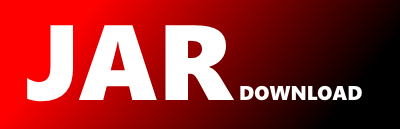
commonMain.Outcome.kt Maven / Gradle / Ivy
The newest version!
package no.dossier.libraries.functional
import kotlin.js.JsExport
@JsExport
sealed class Outcome
class Success(val value: T) : Outcome()
class Failure(val error: E) : Outcome()
@JsExport
inline fun Outcome.map(transform: (T) -> U): Outcome =
when (this) {
is Success -> Success(transform(value))
is Failure -> this
}
@JsExport
inline fun Outcome.mapError(transform: (E) -> F): Outcome =
when (this) {
is Success -> this
is Failure -> Failure(transform(error))
}
@JsExport
inline fun Outcome.andThen(transform: (T) -> Outcome): Outcome =
when (this) {
is Success -> transform(value)
is Failure -> this
}
@JsExport
inline fun Outcome.failUnless(
conditionBuilder: (T) -> Boolean,
errorBuilder: (T) -> E
): Outcome =
when (this) {
is Success -> if (conditionBuilder(value)) this else Failure(errorBuilder(value))
is Failure -> this
}
@JsExport
inline fun Outcome.getOrElse(
onFailure: (Failure) -> Nothing
): T =
when(this) {
is Failure -> onFailure(this)
is Success -> value
}
@Deprecated("Method renamed to unwrap", replaceWith = ReplaceWith("unwrap"))
fun Outcome.forceGet(): T = unwrap()
fun Outcome.unwrap(): T =
getOrElse { throw RuntimeException(it.error.toString()) }
@JsExport
inline fun Outcome.resolve(
onFailure: (Failure) -> T
): T =
when(this) {
is Failure -> onFailure(this)
is Success -> value
}
@JsExport
fun Iterable>.partition(): Pair>, List>> =
Pair(this.filterIsInstance>(), this.filterIsInstance>())
@JsExport
fun Iterable.traverseToOutcome(f: (T) -> Outcome): Outcome> {
tailrec fun go(iter: Iterator, values: MutableList): Outcome> =
if (iter.hasNext()) {
when (val elemResult = f(iter.next())) {
is Success -> {
values.add(elemResult.value)
go(iter, values)
}
is Failure -> elemResult
}
} else {
Success(values)
}
return go(iterator(), mutableListOf())
}
@JsExport
fun Iterable>.sequenceToOutcome(): Outcome> = traverseToOutcome { it }
@JsExport
inline fun runCatching(errorBuilder: (exception: Exception) -> E, block: () -> T): Outcome = try {
Success(block())
}
catch(e: Exception) {
Failure(errorBuilder(e))
}
@JsExport
fun T?.notNull(errorBuilder: () -> E) = this?.let { Success(it) } ?: Failure(errorBuilder())
© 2015 - 2024 Weber Informatics LLC | Privacy Policy