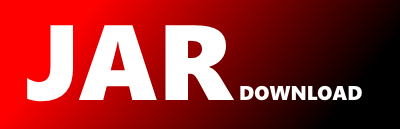
no.arktekk.siren.util.Lens Maven / Gradle / Ivy
package no.arktekk.siren.util;
import java.util.function.BiFunction;
import java.util.function.Function;
public final class Lens implements Function {
public final Function fget;
public final BiFunction fset;
private Lens(Function fget, BiFunction fset) {
this.fget = fget;
this.fset = fset;
}
public B get(A a) {
return fget.apply(a);
}
public A set(A a, B b) {
return fset.apply(a, b);
}
@Override
public B apply(A a) {
return get(a);
}
public A updated(A whole, B part) {
return set(whole, part);
}
public A mod(A a, Function f) {
return set(a, f.apply(apply(a)));
}
public Lens compose(final Lens that) {
return new Lens<>(c -> get(that.apply(c)), (c, b) -> that.mod(c, a -> set(a, b)));
}
public Lens andThen(Lens that) {
return that.compose(this);
}
public static Lens of(Function fget, BiFunction fset) {
return new Lens<>(fget, fset);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy