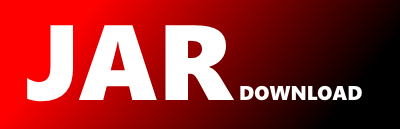
no.kodeworks.kvarg.util.package.scala Maven / Gradle / Ivy
package no.kodeworks.kvarg
import akka.actor.Cancellable
import shapeless.{HList, LowPriority, Typeable, Witness, tag}
import shapeless.tag.{@@, Tagged}
import cats.syntax.option._
import scala.concurrent.{ExecutionContext, Future, Promise}
import scala.util.Try
package object util {
object emptyCancellable extends Cancellable {
override def cancel() = true
override def isCancelled = true
}
implicit class RichFuture[T](f: Future[T]) {
def mapAll[Target](m: Try[T] => Target)(implicit ec: ExecutionContext): Future[Target] = {
val promise = Promise[Target]()
f.onComplete { r => promise success m(r) }(ec)
promise.future
}
def flatMapAll[Target](m: Try[T] => Future[Target])(implicit ec: ExecutionContext): Future[Target] = {
val promise = Promise[Target]()
f.onComplete { r => m(r).onComplete { z => promise complete z }(ec) }(ec)
promise.future
}
}
def seqFutures[T, U]
(ts: TraversableOnce[T])
(ff: T => Future[U])
(implicit ec: ExecutionContext): Future[List[U]] = {
ts.foldLeft(Future.successful[List[U]](Nil)) {
(f, item) =>
f.flatMap {
x => ff(item).map(_ :: x)
}
} map (_.reverse)
}
def lowerFirstChar(s: String): String =
(s.head + 32).toChar.toString + s.substring(1)
def typeableToSimpleName(tt: Typeable[_], firstCharAsLowerCase: Boolean = true): String = {
val s = tt.describe
val s1 = (s.lastIndexOf('.') match {
case -1 => s
case i => s.substring(i + 1)
})
val s2 = s1.indexOf('[') match {
case -1 => s1
case i => s.substring(0, i)
}
if (firstCharAsLowerCase) lowerFirstChar(s2)
else s2
}
def hlistToList[T](hlist: shapeless.HList): List[T] = {
import shapeless._
hlist match {
case HNil => Nil
case head :: tail =>
collection.immutable.::(head.asInstanceOf[T], hlistToList[T](tail))
}
}
def listToHList[H <: HList](list: List[_]): H = {
import shapeless._
(list match {
case Nil => HNil
case collection.immutable.::(head, tail) =>
(head :: listToHList[H](tail))
}).asInstanceOf[H]
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy