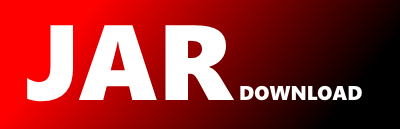
no.finn.lambdacompanion.Failure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda-companion Show documentation
Show all versions of lambda-companion Show documentation
Functional structures and utilities for Java 8 and lambdas
package no.finn.lambdacompanion;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
public class Failure extends Try {
private Throwable e;
public Failure(Throwable e) {
this.e = e;
}
public Throwable getThrowable() {
return e;
}
@SuppressWarnings("unchecked")
@Override
public Try map(ThrowingFunction super T, ? extends U, ? extends Throwable> mapper) {
return (Try) this;
}
@SuppressWarnings("unchecked")
@Override
public Try flatMap(ThrowingFunction super T, ? extends Try, ? extends Throwable> mapper) {
return (Try) this;
}
@Override
public Optional> filter(final Predicate predicate) {
return Optional.of(this);
}
@Override
public void forEach(ThrowingConsumer super T, ? extends Throwable> consumer) {}
@Override
public Try peek(ThrowingConsumer super T, ? extends Throwable> consumer) {
forEach(consumer);
return this;
}
@Override
public Try peekFailure(Consumer> consumer) {
consumer.accept(this);
return this;
}
@Override
public T orElse(T defaultValue) {
return defaultValue;
}
@Override
public T orElseGet(Supplier extends T> defaultValue) {
return defaultValue.get();
}
@Override
public Optional toOptional() {
return Optional.empty();
}
@Override
public U recover(Function super T, ? extends U> successFunc,
Function failureFunc) {
return failureFunc.apply(e);
}
@Override
public Either extends Throwable, T> toEither() {
return Either.left(e);
}
@SuppressWarnings("unchecked")
@Override
public T orElseThrow(Function throwableMapper) throws Y {
throw throwableMapper.apply((X) e);
}
@Override
public T orElseRethrow() throws E {
throw (E) e;
}
@Override
public String toString() {
return "Failure{" +
"e=" + e +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Failure> failure = (Failure>) o;
return !(e != null ? !e.equals(failure.e) : failure.e != null);
}
@Override
public int hashCode() {
return e != null ? e.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy