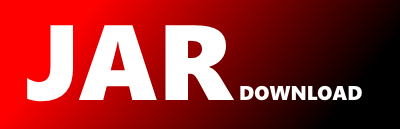
no.finn.lambdacompanion.Functions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda-companion Show documentation
Show all versions of lambda-companion Show documentation
Functional structures and utilities for Java 8 and lambdas
package no.finn.lambdacompanion;
import java.util.List;
import java.util.function.BiFunction;
public final class Functions {
private Functions() {
}
/**
* @param a
* @param b
* @param accumulator accumulator
* @param b b
* @param list list
* @return b
* Head recursive fold. Fold the list by combining the first element with the results of combining the rest
*
* Example:
*
* {@code
* : f
* / \ foldRight f z -> / \
* 1 : 1 f
* / \ / \
* 2 [] 2 z
* }
*
*/
public static B foldRight(final BiFunction accumulator, final B b, final List list) {
return list.isEmpty() ? b : accumulator.apply(head(list), foldRight(accumulator, b, tail(list)));
}
/**
* @param a
* @param b
* @param accumulator accumulator
* @param b b
* @param list list
* @return b
* Tail recursive fold. Fold the list by recursively combining the results of combining all but the last element with the last one
*
* Example:
*
* {@code
* : f
* / \ foldLeft f z -> / \
* 1 : f 2
* / \ / \
* 2 [] z 1
* }
*
*/
public static B foldLeft(final BiFunction accumulator, final B b, final List list) {
return list.isEmpty() ? b : foldLeft(accumulator, accumulator.apply(head(list), b), tail(list));
}
/**
* @param a
* @param list list
* @return first element of the given list.
* @throws IndexOutOfBoundsException if the list is empty
*/
public static A head(final List list) {
return list.get(0);
}
/**
* @param a
* @param list list
* @return all but the first elements of the given list
* @throws IndexOutOfBoundsException if the list is empty
*/
public static List tail(final List list) {
return list.subList(1, list.size());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy