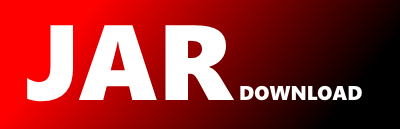
no.arkivverket.standarder.noark5.arkivmelding.Basisregistrering Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v2.3.7
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.10.09 at 07:52:57 AM UTC
//
package no.arkivverket.standarder.noark5.arkivmelding;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import no.arkivverket.standarder.noark5.metadatakatalog.Dokumentmedium;
/**
* Java class for basisregistrering complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="basisregistrering">
* <complexContent>
* <extension base="{http://www.arkivverket.no/standarder/noark5/arkivmelding}registrering">
* <sequence>
* <element name="tittel" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}tittel"/>
* <element name="offentligTittel" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}offentligTittel" minOccurs="0"/>
* <element name="beskrivelse" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}beskrivelse" minOccurs="0"/>
* <element name="noekkelord" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}noekkelord" maxOccurs="unbounded" minOccurs="0"/>
* <element name="forfatter" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}forfatter" maxOccurs="unbounded" minOccurs="0"/>
* <element name="dokumentmedium" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}dokumentmedium" minOccurs="0"/>
* <element name="oppbevaringssted" type="{http://www.arkivverket.no/standarder/noark5/metadatakatalog}oppbevaringssted" maxOccurs="unbounded" minOccurs="0"/>
* <element name="virksomhetsspesifikkeMetadata" type="{http://www.w3.org/2001/XMLSchema}anyType" minOccurs="0"/>
* <element name="merknad" type="{http://www.arkivverket.no/standarder/noark5/arkivmelding}merknad" maxOccurs="unbounded" minOccurs="0"/>
* <element name="kryssreferanse" type="{http://www.arkivverket.no/standarder/noark5/arkivmelding}kryssreferanse" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "basisregistrering", propOrder = {
"tittel",
"offentligTittel",
"beskrivelse",
"noekkelord",
"forfatter",
"dokumentmedium",
"oppbevaringssted",
"virksomhetsspesifikkeMetadata",
"merknad",
"kryssreferanse"
})
@XmlSeeAlso({
Journalpost.class
})
public class Basisregistrering
extends Registrering
{
@XmlElement(required = true)
protected String tittel;
protected String offentligTittel;
protected String beskrivelse;
protected List noekkelord;
protected List forfatter;
@XmlSchemaType(name = "string")
protected Dokumentmedium dokumentmedium;
protected List oppbevaringssted;
protected Object virksomhetsspesifikkeMetadata;
protected List merknad;
protected List kryssreferanse;
/**
* Gets the value of the tittel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTittel() {
return tittel;
}
/**
* Sets the value of the tittel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTittel(String value) {
this.tittel = value;
}
/**
* Gets the value of the offentligTittel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOffentligTittel() {
return offentligTittel;
}
/**
* Sets the value of the offentligTittel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOffentligTittel(String value) {
this.offentligTittel = value;
}
/**
* Gets the value of the beskrivelse property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBeskrivelse() {
return beskrivelse;
}
/**
* Sets the value of the beskrivelse property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBeskrivelse(String value) {
this.beskrivelse = value;
}
/**
* Gets the value of the noekkelord property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the noekkelord property.
*
*
* For example, to add a new item, do as follows:
*
* getNoekkelord().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getNoekkelord() {
if (noekkelord == null) {
noekkelord = new ArrayList();
}
return this.noekkelord;
}
/**
* Gets the value of the forfatter property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the forfatter property.
*
*
* For example, to add a new item, do as follows:
*
* getForfatter().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getForfatter() {
if (forfatter == null) {
forfatter = new ArrayList();
}
return this.forfatter;
}
/**
* Gets the value of the dokumentmedium property.
*
* @return
* possible object is
* {@link Dokumentmedium }
*
*/
public Dokumentmedium getDokumentmedium() {
return dokumentmedium;
}
/**
* Sets the value of the dokumentmedium property.
*
* @param value
* allowed object is
* {@link Dokumentmedium }
*
*/
public void setDokumentmedium(Dokumentmedium value) {
this.dokumentmedium = value;
}
/**
* Gets the value of the oppbevaringssted property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the oppbevaringssted property.
*
*
* For example, to add a new item, do as follows:
*
* getOppbevaringssted().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getOppbevaringssted() {
if (oppbevaringssted == null) {
oppbevaringssted = new ArrayList();
}
return this.oppbevaringssted;
}
/**
* Gets the value of the virksomhetsspesifikkeMetadata property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getVirksomhetsspesifikkeMetadata() {
return virksomhetsspesifikkeMetadata;
}
/**
* Sets the value of the virksomhetsspesifikkeMetadata property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setVirksomhetsspesifikkeMetadata(Object value) {
this.virksomhetsspesifikkeMetadata = value;
}
/**
* Gets the value of the merknad property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the merknad property.
*
*
* For example, to add a new item, do as follows:
*
* getMerknad().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Merknad }
*
*
*/
public List getMerknad() {
if (merknad == null) {
merknad = new ArrayList();
}
return this.merknad;
}
/**
* Gets the value of the kryssreferanse property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the kryssreferanse property.
*
*
* For example, to add a new item, do as follows:
*
* getKryssreferanse().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Kryssreferanse }
*
*
*/
public List getKryssreferanse() {
if (kryssreferanse == null) {
kryssreferanse = new ArrayList();
}
return this.kryssreferanse;
}
/**
* Copies all state of this object to a builder. This method is used by the {@link #copyOf} method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Basisregistrering.Builder<_B> _other) {
super.copyTo(_other);
_other.tittel = this.tittel;
_other.offentligTittel = this.offentligTittel;
_other.beskrivelse = this.beskrivelse;
if (this.noekkelord == null) {
_other.noekkelord = null;
} else {
_other.noekkelord = new ArrayList();
for (String _item: this.noekkelord) {
_other.noekkelord.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
if (this.forfatter == null) {
_other.forfatter = null;
} else {
_other.forfatter = new ArrayList();
for (String _item: this.forfatter) {
_other.forfatter.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.dokumentmedium = this.dokumentmedium;
if (this.oppbevaringssted == null) {
_other.oppbevaringssted = null;
} else {
_other.oppbevaringssted = new ArrayList();
for (String _item: this.oppbevaringssted) {
_other.oppbevaringssted.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.virksomhetsspesifikkeMetadata = this.virksomhetsspesifikkeMetadata;
if (this.merknad == null) {
_other.merknad = null;
} else {
_other.merknad = new ArrayList>>();
for (Merknad _item: this.merknad) {
_other.merknad.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.kryssreferanse == null) {
_other.kryssreferanse = null;
} else {
_other.kryssreferanse = new ArrayList>>();
for (Kryssreferanse _item: this.kryssreferanse) {
_other.kryssreferanse.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Override
public<_B >Basisregistrering.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Basisregistrering.Builder<_B>(_parentBuilder, this, true);
}
@Override
public Basisregistrering.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static Basisregistrering.Builder builder() {
return new Basisregistrering.Builder(null, null, false);
}
public static<_B >Basisregistrering.Builder<_B> copyOf(final Registrering _other) {
final Basisregistrering.Builder<_B> _newBuilder = new Basisregistrering.Builder<_B>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
public static<_B >Basisregistrering.Builder<_B> copyOf(final Basisregistrering _other) {
final Basisregistrering.Builder<_B> _newBuilder = new Basisregistrering.Builder<_B>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the {@link #copyOf} method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Basisregistrering.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree tittelPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("tittel"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(tittelPropertyTree!= null):((tittelPropertyTree == null)||(!tittelPropertyTree.isLeaf())))) {
_other.tittel = this.tittel;
}
final PropertyTree offentligTittelPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("offentligTittel"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(offentligTittelPropertyTree!= null):((offentligTittelPropertyTree == null)||(!offentligTittelPropertyTree.isLeaf())))) {
_other.offentligTittel = this.offentligTittel;
}
final PropertyTree beskrivelsePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("beskrivelse"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(beskrivelsePropertyTree!= null):((beskrivelsePropertyTree == null)||(!beskrivelsePropertyTree.isLeaf())))) {
_other.beskrivelse = this.beskrivelse;
}
final PropertyTree noekkelordPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("noekkelord"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(noekkelordPropertyTree!= null):((noekkelordPropertyTree == null)||(!noekkelordPropertyTree.isLeaf())))) {
if (this.noekkelord == null) {
_other.noekkelord = null;
} else {
_other.noekkelord = new ArrayList();
for (String _item: this.noekkelord) {
_other.noekkelord.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree forfatterPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("forfatter"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(forfatterPropertyTree!= null):((forfatterPropertyTree == null)||(!forfatterPropertyTree.isLeaf())))) {
if (this.forfatter == null) {
_other.forfatter = null;
} else {
_other.forfatter = new ArrayList();
for (String _item: this.forfatter) {
_other.forfatter.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree dokumentmediumPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dokumentmedium"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dokumentmediumPropertyTree!= null):((dokumentmediumPropertyTree == null)||(!dokumentmediumPropertyTree.isLeaf())))) {
_other.dokumentmedium = this.dokumentmedium;
}
final PropertyTree oppbevaringsstedPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("oppbevaringssted"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(oppbevaringsstedPropertyTree!= null):((oppbevaringsstedPropertyTree == null)||(!oppbevaringsstedPropertyTree.isLeaf())))) {
if (this.oppbevaringssted == null) {
_other.oppbevaringssted = null;
} else {
_other.oppbevaringssted = new ArrayList();
for (String _item: this.oppbevaringssted) {
_other.oppbevaringssted.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree virksomhetsspesifikkeMetadataPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("virksomhetsspesifikkeMetadata"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(virksomhetsspesifikkeMetadataPropertyTree!= null):((virksomhetsspesifikkeMetadataPropertyTree == null)||(!virksomhetsspesifikkeMetadataPropertyTree.isLeaf())))) {
_other.virksomhetsspesifikkeMetadata = this.virksomhetsspesifikkeMetadata;
}
final PropertyTree merknadPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("merknad"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(merknadPropertyTree!= null):((merknadPropertyTree == null)||(!merknadPropertyTree.isLeaf())))) {
if (this.merknad == null) {
_other.merknad = null;
} else {
_other.merknad = new ArrayList>>();
for (Merknad _item: this.merknad) {
_other.merknad.add(((_item == null)?null:_item.newCopyBuilder(_other, merknadPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree kryssreferansePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("kryssreferanse"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(kryssreferansePropertyTree!= null):((kryssreferansePropertyTree == null)||(!kryssreferansePropertyTree.isLeaf())))) {
if (this.kryssreferanse == null) {
_other.kryssreferanse = null;
} else {
_other.kryssreferanse = new ArrayList>>();
for (Kryssreferanse _item: this.kryssreferanse) {
_other.kryssreferanse.add(((_item == null)?null:_item.newCopyBuilder(_other, kryssreferansePropertyTree, _propertyTreeUse)));
}
}
}
}
@Override
public<_B >Basisregistrering.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Basisregistrering.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
public Basisregistrering.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >Basisregistrering.Builder<_B> copyOf(final Registrering _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Basisregistrering.Builder<_B> _newBuilder = new Basisregistrering.Builder<_B>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static<_B >Basisregistrering.Builder<_B> copyOf(final Basisregistrering _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Basisregistrering.Builder<_B> _newBuilder = new Basisregistrering.Builder<_B>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static Basisregistrering.Builder copyExcept(final Registrering _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Basisregistrering.Builder copyExcept(final Basisregistrering _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Basisregistrering.Builder copyOnly(final Registrering _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public static Basisregistrering.Builder copyOnly(final Basisregistrering _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public static class Builder<_B >
extends Registrering.Builder<_B>
implements Buildable
{
private String tittel;
private String offentligTittel;
private String beskrivelse;
private List noekkelord;
private List forfatter;
private Dokumentmedium dokumentmedium;
private List oppbevaringssted;
private Object virksomhetsspesifikkeMetadata;
private List>> merknad;
private List>> kryssreferanse;
public Builder(final _B _parentBuilder, final Basisregistrering _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.tittel = _other.tittel;
this.offentligTittel = _other.offentligTittel;
this.beskrivelse = _other.beskrivelse;
if (_other.noekkelord == null) {
this.noekkelord = null;
} else {
this.noekkelord = new ArrayList();
for (String _item: _other.noekkelord) {
this.noekkelord.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
if (_other.forfatter == null) {
this.forfatter = null;
} else {
this.forfatter = new ArrayList();
for (String _item: _other.forfatter) {
this.forfatter.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.dokumentmedium = _other.dokumentmedium;
if (_other.oppbevaringssted == null) {
this.oppbevaringssted = null;
} else {
this.oppbevaringssted = new ArrayList();
for (String _item: _other.oppbevaringssted) {
this.oppbevaringssted.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.virksomhetsspesifikkeMetadata = _other.virksomhetsspesifikkeMetadata;
if (_other.merknad == null) {
this.merknad = null;
} else {
this.merknad = new ArrayList>>();
for (Merknad _item: _other.merknad) {
this.merknad.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.kryssreferanse == null) {
this.kryssreferanse = null;
} else {
this.kryssreferanse = new ArrayList>>();
for (Kryssreferanse _item: _other.kryssreferanse) {
this.kryssreferanse.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
}
}
public Builder(final _B _parentBuilder, final Basisregistrering _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree tittelPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("tittel"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(tittelPropertyTree!= null):((tittelPropertyTree == null)||(!tittelPropertyTree.isLeaf())))) {
this.tittel = _other.tittel;
}
final PropertyTree offentligTittelPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("offentligTittel"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(offentligTittelPropertyTree!= null):((offentligTittelPropertyTree == null)||(!offentligTittelPropertyTree.isLeaf())))) {
this.offentligTittel = _other.offentligTittel;
}
final PropertyTree beskrivelsePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("beskrivelse"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(beskrivelsePropertyTree!= null):((beskrivelsePropertyTree == null)||(!beskrivelsePropertyTree.isLeaf())))) {
this.beskrivelse = _other.beskrivelse;
}
final PropertyTree noekkelordPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("noekkelord"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(noekkelordPropertyTree!= null):((noekkelordPropertyTree == null)||(!noekkelordPropertyTree.isLeaf())))) {
if (_other.noekkelord == null) {
this.noekkelord = null;
} else {
this.noekkelord = new ArrayList();
for (String _item: _other.noekkelord) {
this.noekkelord.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree forfatterPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("forfatter"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(forfatterPropertyTree!= null):((forfatterPropertyTree == null)||(!forfatterPropertyTree.isLeaf())))) {
if (_other.forfatter == null) {
this.forfatter = null;
} else {
this.forfatter = new ArrayList();
for (String _item: _other.forfatter) {
this.forfatter.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree dokumentmediumPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dokumentmedium"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dokumentmediumPropertyTree!= null):((dokumentmediumPropertyTree == null)||(!dokumentmediumPropertyTree.isLeaf())))) {
this.dokumentmedium = _other.dokumentmedium;
}
final PropertyTree oppbevaringsstedPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("oppbevaringssted"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(oppbevaringsstedPropertyTree!= null):((oppbevaringsstedPropertyTree == null)||(!oppbevaringsstedPropertyTree.isLeaf())))) {
if (_other.oppbevaringssted == null) {
this.oppbevaringssted = null;
} else {
this.oppbevaringssted = new ArrayList();
for (String _item: _other.oppbevaringssted) {
this.oppbevaringssted.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree virksomhetsspesifikkeMetadataPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("virksomhetsspesifikkeMetadata"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(virksomhetsspesifikkeMetadataPropertyTree!= null):((virksomhetsspesifikkeMetadataPropertyTree == null)||(!virksomhetsspesifikkeMetadataPropertyTree.isLeaf())))) {
this.virksomhetsspesifikkeMetadata = _other.virksomhetsspesifikkeMetadata;
}
final PropertyTree merknadPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("merknad"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(merknadPropertyTree!= null):((merknadPropertyTree == null)||(!merknadPropertyTree.isLeaf())))) {
if (_other.merknad == null) {
this.merknad = null;
} else {
this.merknad = new ArrayList>>();
for (Merknad _item: _other.merknad) {
this.merknad.add(((_item == null)?null:_item.newCopyBuilder(this, merknadPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree kryssreferansePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("kryssreferanse"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(kryssreferansePropertyTree!= null):((kryssreferansePropertyTree == null)||(!kryssreferansePropertyTree.isLeaf())))) {
if (_other.kryssreferanse == null) {
this.kryssreferanse = null;
} else {
this.kryssreferanse = new ArrayList>>();
for (Kryssreferanse _item: _other.kryssreferanse) {
this.kryssreferanse.add(((_item == null)?null:_item.newCopyBuilder(this, kryssreferansePropertyTree, _propertyTreeUse)));
}
}
}
}
}
protected<_P extends Basisregistrering >_P init(final _P _product) {
_product.tittel = this.tittel;
_product.offentligTittel = this.offentligTittel;
_product.beskrivelse = this.beskrivelse;
if (this.noekkelord!= null) {
final List noekkelord = new ArrayList(this.noekkelord.size());
for (Buildable _item: this.noekkelord) {
noekkelord.add(((String) _item.build()));
}
_product.noekkelord = noekkelord;
}
if (this.forfatter!= null) {
final List forfatter = new ArrayList(this.forfatter.size());
for (Buildable _item: this.forfatter) {
forfatter.add(((String) _item.build()));
}
_product.forfatter = forfatter;
}
_product.dokumentmedium = this.dokumentmedium;
if (this.oppbevaringssted!= null) {
final List oppbevaringssted = new ArrayList(this.oppbevaringssted.size());
for (Buildable _item: this.oppbevaringssted) {
oppbevaringssted.add(((String) _item.build()));
}
_product.oppbevaringssted = oppbevaringssted;
}
_product.virksomhetsspesifikkeMetadata = this.virksomhetsspesifikkeMetadata;
if (this.merknad!= null) {
final List merknad = new ArrayList(this.merknad.size());
for (Merknad.Builder> _item: this.merknad) {
merknad.add(_item.build());
}
_product.merknad = merknad;
}
if (this.kryssreferanse!= null) {
final List kryssreferanse = new ArrayList(this.kryssreferanse.size());
for (Kryssreferanse.Builder> _item: this.kryssreferanse) {
kryssreferanse.add(_item.build());
}
_product.kryssreferanse = kryssreferanse;
}
return super.init(_product);
}
/**
* Sets the new value of "tittel" (any previous value will be replaced)
*
* @param tittel
* New value of the "tittel" property.
*/
public Basisregistrering.Builder<_B> withTittel(final String tittel) {
this.tittel = tittel;
return this;
}
/**
* Sets the new value of "offentligTittel" (any previous value will be replaced)
*
* @param offentligTittel
* New value of the "offentligTittel" property.
*/
public Basisregistrering.Builder<_B> withOffentligTittel(final String offentligTittel) {
this.offentligTittel = offentligTittel;
return this;
}
/**
* Sets the new value of "beskrivelse" (any previous value will be replaced)
*
* @param beskrivelse
* New value of the "beskrivelse" property.
*/
public Basisregistrering.Builder<_B> withBeskrivelse(final String beskrivelse) {
this.beskrivelse = beskrivelse;
return this;
}
/**
* Adds the given items to the value of "noekkelord"
*
* @param noekkelord
* Items to add to the value of the "noekkelord" property
*/
public Basisregistrering.Builder<_B> addNoekkelord(final Iterable extends String> noekkelord) {
if (noekkelord!= null) {
if (this.noekkelord == null) {
this.noekkelord = new ArrayList();
}
for (String _item: noekkelord) {
this.noekkelord.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "noekkelord" (any previous value will be replaced)
*
* @param noekkelord
* New value of the "noekkelord" property.
*/
public Basisregistrering.Builder<_B> withNoekkelord(final Iterable extends String> noekkelord) {
if (this.noekkelord!= null) {
this.noekkelord.clear();
}
return addNoekkelord(noekkelord);
}
/**
* Adds the given items to the value of "noekkelord"
*
* @param noekkelord
* Items to add to the value of the "noekkelord" property
*/
public Basisregistrering.Builder<_B> addNoekkelord(String... noekkelord) {
addNoekkelord(Arrays.asList(noekkelord));
return this;
}
/**
* Sets the new value of "noekkelord" (any previous value will be replaced)
*
* @param noekkelord
* New value of the "noekkelord" property.
*/
public Basisregistrering.Builder<_B> withNoekkelord(String... noekkelord) {
withNoekkelord(Arrays.asList(noekkelord));
return this;
}
/**
* Adds the given items to the value of "forfatter"
*
* @param forfatter
* Items to add to the value of the "forfatter" property
*/
public Basisregistrering.Builder<_B> addForfatter(final Iterable extends String> forfatter) {
if (forfatter!= null) {
if (this.forfatter == null) {
this.forfatter = new ArrayList();
}
for (String _item: forfatter) {
this.forfatter.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "forfatter" (any previous value will be replaced)
*
* @param forfatter
* New value of the "forfatter" property.
*/
public Basisregistrering.Builder<_B> withForfatter(final Iterable extends String> forfatter) {
if (this.forfatter!= null) {
this.forfatter.clear();
}
return addForfatter(forfatter);
}
/**
* Adds the given items to the value of "forfatter"
*
* @param forfatter
* Items to add to the value of the "forfatter" property
*/
public Basisregistrering.Builder<_B> addForfatter(String... forfatter) {
addForfatter(Arrays.asList(forfatter));
return this;
}
/**
* Sets the new value of "forfatter" (any previous value will be replaced)
*
* @param forfatter
* New value of the "forfatter" property.
*/
public Basisregistrering.Builder<_B> withForfatter(String... forfatter) {
withForfatter(Arrays.asList(forfatter));
return this;
}
/**
* Sets the new value of "dokumentmedium" (any previous value will be replaced)
*
* @param dokumentmedium
* New value of the "dokumentmedium" property.
*/
public Basisregistrering.Builder<_B> withDokumentmedium(final Dokumentmedium dokumentmedium) {
this.dokumentmedium = dokumentmedium;
return this;
}
/**
* Adds the given items to the value of "oppbevaringssted"
*
* @param oppbevaringssted
* Items to add to the value of the "oppbevaringssted" property
*/
public Basisregistrering.Builder<_B> addOppbevaringssted(final Iterable extends String> oppbevaringssted) {
if (oppbevaringssted!= null) {
if (this.oppbevaringssted == null) {
this.oppbevaringssted = new ArrayList();
}
for (String _item: oppbevaringssted) {
this.oppbevaringssted.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "oppbevaringssted" (any previous value will be replaced)
*
* @param oppbevaringssted
* New value of the "oppbevaringssted" property.
*/
public Basisregistrering.Builder<_B> withOppbevaringssted(final Iterable extends String> oppbevaringssted) {
if (this.oppbevaringssted!= null) {
this.oppbevaringssted.clear();
}
return addOppbevaringssted(oppbevaringssted);
}
/**
* Adds the given items to the value of "oppbevaringssted"
*
* @param oppbevaringssted
* Items to add to the value of the "oppbevaringssted" property
*/
public Basisregistrering.Builder<_B> addOppbevaringssted(String... oppbevaringssted) {
addOppbevaringssted(Arrays.asList(oppbevaringssted));
return this;
}
/**
* Sets the new value of "oppbevaringssted" (any previous value will be replaced)
*
* @param oppbevaringssted
* New value of the "oppbevaringssted" property.
*/
public Basisregistrering.Builder<_B> withOppbevaringssted(String... oppbevaringssted) {
withOppbevaringssted(Arrays.asList(oppbevaringssted));
return this;
}
/**
* Sets the new value of "virksomhetsspesifikkeMetadata" (any previous value will be replaced)
*
* @param virksomhetsspesifikkeMetadata
* New value of the "virksomhetsspesifikkeMetadata" property.
*/
public Basisregistrering.Builder<_B> withVirksomhetsspesifikkeMetadata(final Object virksomhetsspesifikkeMetadata) {
this.virksomhetsspesifikkeMetadata = virksomhetsspesifikkeMetadata;
return this;
}
/**
* Adds the given items to the value of "merknad"
*
* @param merknad
* Items to add to the value of the "merknad" property
*/
public Basisregistrering.Builder<_B> addMerknad(final Iterable extends Merknad> merknad) {
if (merknad!= null) {
if (this.merknad == null) {
this.merknad = new ArrayList>>();
}
for (Merknad _item: merknad) {
this.merknad.add(new Merknad.Builder>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "merknad" (any previous value will be replaced)
*
* @param merknad
* New value of the "merknad" property.
*/
public Basisregistrering.Builder<_B> withMerknad(final Iterable extends Merknad> merknad) {
if (this.merknad!= null) {
this.merknad.clear();
}
return addMerknad(merknad);
}
/**
* Adds the given items to the value of "merknad"
*
* @param merknad
* Items to add to the value of the "merknad" property
*/
public Basisregistrering.Builder<_B> addMerknad(Merknad... merknad) {
addMerknad(Arrays.asList(merknad));
return this;
}
/**
* Sets the new value of "merknad" (any previous value will be replaced)
*
* @param merknad
* New value of the "merknad" property.
*/
public Basisregistrering.Builder<_B> withMerknad(Merknad... merknad) {
withMerknad(Arrays.asList(merknad));
return this;
}
/**
* Returns a new builder to build an additional value of the "Merknad" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Merknad.Builder#end()} to return to the current builder.
*
* @return
* a new builder to build an additional value of the "Merknad" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Merknad.Builder#end()} to return to the current builder.
*/
public Merknad.Builder extends Basisregistrering.Builder<_B>> addMerknad() {
if (this.merknad == null) {
this.merknad = new ArrayList>>();
}
final Merknad.Builder> merknad_Builder = new Merknad.Builder>(this, null, false);
this.merknad.add(merknad_Builder);
return merknad_Builder;
}
/**
* Adds the given items to the value of "kryssreferanse"
*
* @param kryssreferanse
* Items to add to the value of the "kryssreferanse" property
*/
public Basisregistrering.Builder<_B> addKryssreferanse(final Iterable extends Kryssreferanse> kryssreferanse) {
if (kryssreferanse!= null) {
if (this.kryssreferanse == null) {
this.kryssreferanse = new ArrayList>>();
}
for (Kryssreferanse _item: kryssreferanse) {
this.kryssreferanse.add(new Kryssreferanse.Builder>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "kryssreferanse" (any previous value will be replaced)
*
* @param kryssreferanse
* New value of the "kryssreferanse" property.
*/
public Basisregistrering.Builder<_B> withKryssreferanse(final Iterable extends Kryssreferanse> kryssreferanse) {
if (this.kryssreferanse!= null) {
this.kryssreferanse.clear();
}
return addKryssreferanse(kryssreferanse);
}
/**
* Adds the given items to the value of "kryssreferanse"
*
* @param kryssreferanse
* Items to add to the value of the "kryssreferanse" property
*/
public Basisregistrering.Builder<_B> addKryssreferanse(Kryssreferanse... kryssreferanse) {
addKryssreferanse(Arrays.asList(kryssreferanse));
return this;
}
/**
* Sets the new value of "kryssreferanse" (any previous value will be replaced)
*
* @param kryssreferanse
* New value of the "kryssreferanse" property.
*/
public Basisregistrering.Builder<_B> withKryssreferanse(Kryssreferanse... kryssreferanse) {
withKryssreferanse(Arrays.asList(kryssreferanse));
return this;
}
/**
* Returns a new builder to build an additional value of the "Kryssreferanse" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Kryssreferanse.Builder#end()} to return to the current builder.
*
* @return
* a new builder to build an additional value of the "Kryssreferanse" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Kryssreferanse.Builder#end()} to return to the current builder.
*/
public Kryssreferanse.Builder extends Basisregistrering.Builder<_B>> addKryssreferanse() {
if (this.kryssreferanse == null) {
this.kryssreferanse = new ArrayList>>();
}
final Kryssreferanse.Builder> kryssreferanse_Builder = new Kryssreferanse.Builder>(this, null, false);
this.kryssreferanse.add(kryssreferanse_Builder);
return kryssreferanse_Builder;
}
/**
* Sets the new value of "systemID" (any previous value will be replaced)
*
* @param systemID
* New value of the "systemID" property.
*/
@Override
public Basisregistrering.Builder<_B> withSystemID(final String systemID) {
super.withSystemID(systemID);
return this;
}
/**
* Sets the new value of "opprettetDato" (any previous value will be replaced)
*
* @param opprettetDato
* New value of the "opprettetDato" property.
*/
@Override
public Basisregistrering.Builder<_B> withOpprettetDato(final XMLGregorianCalendar opprettetDato) {
super.withOpprettetDato(opprettetDato);
return this;
}
/**
* Sets the new value of "opprettetAv" (any previous value will be replaced)
*
* @param opprettetAv
* New value of the "opprettetAv" property.
*/
@Override
public Basisregistrering.Builder<_B> withOpprettetAv(final String opprettetAv) {
super.withOpprettetAv(opprettetAv);
return this;
}
/**
* Sets the new value of "arkivertDato" (any previous value will be replaced)
*
* @param arkivertDato
* New value of the "arkivertDato" property.
*/
@Override
public Basisregistrering.Builder<_B> withArkivertDato(final XMLGregorianCalendar arkivertDato) {
super.withArkivertDato(arkivertDato);
return this;
}
/**
* Sets the new value of "arkivertAv" (any previous value will be replaced)
*
* @param arkivertAv
* New value of the "arkivertAv" property.
*/
@Override
public Basisregistrering.Builder<_B> withArkivertAv(final String arkivertAv) {
super.withArkivertAv(arkivertAv);
return this;
}
/**
* Sets the new value of "registreringsID" (any previous value will be replaced)
*
* @param registreringsID
* New value of the "registreringsID" property.
*/
@Override
public Basisregistrering.Builder<_B> withRegistreringsID(final String registreringsID) {
super.withRegistreringsID(registreringsID);
return this;
}
/**
* Sets the new value of "referanseForelderMappe" (any previous value will be replaced)
*
* @param referanseForelderMappe
* New value of the "referanseForelderMappe" property.
*/
@Override
public Basisregistrering.Builder<_B> withReferanseForelderMappe(final String referanseForelderMappe) {
super.withReferanseForelderMappe(referanseForelderMappe);
return this;
}
/**
* Sets the new value of "referanseArkivdel" (any previous value will be replaced)
*
* @param referanseArkivdel
* New value of the "referanseArkivdel" property.
*/
@Override
public Basisregistrering.Builder<_B> withReferanseArkivdel(final String referanseArkivdel) {
super.withReferanseArkivdel(referanseArkivdel);
return this;
}
/**
* Sets the new value of "referanseKlasse" (any previous value will be replaced)
*
* @param referanseKlasse
* New value of the "referanseKlasse" property.
*/
@Override
public Basisregistrering.Builder<_B> withReferanseKlasse(final String referanseKlasse) {
super.withReferanseKlasse(referanseKlasse);
return this;
}
/**
* Sets the new value of "skjerming" (any previous value will be replaced)
*
* @param skjerming
* New value of the "skjerming" property.
*/
@Override
public Basisregistrering.Builder<_B> withSkjerming(final Skjerming skjerming) {
super.withSkjerming(skjerming);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the "skjerming" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Skjerming.Builder#end()} to return to the current builder.
*
* @return
* A new builder to build the value of the "skjerming" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Skjerming.Builder#end()} to return to the current builder.
*/
public Skjerming.Builder extends Basisregistrering.Builder<_B>> withSkjerming() {
return ((Skjerming.Builder extends Basisregistrering.Builder<_B>> ) super.withSkjerming());
}
/**
* Sets the new value of "gradering" (any previous value will be replaced)
*
* @param gradering
* New value of the "gradering" property.
*/
@Override
public Basisregistrering.Builder<_B> withGradering(final Gradering gradering) {
super.withGradering(gradering);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the "gradering" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Gradering.Builder#end()} to return to the current builder.
*
* @return
* A new builder to build the value of the "gradering" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Gradering.Builder#end()} to return to the current builder.
*/
public Gradering.Builder extends Basisregistrering.Builder<_B>> withGradering() {
return ((Gradering.Builder extends Basisregistrering.Builder<_B>> ) super.withGradering());
}
/**
* Adds the given items to the value of "dokumentbeskrivelse_"
*
* @param dokumentbeskrivelse_
* Items to add to the value of the "dokumentbeskrivelse_" property
*/
@Override
public Basisregistrering.Builder<_B> addDokumentbeskrivelse(final Iterable extends Dokumentbeskrivelse> dokumentbeskrivelse_) {
super.addDokumentbeskrivelse(dokumentbeskrivelse_);
return this;
}
/**
* Adds the given items to the value of "dokumentbeskrivelse_"
*
* @param dokumentbeskrivelse_
* Items to add to the value of the "dokumentbeskrivelse_" property
*/
@Override
public Basisregistrering.Builder<_B> addDokumentbeskrivelse(Dokumentbeskrivelse... dokumentbeskrivelse_) {
super.addDokumentbeskrivelse(dokumentbeskrivelse_);
return this;
}
/**
* Returns a new builder to build an additional value of the "dokumentbeskrivelse" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Dokumentbeskrivelse.Builder#end()} to return to the current builder.
*
* @return
* a new builder to build an additional value of the "dokumentbeskrivelse" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Dokumentbeskrivelse.Builder#end()} to return to the current builder.
*/
@Override
public Dokumentbeskrivelse.Builder extends Basisregistrering.Builder<_B>> addDokumentbeskrivelse() {
return ((Dokumentbeskrivelse.Builder extends Basisregistrering.Builder<_B>> ) super.addDokumentbeskrivelse());
}
/**
* Adds the given items to the value of "dokumentobjekt_"
*
* @param dokumentobjekt_
* Items to add to the value of the "dokumentobjekt_" property
*/
@Override
public Basisregistrering.Builder<_B> addDokumentobjekt(final Iterable extends Dokumentobjekt> dokumentobjekt_) {
super.addDokumentobjekt(dokumentobjekt_);
return this;
}
/**
* Adds the given items to the value of "dokumentobjekt_"
*
* @param dokumentobjekt_
* Items to add to the value of the "dokumentobjekt_" property
*/
@Override
public Basisregistrering.Builder<_B> addDokumentobjekt(Dokumentobjekt... dokumentobjekt_) {
super.addDokumentobjekt(dokumentobjekt_);
return this;
}
/**
* Returns a new builder to build an additional value of the "dokumentobjekt" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Dokumentobjekt.Builder#end()} to return to the current builder.
*
* @return
* a new builder to build an additional value of the "dokumentobjekt" property.
* Use {@link no.arkivverket.standarder.noark5.arkivmelding.Dokumentobjekt.Builder#end()} to return to the current builder.
*/
@Override
public Dokumentobjekt.Builder extends Basisregistrering.Builder<_B>> addDokumentobjekt() {
return ((Dokumentobjekt.Builder extends Basisregistrering.Builder<_B>> ) super.addDokumentobjekt());
}
/**
* Adds the given items to the value of "dokumentbeskrivelseAndDokumentobjekt"
*
* @param dokumentbeskrivelseAndDokumentobjekt
* Items to add to the value of the "dokumentbeskrivelseAndDokumentobjekt" property
*/
@Override
public Basisregistrering.Builder<_B> addDokumentbeskrivelseAndDokumentobjekt(final Iterable> dokumentbeskrivelseAndDokumentobjekt) {
super.addDokumentbeskrivelseAndDokumentobjekt(dokumentbeskrivelseAndDokumentobjekt);
return this;
}
/**
* Adds the given items to the value of "dokumentbeskrivelseAndDokumentobjekt"
*
* @param dokumentbeskrivelseAndDokumentobjekt
* Items to add to the value of the "dokumentbeskrivelseAndDokumentobjekt" property
*/
@Override
public Basisregistrering.Builder<_B> addDokumentbeskrivelseAndDokumentobjekt(Object... dokumentbeskrivelseAndDokumentobjekt) {
super.addDokumentbeskrivelseAndDokumentobjekt(dokumentbeskrivelseAndDokumentobjekt);
return this;
}
/**
* Sets the new value of "dokumentbeskrivelseAndDokumentobjekt" (any previous value will be replaced)
*
* @param dokumentbeskrivelseAndDokumentobjekt
* New value of the "dokumentbeskrivelseAndDokumentobjekt" property.
*/
@Override
public Basisregistrering.Builder<_B> withDokumentbeskrivelseAndDokumentobjekt(final Iterable> dokumentbeskrivelseAndDokumentobjekt) {
super.withDokumentbeskrivelseAndDokumentobjekt(dokumentbeskrivelseAndDokumentobjekt);
return this;
}
/**
* Sets the new value of "dokumentbeskrivelseAndDokumentobjekt" (any previous value will be replaced)
*
* @param dokumentbeskrivelseAndDokumentobjekt
* New value of the "dokumentbeskrivelseAndDokumentobjekt" property.
*/
@Override
public Basisregistrering.Builder<_B> withDokumentbeskrivelseAndDokumentobjekt(Object... dokumentbeskrivelseAndDokumentobjekt) {
super.withDokumentbeskrivelseAndDokumentobjekt(dokumentbeskrivelseAndDokumentobjekt);
return this;
}
@Override
public Basisregistrering build() {
if (_storedValue == null) {
return this.init(new Basisregistrering());
} else {
return ((Basisregistrering) _storedValue);
}
}
public Basisregistrering.Builder<_B> copyOf(final Basisregistrering _other) {
_other.copyTo(this);
return this;
}
public Basisregistrering.Builder<_B> copyOf(final Basisregistrering.Builder _other) {
return copyOf(_other.build());
}
}
public static class Select
extends Basisregistrering.Selector
{
Select() {
super(null, null, null);
}
public static Basisregistrering.Select _root() {
return new Basisregistrering.Select();
}
}
public static class Selector , TParent >
extends Registrering.Selector
{
private com.kscs.util.jaxb.Selector> tittel = null;
private com.kscs.util.jaxb.Selector> offentligTittel = null;
private com.kscs.util.jaxb.Selector> beskrivelse = null;
private com.kscs.util.jaxb.Selector> noekkelord = null;
private com.kscs.util.jaxb.Selector> forfatter = null;
private com.kscs.util.jaxb.Selector> dokumentmedium = null;
private com.kscs.util.jaxb.Selector> oppbevaringssted = null;
private com.kscs.util.jaxb.Selector> virksomhetsspesifikkeMetadata = null;
private Merknad.Selector> merknad = null;
private Kryssreferanse.Selector> kryssreferanse = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap();
products.putAll(super.buildChildren());
if (this.tittel!= null) {
products.put("tittel", this.tittel.init());
}
if (this.offentligTittel!= null) {
products.put("offentligTittel", this.offentligTittel.init());
}
if (this.beskrivelse!= null) {
products.put("beskrivelse", this.beskrivelse.init());
}
if (this.noekkelord!= null) {
products.put("noekkelord", this.noekkelord.init());
}
if (this.forfatter!= null) {
products.put("forfatter", this.forfatter.init());
}
if (this.dokumentmedium!= null) {
products.put("dokumentmedium", this.dokumentmedium.init());
}
if (this.oppbevaringssted!= null) {
products.put("oppbevaringssted", this.oppbevaringssted.init());
}
if (this.virksomhetsspesifikkeMetadata!= null) {
products.put("virksomhetsspesifikkeMetadata", this.virksomhetsspesifikkeMetadata.init());
}
if (this.merknad!= null) {
products.put("merknad", this.merknad.init());
}
if (this.kryssreferanse!= null) {
products.put("kryssreferanse", this.kryssreferanse.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> tittel() {
return ((this.tittel == null)?this.tittel = new com.kscs.util.jaxb.Selector>(this._root, this, "tittel"):this.tittel);
}
public com.kscs.util.jaxb.Selector> offentligTittel() {
return ((this.offentligTittel == null)?this.offentligTittel = new com.kscs.util.jaxb.Selector>(this._root, this, "offentligTittel"):this.offentligTittel);
}
public com.kscs.util.jaxb.Selector> beskrivelse() {
return ((this.beskrivelse == null)?this.beskrivelse = new com.kscs.util.jaxb.Selector>(this._root, this, "beskrivelse"):this.beskrivelse);
}
public com.kscs.util.jaxb.Selector> noekkelord() {
return ((this.noekkelord == null)?this.noekkelord = new com.kscs.util.jaxb.Selector>(this._root, this, "noekkelord"):this.noekkelord);
}
public com.kscs.util.jaxb.Selector> forfatter() {
return ((this.forfatter == null)?this.forfatter = new com.kscs.util.jaxb.Selector>(this._root, this, "forfatter"):this.forfatter);
}
public com.kscs.util.jaxb.Selector> dokumentmedium() {
return ((this.dokumentmedium == null)?this.dokumentmedium = new com.kscs.util.jaxb.Selector>(this._root, this, "dokumentmedium"):this.dokumentmedium);
}
public com.kscs.util.jaxb.Selector> oppbevaringssted() {
return ((this.oppbevaringssted == null)?this.oppbevaringssted = new com.kscs.util.jaxb.Selector>(this._root, this, "oppbevaringssted"):this.oppbevaringssted);
}
public com.kscs.util.jaxb.Selector> virksomhetsspesifikkeMetadata() {
return ((this.virksomhetsspesifikkeMetadata == null)?this.virksomhetsspesifikkeMetadata = new com.kscs.util.jaxb.Selector>(this._root, this, "virksomhetsspesifikkeMetadata"):this.virksomhetsspesifikkeMetadata);
}
public Merknad.Selector> merknad() {
return ((this.merknad == null)?this.merknad = new Merknad.Selector>(this._root, this, "merknad"):this.merknad);
}
public Kryssreferanse.Selector> kryssreferanse() {
return ((this.kryssreferanse == null)?this.kryssreferanse = new Kryssreferanse.Selector>(this._root, this, "kryssreferanse"):this.kryssreferanse);
}
}
}