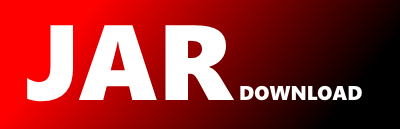
nl.vpro.nep.sam.model.Pagination Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of media-nep Show documentation
Show all versions of media-nep Show documentation
Support for the several APIs of NEP that POMS is integrating with
/*
* Stream Access Manager
* Get access to a stream in order to view it
*
* The version of the OpenAPI document: 4.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package nl.vpro.nep.sam.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import jakarta.validation.constraints.*;
import jakarta.validation.Valid;
/**
* Pagination
*/
@JsonPropertyOrder({
Pagination.JSON_PROPERTY_TOTAL,
Pagination.JSON_PROPERTY_LIMIT,
Pagination.JSON_PROPERTY_PAGE,
Pagination.JSON_PROPERTY_PAGE_COUNT
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-18T10:26:20.602909888Z[Etc/UTC]", comments = "Generator version: 7.6.0")
public class Pagination {
public static final String JSON_PROPERTY_TOTAL = "total";
private Integer total;
public static final String JSON_PROPERTY_LIMIT = "limit";
private Integer limit;
public static final String JSON_PROPERTY_PAGE = "page";
private Integer page;
public static final String JSON_PROPERTY_PAGE_COUNT = "pageCount";
private Integer pageCount;
public Pagination() {
}
public Pagination total(Integer total) {
this.total = total;
return this;
}
/**
* The total amount of items
* @return total
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TOTAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getTotal() {
return total;
}
@JsonProperty(JSON_PROPERTY_TOTAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotal(Integer total) {
this.total = total;
}
public Pagination limit(Integer limit) {
this.limit = limit;
return this;
}
/**
* The number of items per page
* @return limit
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getLimit() {
return limit;
}
@JsonProperty(JSON_PROPERTY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLimit(Integer limit) {
this.limit = limit;
}
public Pagination page(Integer page) {
this.page = page;
return this;
}
/**
* The current page number
* @return page
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPage() {
return page;
}
@JsonProperty(JSON_PROPERTY_PAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPage(Integer page) {
this.page = page;
}
public Pagination pageCount(Integer pageCount) {
this.pageCount = pageCount;
return this;
}
/**
* The total number of pages
* @return pageCount
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PAGE_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPageCount() {
return pageCount;
}
@JsonProperty(JSON_PROPERTY_PAGE_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPageCount(Integer pageCount) {
this.pageCount = pageCount;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Pagination pagination = (Pagination) o;
return Objects.equals(this.total, pagination.total) &&
Objects.equals(this.limit, pagination.limit) &&
Objects.equals(this.page, pagination.page) &&
Objects.equals(this.pageCount, pagination.pageCount);
}
@Override
public int hashCode() {
return Objects.hash(total, limit, page, pageCount);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Pagination {\n");
sb.append(" total: ").append(toIndentedString(total)).append("\n");
sb.append(" limit: ").append(toIndentedString(limit)).append("\n");
sb.append(" page: ").append(toIndentedString(page)).append("\n");
sb.append(" pageCount: ").append(toIndentedString(pageCount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy