the required parameter 'streamPatchItem' when calling v2StreamsStreamIdPut");
}
// create path and map variables
String localVarPath = "/v2/streams/{streamId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "streamId" + "\\}", apiClient.escapeString(streamId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "bearerAuth" };
apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy