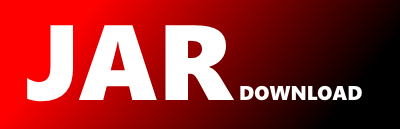
no.difi.vefa.peppol.evidence.jaxb.saml.ObjectFactory Maven / Gradle / Ivy
Show all versions of peppol-evidence Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.7
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.02.24 at 09:35:26 PM UTC
//
package no.difi.vefa.peppol.evidence.jaxb.saml;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the no.difi.vefa.peppol.evidence.jaxb.saml package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AuthnContextDeclRef_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthnContextDeclRef");
private final static QName _Attribute_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Attribute");
private final static QName _AuthnContext_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthnContext");
private final static QName _EncryptedAssertion_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "EncryptedAssertion");
private final static QName _Statement_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Statement");
private final static QName _AuthzDecisionStatement_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthzDecisionStatement");
private final static QName _EncryptedAttribute_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "EncryptedAttribute");
private final static QName _AudienceRestriction_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AudienceRestriction");
private final static QName _AuthenticatingAuthority_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthenticatingAuthority");
private final static QName _AuthnStatement_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthnStatement");
private final static QName _AssertionIDRef_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AssertionIDRef");
private final static QName _Audience_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Audience");
private final static QName _Assertion_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Assertion");
private final static QName _AuthnContextDecl_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthnContextDecl");
private final static QName _Evidence_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Evidence");
private final static QName _Issuer_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Issuer");
private final static QName _Action_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Action");
private final static QName _EncryptedID_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "EncryptedID");
private final static QName _BaseID_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "BaseID");
private final static QName _Condition_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Condition");
private final static QName _Subject_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Subject");
private final static QName _SubjectConfirmation_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "SubjectConfirmation");
private final static QName _OneTimeUse_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "OneTimeUse");
private final static QName _AttributeValue_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AttributeValue");
private final static QName _Conditions_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Conditions");
private final static QName _SubjectLocality_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "SubjectLocality");
private final static QName _SubjectConfirmationData_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "SubjectConfirmationData");
private final static QName _AttributeStatement_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AttributeStatement");
private final static QName _AssertionURIRef_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AssertionURIRef");
private final static QName _Advice_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "Advice");
private final static QName _NameID_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "NameID");
private final static QName _ProxyRestriction_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "ProxyRestriction");
private final static QName _AuthnContextClassRef_QNAME = new QName("urn:oasis:names:tc:SAML:2.0:assertion", "AuthnContextClassRef");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: no.difi.vefa.peppol.evidence.jaxb.saml
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ActionType }
*
*/
public ActionType createActionType() {
return new ActionType();
}
/**
* Create an instance of {@link NameIDType }
*
*/
public NameIDType createNameIDType() {
return new NameIDType();
}
/**
* Create an instance of {@link AttributeType }
*
*/
public AttributeType createAttributeType() {
return new AttributeType();
}
/**
* Create an instance of {@link SubjectConfirmationDataType }
*
*/
public SubjectConfirmationDataType createSubjectConfirmationDataType() {
return new SubjectConfirmationDataType();
}
/**
* Create an instance of {@link EncryptedElementType }
*
*/
public EncryptedElementType createEncryptedElementType() {
return new EncryptedElementType();
}
/**
* Create an instance of {@link ConditionsType }
*
*/
public ConditionsType createConditionsType() {
return new ConditionsType();
}
/**
* Create an instance of {@link SubjectLocalityType }
*
*/
public SubjectLocalityType createSubjectLocalityType() {
return new SubjectLocalityType();
}
/**
* Create an instance of {@link AuthnContextType }
*
*/
public AuthnContextType createAuthnContextType() {
return new AuthnContextType();
}
/**
* Create an instance of {@link AudienceRestrictionType }
*
*/
public AudienceRestrictionType createAudienceRestrictionType() {
return new AudienceRestrictionType();
}
/**
* Create an instance of {@link SubjectConfirmationType }
*
*/
public SubjectConfirmationType createSubjectConfirmationType() {
return new SubjectConfirmationType();
}
/**
* Create an instance of {@link EvidenceType }
*
*/
public EvidenceType createEvidenceType() {
return new EvidenceType();
}
/**
* Create an instance of {@link OneTimeUseType }
*
*/
public OneTimeUseType createOneTimeUseType() {
return new OneTimeUseType();
}
/**
* Create an instance of {@link AuthzDecisionStatementType }
*
*/
public AuthzDecisionStatementType createAuthzDecisionStatementType() {
return new AuthzDecisionStatementType();
}
/**
* Create an instance of {@link AssertionType }
*
*/
public AssertionType createAssertionType() {
return new AssertionType();
}
/**
* Create an instance of {@link ProxyRestrictionType }
*
*/
public ProxyRestrictionType createProxyRestrictionType() {
return new ProxyRestrictionType();
}
/**
* Create an instance of {@link SubjectType }
*
*/
public SubjectType createSubjectType() {
return new SubjectType();
}
/**
* Create an instance of {@link AttributeStatementType }
*
*/
public AttributeStatementType createAttributeStatementType() {
return new AttributeStatementType();
}
/**
* Create an instance of {@link AuthnStatementType }
*
*/
public AuthnStatementType createAuthnStatementType() {
return new AuthnStatementType();
}
/**
* Create an instance of {@link AdviceType }
*
*/
public AdviceType createAdviceType() {
return new AdviceType();
}
/**
* Create an instance of {@link KeyInfoConfirmationDataType }
*
*/
public KeyInfoConfirmationDataType createKeyInfoConfirmationDataType() {
return new KeyInfoConfirmationDataType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AuthnContextDeclRef")
public JAXBElement createAuthnContextDeclRef(String value) {
return new JAXBElement(_AuthnContextDeclRef_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AttributeType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "Attribute")
public JAXBElement createAttribute(AttributeType value) {
return new JAXBElement(_Attribute_QNAME, AttributeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AuthnContextType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AuthnContext")
public JAXBElement createAuthnContext(AuthnContextType value) {
return new JAXBElement(_AuthnContext_QNAME, AuthnContextType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EncryptedElementType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "EncryptedAssertion")
public JAXBElement createEncryptedAssertion(EncryptedElementType value) {
return new JAXBElement(_EncryptedAssertion_QNAME, EncryptedElementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StatementAbstractType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "Statement")
public JAXBElement createStatement(StatementAbstractType value) {
return new JAXBElement(_Statement_QNAME, StatementAbstractType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AuthzDecisionStatementType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AuthzDecisionStatement")
public JAXBElement createAuthzDecisionStatement(AuthzDecisionStatementType value) {
return new JAXBElement(_AuthzDecisionStatement_QNAME, AuthzDecisionStatementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EncryptedElementType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "EncryptedAttribute")
public JAXBElement createEncryptedAttribute(EncryptedElementType value) {
return new JAXBElement(_EncryptedAttribute_QNAME, EncryptedElementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AudienceRestrictionType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AudienceRestriction")
public JAXBElement createAudienceRestriction(AudienceRestrictionType value) {
return new JAXBElement(_AudienceRestriction_QNAME, AudienceRestrictionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AuthenticatingAuthority")
public JAXBElement createAuthenticatingAuthority(String value) {
return new JAXBElement(_AuthenticatingAuthority_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AuthnStatementType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AuthnStatement")
public JAXBElement createAuthnStatement(AuthnStatementType value) {
return new JAXBElement(_AuthnStatement_QNAME, AuthnStatementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AssertionIDRef")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
public JAXBElement createAssertionIDRef(String value) {
return new JAXBElement(_AssertionIDRef_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "Audience")
public JAXBElement createAudience(String value) {
return new JAXBElement(_Audience_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AssertionType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "Assertion")
public JAXBElement createAssertion(AssertionType value) {
return new JAXBElement(_Assertion_QNAME, AssertionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:2.0:assertion", name = "AuthnContextDecl")
public JAXBElement